From this tutorial, you will be learning about Python Iterator. It is a type of container holding references to other elements. It provides the next() method to access each item. Today, you’ll see how it works and also get to use built-in iterators like lists, tuples, etc. with examples.
Moreover, Python permits us to create user-defined iterators. We can do so by defining it using a Python class. The class then has to implement the required iterator properties and methods. We’ve got it covered in this tutorial and also provided code for practice.
Note: The syntax used here is for Python 3. You may modify it to use with other versions of Python.
What is Python Iterator?
An iterator is a collection object that holds multiple values and provides a mechanism to traverse through them. Examples of inbuilt iterators in Python are lists, dictionaries, tuples, etc.
It works according to the iterator protocol. The protocol requires to implement two methods. They are __iter__ and __next__.
The __iter__() function returns an iterable object, whereas the __next__() gives a reference of the following items in the collection.
How to use iterators in Python?
Most of the time, you have to use an import statement for calling functions of a module in Python. However, iterators don’t need one as you can use them implicitly.
When you create an object, you can make it iterable by calling the __iter__() method over it. After that, you can iterate its values with the help of __next__(). When there is nothing left to traverse, then you get the StopIteration exception. It indicates that you’ve reached the end of the iterable object.
The for loop automatically creates an iterator while traversing through an object’s element.
The following flowchart attempts to simplify the concept for you.
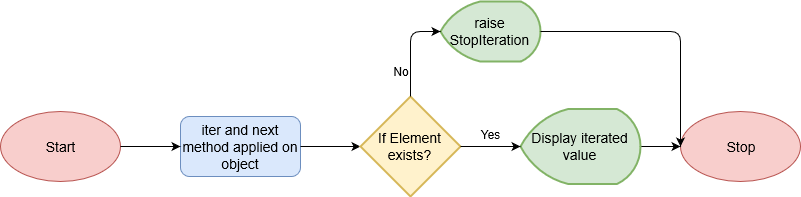
Iterator Syntax
To use iterators, you can use the methods as defined above __iter__ and __next__ methods.
You can create an iterable object as per the below instruction:
iterable_object = iter(my_object_to_iterate_through)
Once, you get a hold of the iterator, then use the following statement to cycle through it.
iterable_object = iter(my_object_to_iterate_through) next(iterable_object)
By the way, the Python zip function can be quite useful in your regular programming tasks. It helps you iterate, combine, compare, and search over multiple lists.
Iterator Examples
For illustration, here are some Python code examples where you can learn how to use the iterator.
Creating an iterable from Tuple
Cubes = (1, 8, 27, 64, 125, 216) cube = iter(Cubes) print(next(cube)) print(next(cube))
Output
1 8
Creating an iterable from List
Negative_numbers = [-1, -8, -27, -64, -125, -216] Negative_number = iter(Negative_numbers) print(next(Negative_number)) print(next(Negative_number))
Output
-1 -8
Iterating through an empty object
List = [] empty_element = iter(List) print(next(empty_element)) print(next(empty_element))
Output
Traceback (most recent call last): File "C:\Users\porting-dev\AppData\Local\Programs\Python\Python35\test11.py", line 3, in <module> next(empty_element) StopIteration
Iterating a non-existent object
List = [1,2,3,4] empty = iter(List) print(next(empty)) print(next(empty)) # Output # 1 2
Printing a list of natural numbers
The below example provides a script that can get called or executed in the interpreter shell.
Please be careful about the indentation blocks when you enter the code in the interpreter shell.
class natural_numbers: def __init__(self, max = 0): self.max = max def __iter__(self): self.number = 1 return self def __next__(self): if self.max == self.number: raise StopIteration else: number = self.number self.number += 1 return number numbers = natural_numbers(10) i = iter(numbers) print("# Calling next() one by one:") print(next(i)) print(next(i)) print("\n") # Call next method in a loop print("# Calling next() in a loop:") for i in numbers: print(i)
To execute the above program, use the command python3 /path_to_filename depending upon the default Python version used. The following is the output of the above Python iterator program.
# Calling next() one by one: 1 2 # Calling next() in a loop: 1 2 3 4 5 6 7 8 9
Summary – Iterator in Python
We hope that after wrapping up this tutorial, you must be feeling comfortable using the Python iterator. However, you may practice more with examples to gain confidence.
Next, we recommend you read about generators in Python. They are also used to create iterators but in a much easier fashion. You don’t need to write __iter__() and __next__() functions. Instead, you write a generator function that uses the yield statement for returning a value.
The yield’s call saves the state of the function and resumes from the same point if called again. It helps the code to generate a set of values over time, rather than getting them all at once. You can get the complete details from the below tutorial.