From this tutorial, you will learn about the Python list copy method. You will see how to use it with lists with the help of examples.
Note: The syntax used here is for Python 3. You may modify it to use with other versions of Python.
Python List Copy
To Learn Python from Scratch – Read Python Tutorial
List Copy Method
The Copy method performs the shallow copy of a list. The syntax used is:
List_name.copy()
It doesn’t accept any argument and also doesn’t return a value. It produces a shallow copy and exits after it.
Please don’t get confused with the List Copy method with the Python Copy module. The latter provides developers with the ability to create both Shallow copy and deep copy.
The List copy only provides the ability to create a shallow copy. Next, you will see the difference between a Shallow copy and a Deep copy.
Difference: Shallow Copy Vs. Deep Copy
A shallow copy is one in which a new object gets created which stores the reference of another object.
While the deep copy produces a new object that stores all references of another object making it another list separate from the original one.
Thus when you make a change to the deep copy of a list, the old list doesn’t get affected. But the same does get changed during the shallow copying.
List Copy Mechanism
When we call the copy method in a Python program, it takes the old list, creates a new object, and stores in it all the references to the old one.
The following flowchart attempts to simplify it for you:
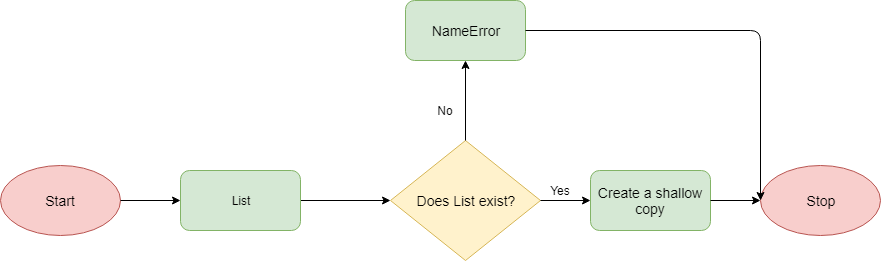
Examples
1. Creating a copy of List containing elements
Natural_Numbers = [1,2,3,4,5,6,7,8,9] New_Copy = Natural_Numbers.copy() print (New_Copy)
#1 Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
2. Creating a copy of List containing strings
Strings = ["Linux", "Windows", "MacOS", "Chrome OS"] New_Strings = Strings.copy() print (New_Strings)
#2 Output:
['Linux', 'Windows', 'MacOS', 'Chrome OS']
3. Creating a copy of an empty list
List = [] New_List = List.copy() print (New_List)
#3 Output:
[]
4. Creating a copy of a non-existent List
New_List = List.copy()
#4 Output:
Traceback (most recent call last): File "C:\Python\Python35\listcopy.py", line 1, in <module> New_List = List.copy() NameError: name 'List' is not defined
Also Read: How to Find the Index of an Item in a List?
Key Facts
Here are important facts about the Python list copy() method. The table below is nicely summing up all of them:
Aspect | Description |
---|---|
Name | copy() method |
Purpose | To create a shallow copy of an existing list, producing a new list with the same elements. |
Input | Takes no arguments; it operates on the list from which it’s called. |
Output | Returns a new list with the same elements as the original list. |
Shallow Copy | Produces a shallow copy, meaning it duplicates the list structure but not the nested objects. |
Modifies Original | Keeps the original list as is; any changes to the new list do not affect the original. |
Nested Objects | If the list contains nested objects (e.g., lists or dictionaries), the copy still references the same nested objects. |
Common Use Cases | Used when you need to duplicate a list to work with independently while preserving the original. |
Memory Efficiency | Creates a new list, consuming additional memory; useful when you want separate copies. |
Nesting Implications | Be aware that changes to nested objects are reflected in both the original and copied lists if they reference the same objects. |
Safety for Modifications | Suitable for scenarios where you want to avoid unintended modifications to the original list. |
Similarity to List Slicing | Similar to list slicing [:] , but the copy() method is more explicit for creating copies. |
The copy() method is a straightforward way to duplicate a list in Python, allowing you to work with the copy independently while preserving the original list.
Best,
TechBeamers