From this tutorial, you will learn about the Python list Insert method. You will see how to use it on sequences with the help of examples.
Note: The syntax used in the below section is for Python 3. You can change it to any other version of Python.
Python List Insert
To Learn about Lists – Read Python List
List Insert Method
The Insert function is a built-in list method that allows you to insert items or objects at any position in a sequence. It’s a list-only method.
Its syntax is as follows:
List_name.insert(index, element)
The arguments are “index” and “element” where the index is the position of the item that needs to be inserted, and the “element” is the element given by the user.
The insert method returns nothing, i.e., it has no return value. See the below example.
>>> myList = [1, 9, 16, 25] >>> myList.insert(1, 4) >>> print(myList) [1, 4, 9, 16, 25] >>>
In the above example code, you can observe that we have a list containing the sequence of squares of natural numbers from 1 to 5. Here the “4” was missing. To remedy this, we inserted it at 1st index in the list.
How does the Insert() function work?
When the user calls the insert method and passes the index, the function first checks its existence in the list.
If it exists, then the element gets inserted at the given position in the list. After that, it also updates the indexes of the rest of the items.
The following flowchart simplifies it for you:
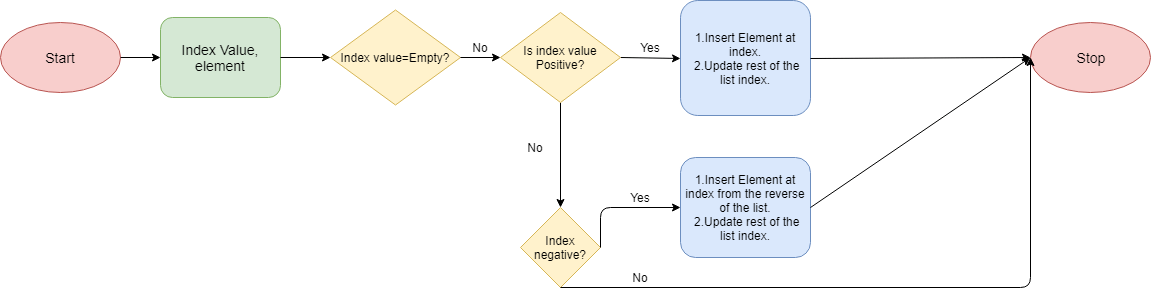
Program Examples
We can insert different data types in a list. Examples include a list, tuple, strings, etc.
Insert a string in the list
List = [1,2,3] List.insert(0, "Insert") print(List)
The output is as follows:
['Insert', 1, 2, 3]
Insert a tuple in the list
List = [1, 2, 3] List.insert(1, (6, 5)) print(List)
The result is as follows:
[1, (6, 5), 2, 3]
List after using the wrong index
List = [1,2,3] List.insert(5,"Insert") print (List) List.insert(-4, "Beginning") print (List)
The output is as follows:
[1, 2, 3, 'Insert'] ['Beginning', 1, 2, 3, 'Insert']
Even when using out-of-range index values, the insert method will still insert at the ends of the list.
Key Facts
Here is a table summarizing some important facts about the list.insert() method in Python:
Fact | Description |
---|---|
The list insert() method inserts an element into a list at a specified index. | The first argument is the index of the element before which to insert, so Python list.insert(0, x) inserts at the front of the list, and list.insert(len(list), x) is equivalent to list.append(x). |
The insert() method does not return any value. | It simply inserts the element into the list. |
The insert() method can be used to insert any type of object into a list. | This includes strings, numbers, lists, dictionaries, and tuples. |
The insert() method can be used to insert multiple elements into a list at once. | To do this, you can pass a list of elements as the second argument to the insert() method. |
Also Read: 40 Python Exercises for Beginners
When should you use list.insert() in Python?
Here are some unique examples or problems where the list.insert() method can be useful:
- Inserting an element into a list at a specific index: This is the most common use case for the list.insert() method. For example, you could use it to insert a new name into a list of students or to insert a new date into a list of birthdays.
- Inserting multiple elements into a list at once: You can use the list.insert() method to insert multiple elements into a list at once. For example, you could use it to insert a list of new students into a list of all students or to insert a list of new dates into a list of all birthdays.
- Inserting an element into a list in a sorted order: You can use the list.insert() method to insert an element into a list in a sorted order. For example, you could use it to insert a new student into a list of students sorted by name or to insert a new date into a list of birthdays sorted by date.
- Inserting an element into a list at the beginning or end: You can use the list.insert() method to insert an element into a list at the beginning or end. For example, you could use it to insert a new student at the beginning of a list of students or to insert a new date at the end of a list of birthdays.
- Inserting an element into a list in place of another element: You can use the list.insert() method to insert an element into a list in place of another element. For example, you could use it to replace a student’s name in a list of students or to replace a date in a list of birthdays.
We hope this explanation is helpful. If you have any other questions, please let us know.
Best,
TechBeamers