From this tutorial, you will learn and be able to use the Python file I/O methods to read and write in a text file (.txt) with the help of an extensive set of code examples.
Understand Python File Read/Write Operations
For writing to a txt file in Python, you would need a couple of functions such as Open(), Write(), and Read(). All these are built-in Python functions and don’t require a module to import.
You may have to interact with two major types of files while programming. One is the text file that contains streams of ASCII or UNICODE (UTF-8) characters. Each line ends with a new line (“\n”) char, a.k.a. EOL (End of Line).
Another type of file is called binary which contains machine-readable data. It doesn’t have, a so-called line as there is no line-ending. Only the application using it would know about its content.
This tutorial will strictly tell you to work with the text files only. Let’s begin this tutorial by taking on the first call required to write to a file in Python, i.e., Open().
Open File in Python
You first have to open a file in Python for writing. Python provides the built-in open() function.
The open() function would return a handle to the file if opened successfully. It takes two arguments, as shown below:
''' Python open file syntax ''' file_handle = open("file_name", "access_mode")
The first argument is the name or path of the file (including the file name). For example – sample_log.txt or /Users/john/home/sample_log.txt.
The second parameter (optional) represents a mode to open the file. The value of the “access_mode” defines the operation you want to perform on it. The default value is the READ-only mode.
# Open a file named "sample_log.txt" # It rests in the same directory as you are working in. file_handle1 = open("sample_log.txt") # Let's open the file from a given path file_handle2 = open("/Users/john/home/sample_log.txt")
Also Check: Loop Through Files in a Directory using Python
File Open Modes
It is optional to pass the mode argument. If you don’t set it, then Python uses “r” as the default value for the access mode. It means that Python will open a file for read-only purposes.
However, there are a total of six access modes available in Python.
- “r” – It opens a text file for reading. It keeps the offset at the start of the file. If the file is missing, then it raises an I/O error. It is also the default mode.
- “r+” – It opens the file for both READ and WRITE operations. It sets the offset at the start of the file. An I/O error occurs for a non-existent file.
- “w” – It opens a file for writing and overwrites any existing content. The handle remains at the start of the data. If the file doesn’t exist, then it creates one.
- “w+” – It opens the file for both READ and WRITE operations. Rest, it works the same as the “w” mode.
- “a” – It opens a file for writing or creates a new one if the file is not found. The handle moves to the end (EOF). It preserves the existing content and inserts data to the end.
- “a+” – It opens the file for both READ and WRITE operations. Rest, it works the same as the “a” mode.
Check out a few examples:
# Open a file named "sample_log.txt" in write mode ### # It rests in the same directory as you are working in. file_handle1 = open("sample_log.txt", "w") # Open the file from a given path in append mode file_handle2 = open("/Users/john/home/sample_log.txt", "a")
Write File in Python
Python provides two functions to write to a text file having a txt extension: Write()
and Writelines()
.
1. write()
– Let’s first use write() for writing to a file in Python. This function puts the given text in a single line.
''' Python write() function ''' file_handle.write("some text")
But, first, open any IDE and create a file named “sample_log.txt
” for our test. Don’t make any other changes to it.
Please note – If you try to open a file for reading and it doesn’t exist, then Python will throw the FileNotFoundError exception.
To edit this file from your Python program, we’ve given the following code:
# A simple example - Python write file ### file_handle = open("sample_log.txt","w") file_handle.write("Hello Everyone!") file_handle.write("It is my first attempt to write to a file in Python.") file_handle.write("I'll first open the file and then write.") file_handle.write("Finally, I'll close it.") file_handle.close()
We’ve opened the file in “w” mode, which means to overwrite anything written previously. So, after you open it and see its content, you’ll find the new text in four different lines.
2. writelines()
– The writelines()
is another Python function that you can try for writing to a Txt file. takes a list of strings as the input and inserts each of them as a separate line in one go. You can check its syntax below:
''' Python writelines() function ''' file_handle.writelines([str1, str2, str3, ...])
Append File in Python
The append is also a file operation for writing the text to the end of a file.
You also need to know how to append the new text to an existing file. There are two modes available for this purpose: a and a+.
Whenever you open a file using one of these modes, the file offset is set to the EOF. So, you can write the new content or text next to the existing content.
Let’s understand it with a few lines of code:
We’ll first open a file in “a” mode. If you run this example the first time, then it creates the file.
# Python Append File in "a" mode Example ### fh = open("test_append_a.txt", "a") fh.write("Insert First Line\n") fh.write("Append Next Line\n")
So far, two lines have been added to the file. The second Python statement writes the strings to the end of the text file.
Now, you’ll see the difference between the “a” and “a+” modes. Let’s try a read operation and see what happens.
fh.read() # io.UnsupportedOperation: not readable
The above line of code would fail as the “a” mode doesn’t allow READ. So, close it, open it, and then do a read operation.
fh.close() # Close the file fh = open("test_append_a.txt") # Open in the default read mode print(fh.read()) # Now, read and print the entire file fh.close()
The output is something like:
Insert First Line Append Next Line
Let’s now try appending using the “a+” mode. Check out the below code:
# Python Append File in "a+" mode Example ### fh = open("test_append_aplus.txt", "a+") fh.write("Insert First Line\n") fh.write("Append Next Line\n") fh.seek(0) # Set offset position to the start print(fh.read()) # READ is sucess in a+ mode ## Output # Insert First Line # Append Next Line fh.write("Append Another Line\n") # WRITE another line to the text file fh.seek(0) # Set the offset for reading print(fh.read()) # Do the READ operation again ## Output # Insert First Line # Append Next Line # Append Another Line
Read File in Python
For reading a text file, Python bundles the following three functions: read()
, readline()
, and readlines()
1. read()
– It reads the given number of bytes (N) as a string. If no value is given, then it reads the file till the EOF.
''' Python read() function ''' #Syntax file_handle.read([N])
2. readline()
– It reads the specified number of bytes (N) as a string from a single line in the file. It restricts to one line per call even if N is more than the bytes available in one line.
''' Python readline() function ''' #Syntax file_handle.readline([N])
3. readlines()
– It reads every line present in the text file and returns them as a list of strings.
''' Python readlines() function ''' #Syntax file_handle.readlines()
It is so easy to use the Python read file functions that you can check yourself. Just type the following code in your IDE or the default Python IDE, i.e., IDLE.
# Python Read File Example ### fh = open("sample_log.txt") # No need to specify the mode as READ is the default mode print(fh.read()) # Expect the whole file to get printed here fh.seek(0) # Reset the file offset to the beginning of the file print(fh.readline()) # Print just the first line from the file fh.seek(0) # Reset the offset again print(fh.readlines()) # Print the list of lines fh.close() # Close the file handle
Python seek() function sets a location in the file and allows programs to read or write text from there. As a result of these operations, the file pointer moves along.
Close File in Python
File handling in Python starts with opening a file and ends with closing it. It means that you must close a file after you are finished doing the file operations.
Closing a file is a cleanup activity, which means to free up the system resources. It is also essential because you can only open a limited number of file handles.
Also, please note that any attempt to access the file after closing would throw an I/O error. You may have already seen us using it in our previous examples in this post.
With Statement in Python
If you want a cleaner and elegant way to write to a file in Python, then try using the WITH statement. It does the automatic cleanup of the system resources like file handles.
Also, it provides out-of-the-box exception handling (Python try-except) while you are working with the files. Check out the following example to see how with statement works.
# Write File in Python using WITH statement ## # Sample code(1) Write to a text file fh = open("sample_log.txt", "w") try: fh.write("I love Python Programming!") finally: fh.close() # Sample code(2) Write using with statement with open("sample_log.txt", "w") as fh: fh.write("I love Python even more!!")
Working sample code
Below is a full-fledged example that demonstrates the usage of the following functions:
- Write to a text file using Python
write()
&writelines()
- Read from a txt file using Python
read()
,readline()
, andreadlines()
# Python Write File/ Read File Example ### print("### Python Write File/ Read File Example ###\n") file_handle = open("sample_log.txt", "w") list_of_strings = ["Python programming \n","Web development \n","Agile Scrum \n"] # Write a newline char at each line in the file file_handle.write("Welcome! \n") file_handle.writelines(list_of_strings) file_handle.close() # Open the text file for reading file_handle = open("sample_log.txt", "r+") # Read the entire text file and display its content print("1) Demonstrate Python read() function") out = file_handle.read() print("\n>>>Python read() file output:\n{}".format(out)) # Now, set the file offset to the beginning file_handle.seek(False) # Read the first line from the text file using readline() print("2) Demonstrate Python readline() function") out = file_handle.readline() print("\n>>>Python readline() file output:\n\tLine#1{}".format(out)) # Again, position the file offset to zero file_handle.seek(False) # Read the entire text file using readlines() print("3) Demonstrate Python readlines() function") out = file_handle.readlines() file_handle.close() print("\n>>>Python readlines() file output:") for i, line in enumerate(out): print("\tLine#{} {}".format(i+1, line))
This Python program generates the following output:
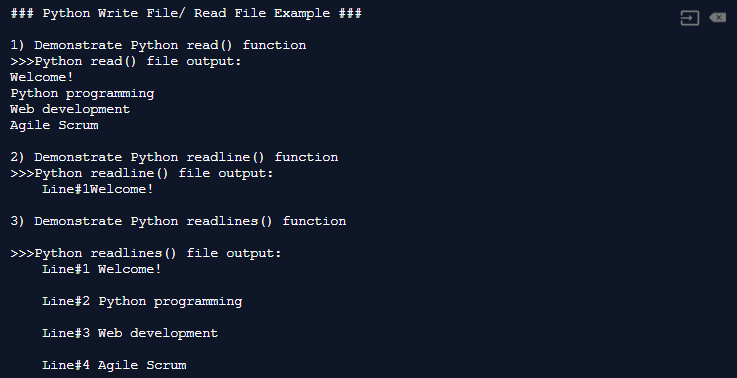
Attempt the Quiz
We’ve now come to the closure of this Read/Write File in Python tutorial. If you have read it from start to end, then file handling would be on your tips. However, we recommend the following quizzes to attempt.
These are quick questionnaires to test how much knowledge you have retained after reading the above.
Python File Handling Quiz
Take this quiz to test your knowledge of Python file-handling functions and access modes.
Also, you must use these concepts in your projects or write some hands-on code to solve real-time problems. It’ll certainly help you grasp faster and remember better.
Quick Reference
By the way, read our step-by-step tutorials from the following link, if you wish to learn Python from scratch to depth.