Let’s learn how to write a factorial program in Python with just a few lines of code. The factorial of a number is the product of all positive integers from 1 to that number.
In Python, we can write a simple and efficient algorithm to calculate factorials. Let’s explore how it can be done.
3 Ways to Find Factorial in Python
In this tutorial, we are covering the following methods.
- The first method uses a for loop to write the logic.
- In the second one, you will see recursion in action.
- Thereafter, the example will utilize a built-in function.
And lastly, you’ll find a program with added error handling. A basic flowchart is also available at the end of the tutorial.
1. Factorial Program in Python Using For Loop
The program calculates factorial by multiplying numbers from 1 to the given number. It uses Python for loop to make the logic easier to understand.
Example
# Take user input to calculate factorial num = int(input("Enter a number: ")) factorial = 1 # Check if the input is negative, positive or zero if num < 0: print("Factorial of negative value is not defined") elif num == 0: print("Factorial of 0 is ‘1’") else: for i in range(1, num+1): factorial *= i print("The factorial of", num, "is", factorial)
Summary
It was a simple factorial program in Python using the for loop.
1) The code first verifies whether the user input is positive, negative, or zero.
2) If it is positive the loop starts from 1 and goes up to num+1. The range() function excludes the upper limit. The program multiples the factorial variable by each number in the loop.
In short, the code prompts the user to enter a number. After that, it calculates the factorial using a loop and then displays the result to the user.
2. Factorial Program in Python Using Recursion
This program calculates factorial using recursion, calling itself to multiply numbers. This approach simplifies the process in Python, making it easier to understand and implement.
Example
# Recursive factorial function def factorial(n): if n == 0: return 1 else: # Recursive call to the factorial function return n * factorial(n-1) # Asking the user to supply input num = int(input("Enter a value: ")) # Call the Factorial function result = factorial(num) print("The factorial of", num, "is", result)
Summary
The above program demonstrates factorial calculation in Python using recursion.
1) The code first defines a factorial function that uses recursion. It first prompts the user to enter a value.
2) The program then calls the factorial function with the given input.
3) The factorial function calls itself recursively, each time decreasing the value of ‘num’ by 1.
4) By the time, the value of ‘num’ becomes 0, the factorial of the number is calculated and saved in the result.
3. Factorial Using Built-in Python Function
This program calls a built-in Python function, i.e., factorial(). It is a much simpler approach than the previous two.
However, it requires the use of an external module.
Example
import math n = int(input ("Input the number: ")) result = math.factorial(n) print("The factorial of", n, "is", result)
Summary
The above Python factorial program follows below simple steps.
1) In the first line, we import the math module. It allows us to do various mathematical operations.
2) We then define the variable “n” for which we want to calculate the factorial.
3) Next, we call the math.factorial() function with num as the argument. And then stores the output in the variable result.
4) Finally, we will print the result of the Python example to the console.
Please note that the factorial() function works only for non-negative integers. It will raise a ValueError exception for any negative value or a non-integer input.
4. Factorial Program with Error Handling
Here is one more example of factorial in Python. It also does error handling.
def factorial(n): if n < 0: raise ValueError("factorial is not defined for negative values") elif n == 0: return 1 else: return n * factorial(n-1) # Example usage try: fact = int(input("Enter a number: ")) print("The factorial of", fact, "is", factorial(fact)) except ValueError as e: print("Error:", e)
5. Flowchart
As we are now at the end of this tutorial, review this basic flowchart model. It covers the steps of a general factorial program in Python.
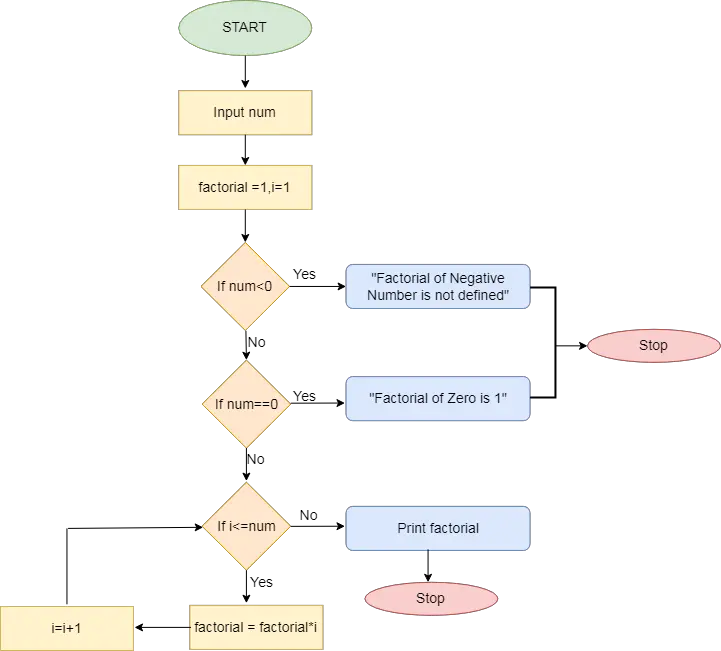
Quick Wrap Up
To sum up, the factorial program in Python is a helpful exercise for beginner programmers to hone their programming skills. It even makes them recall basic math concepts and think about their logic.
By learning and using this program, you can improve your problem-solving skills and explore more possibilities in Python programming.
Happy Coding!