From this tutorial, you will be learning about the Python list Sort method. You will see how to use it with lists with the help of examples.
Note: The syntax used in the below section is for Python 3. You can change it to any other version of Python.
Python List Sort() Explained
In order to learn more about List features and methods – Read from our in-depth guide on Python List.
List Sort Method
The sort method performs the sorting of list elements in either ascending or descending directions. Its syntax is as follows:
# Syntax - How to use sort method. List_name.sort(key = …, reverse = ...)
When the sort() gets called without arguments, it sorts in ascending order by default. It doesn’t have a return value.
It merely returns to the next line without returning any output.
Please note that it is not related to the built-in sorted() function. The sort method mutates or modifies an old list whereas sorted() creates a new sorted sequence.
How does the Sort method work?
When we call the Python sort method, it traverses the list of elements in a loop and rearranges them in ascending order when there are no arguments.
If we specify “reverse = true” as an argument, then the list gets sorted in descending order.
The primary parameter is the steps that the method must go through when sorting through a list of elements. The value given to the key can be a function or a simple calculation etc.
The flowchart for the mechanism is as follows:
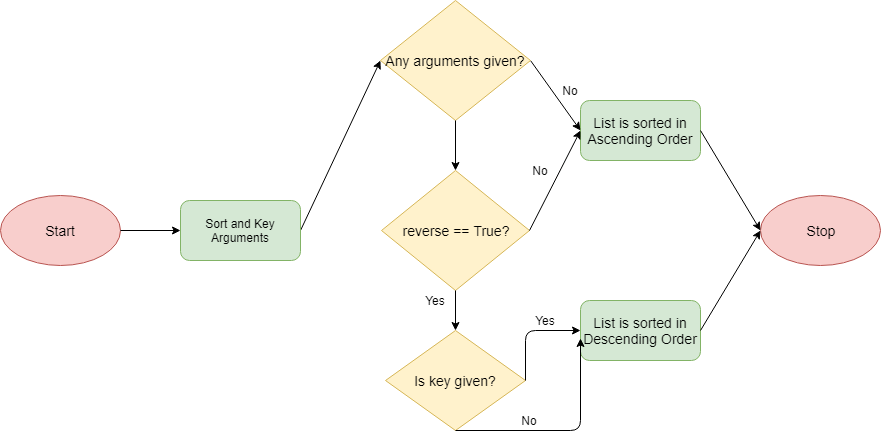
Sort Method Examples
In order to understand how can we sort a list in Python, let’s check out different examples. We need to go through a variety of use cases to understand the sorting concept.
Sort a list of numbers
a. Sorting a list of numbers in ascending order
Natural_numbers = [1,4,23,3,2,1,0,9,7] Natural_numbers.sort() print (Natural_numbers)
Output:
[0, 1, 1, 2, 3, 4, 7, 9, 23]
b. Sorting a list of numbers in descending order
Natural_numbers = [1,23,4,25,22,3,4,5,9,7,5] Natural_numbers.sort(reverse = True) print (Natural_numbers)
Output:
[25, 23, 22, 9, 7, 5, 5, 4, 4, 3, 1]
While here you get to see a variety of usage of the sort method, understanding the Python zip function would still be worth reading. It is a powerful tool that has many applications apart from sorting multiple lists in parallel.
Sort a list of letters
a. Sorting a list of letters in Python (ascending order)
Vowels = ["a", "u", "i", "o", "e"] Vowels.sort() print (Vowels)
Output:
['a', 'e', 'i', 'o', 'u']
b. Sorting a list of letters in Python (descending order)
Vowels = ["a", "u", "i", "o", "e"] Vowels.sort(reverse = True) print (Vowels)
Output:
['u', 'o', 'i', 'e', 'a']
Sort a list of strings in Python
a. Sorting a list of strings in ascending order
Fruits = ["Apple", "Banana", "Tomato", "Grapes"] Fruits.sort() print (Fruits)
Output:
['Apple', 'Banana', 'Grapes', 'Tomato']
b. Sorting a Python list in descending order
Fruits = ["Apple", "Banana", "Tomato", "Grapes"] Fruits.sort(reverse = True) print (Fruits)
Output:
['Tomato', 'Grapes', 'Banana', 'Apple']
Sort list by a key function
a. Sort a list by a key function (ascending)
# Let's sort on the basis of 2nd element def keyFunc(item): return item[1] # Unordered list unordered = [('b', 'b'), ('c', 'd'), ('d', 'a'), ('a', 'c')] # Order list using key unordered.sort(key=keyFunc) # Output the sorted list print('Ordered list:', unordered)
Output:
Ordered list: [('d', 'a'), ('b', 'b'), ('a', 'c'), ('c', 'd')]
b. Sorting a list using a key function (descending)
# Let's sort on the basis of 2nd element def keyFunc(item): return item[1] # Unordered list unordered = [('b', 'b'), ('c', 'd'), ('d', 'a'), ('a', 'c')] # Order list using key in the reverse direction unordered.sort(key=keyFunc, reverse = True) # Output the sorted list print('Ordered list:', unordered)
Output:
Ordered list: [('c', 'd'), ('a', 'c'), ('b', 'b'), ('d', 'a')]
Must Read: Sorting a Dictionary in Python
Best,
TechBeamers