Greetings readers, in today’s tutorial, we’ll explain the essential elements of Python socket programming. Python’s socket interface is similar to C and Java. So if you already have a C/Java programming background, then it is much easier for you to learn socket programming in Python.
Using sockets in Python is a lot simpler than C which fosters rapid application development. So, don’t worry if Python is your first programming language instead feel blessed.
Python offers two types of API libraries that we can use for socket programming. At the low level, Python utilizes the socket library to implement client and server modules for both connectionless and connection-oriented network protocols. Whereas, at the higher level, You can use libraries such as the ftplib
and httplib
to interact with application-level network protocols like FTP and HTTP.
In this post, we will be discussing the most widely used socket library specially designed for Python Socket Programming. We will go through the primary functions available with this library that can help you build the client and server modules. Finally, you’ll see a demonstration of client-server communication via a sample code.
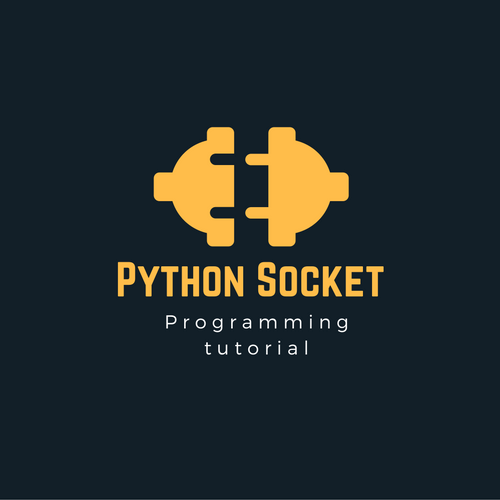
To help you get started quickly, we have organized the socket concepts in the table of contents below. Just click on the topics that interest you to learn more.
A socket is the most vital and fundamental entity that you need to know for learning Python socket programming. In this section, we’ve covered the socket concept and the related methods to create and communicate with the sockets.
What are Sockets?
Sockets are the end-point of a two-way communication link. An endpoint is a combination of the IP address and the port number.
For Client-Server communication, sockets are to be configured at the two ends to initiate a connection, listen for incoming messages, and then send the responses at both ends thereby establishing a bidirectional communication.
Sockets allow communication between processes that lie on the same machine, or different machines working in diverse environments and even across different continents.
How do You Create a Socket Object in Python?
To create/initialize a socket, we use the socket.socket() method. It has the definition in Python’s socket module. To start with the socket programming in Python, first, you use the following syntax to create a socket.
sock_obj = socket.socket( socket_family, socket_type, protocol=0)
Where,
- socket_family: Defines the family of protocols used as the transport mechanism. It can have either of the two values.
- Either AF_UNIX, or
- AF_INET (IP version 4 or IPv4).
- socket_type: Defines the types of communication between the two end-points. It can have the following values.
- SOCK_STREAM (for connection-oriented protocols, e.g., TCP), or
- SOCK_DGRAM (for connectionless protocols e.g. UDP).
- protocol: We typically leave this field or set this field to zero.
import socket #for sockets #Instantiate an AF_INET, STREAM socket (TCP) sock_obj = socket.socket(socket.AF_INET, socket.SOCK_STREAM) print ('Socket Initialized')
So this is how you can create a socket object. But what if the above examples fail to instantiate the socket? How would you troubleshoot the error in your Python socket programming script?
You need to wrap the above code in Python’s try-except block. With Python exception handling, you can trace the cause of the error.
#Managing errors in python socket programming import socket #for sockets import sys #for exit try: #create an AF_INET, STREAM socket (TCP) sock_obj = socket.socket(socket.AF_INET, socket.SOCK_STREAM) except socket.error as err_msg: print ('Unable to instantiate socket. Error code: ' + str(err_msg[0]) + ' , Error message : ' + err_msg[1]) sys.exit(); print ('Socket Initialized')
In the subsequent section, we will explain the functions available in the Socket library to create a client/server program.
Types of Socket Methods Available in Python
We can classify the socket methods in the following three categories used for Python socket programming.
- Server Socket Methods,
- Client Socket Methods, and
- General Socket Methods.
What Server Socket Methods are Available in Python?
Server Socket Methods
- sock_object.bind(address):
- This method binds the socket to the address (hostname, port number pair)
- sock_object.listen(backlog):
- This method is used to listen to the connections associated with the socket.
- The backlog parameter indicates the maximum number of queued connections.
- The maximum value can go up to 5, and the minimum should be at least zero.
- sock_object.accept():
- This function returns (conn, address) pair where ‘conn’ is the new socket object used to send and receive data on the communication channel, and ‘address’ is the IP address tied to the socket on another end of the channel.
- The ACCEPT() method returns a socket object that is different from the socket object created using
socket.socket()
. - This new socket object is dedicatedly used to manage the communication with the particular client with which accept happened.
- This mechanism also helps the Server to maintain the connection with n number of clients simultaneously.
Which Method will You Use for the Client Cocket?
Client Socket Methods
- sock_object.connect():
- This method is used to connect the client to the host and port and initiate the connection to the server.
What are the General Socket Methods Available in Python?
General Socket Methods
sock_object.recv():
- Use this method to receive messages at endpoints when the value of the protocol parameter is TCP.
sock_object.send():
- Apply this method to send messages from endpoints in case the protocol is TCP.
sock_object.recvfrom():
- Call this method to receive messages at endpoints if the protocol used is UDP.
sock_object.sendto():
- Invoke this method to send messages from endpoints if the protocol parameter is UDP.
sock_object.gethostname():
- This method returns the hostname.
sock_object.close():
- This method is used to close the socket. The remote endpoint will not receive data from the other side.
So far, we’ve listed all the socket tools that the ‘socket‘ library provides for Python socket programming. Next, we’ll show you the socket function call workflow to achieve client-server communication. Please refer to the below snapshot. It illustrates every socket call required to establish a channel between client and server.
Python Socket Programming WorkFlow
The below image depicts the calling sequence of the socket methods for both the Client and the Server endpoints.
So from the above flowchart diagram, you would have learned what all socket methods required to create a client/server socket program in Python. Now it’s time to set up the real Python client and server components.
Sample Code for Client-Server Communication in Python
The client-server program will have the following two Python modules.
- Python-Server.py and
- Python-Client.py.
Let’s check out the server code first. For your note, we’ve tested this code on Python 3.
Python-Server.py
This server module will both send and receive data to/from the client.
- The Python-Server.py file has the code to create the server socket which remains in the wait state until it receives a request from the client.
- Whenever a client gets connected, the server accepts that connection.
- The client will then start passing messages to the server.
- Whereas the server will process those messages and send the response back to the client.
- In the below Python socket programming code, we are also asking the user to input the response that he wants to pass to the client.
import socket import time def Main(): host = "127.0.0.1" port = 5001 mySocket = socket.socket() mySocket.bind((host,port)) mySocket.listen(1) conn, addr = mySocket.accept() print ("Connection from: " + str(addr)) while True: data = conn.recv(1024).decode() if not data: break print ("from connected user: " + str(data)) data = str(data).upper() print ("Received from User: " + str(data)) data = input(" ? ") conn.send(data.encode()) conn.close() if __name__ == '__main__': Main()
Python-Client.py
On the client side, we create a socket and connect to the server using the supplied host and port values.
- Client code has a while loop for exchanging messages. It keeps printing all the data obtained from the server.
- After this, there is a call to the input function asking for the client’s response. The response is then passed to the server.
- The user may also enter ‘q’ to stop the communication at any point in time.
import socket def Main(): host = '127.0.0.1' port = 5001 mySocket = socket.socket() mySocket.connect((host,port)) message = input(" ? ") while message != 'q': mySocket.send(message.encode()) data = mySocket.recv(1024).decode() print ('Received from server: ' + data) message = input(" ? ") mySocket.close() if __name__ == '__main__': Main()
How to Run the Client-Server Program?
You’ll need to run both modules from the separate command windows, or you can run them in two different IDLE instances.
First of all, you would execute the server module followed by the client. We’ve given the full Execution Summary of the client-server program.
Python 3.5.1 (v3.5.1:37a07cee5969, Dec 6 2015, 01:54:25) [MSC v.1900 64 bit (AMD64)] on win32 Type "copyright", "credits" or "license()" for more information. RESTART: C:\Users\Techbeamers\AppData\Local\Programs\Python\Python35\Python-Server.py Connection from: ('127.0.0.1', 50001) from connected user: Hello TechBeamers Received from User: HELLO TECHBEAMERS ? Hello Dear Reader from connected user: You posts are awesome :) Received from User: YOU POSTS ARE AWESOME :) ? Thank you very much. This is what keeps us motivated.
Python 3.5.1 (v3.5.1:37a07cee5969, Dec 6 2015, 01:54:25) [MSC v.1900 64 bit (AMD64)] on win32 Type "copyright", "credits" or "license()" for more information. RESTART: C:\Users\Techbeamers\AppData\Local\Programs\Python\Python35\Python-Client.py ? Hello TechBeamers Received from server: Hello Dear Reader ? You posts are awesome :) Received from server: Thank you very much. This is what keeps us motivated. ? q
Check Program Compatibility.
For your note, we’ve tested the above client-server code using the Python 3 release. But you can easily convert the above code to run on Python 2.7. You need to replace the following line of code.
data = input(" ? ")
Use the following Python input function for Python 2.7.
data = raw_input(" ? ")
There are more differences, some of which we are listing below.
- Some of the other functions like print in Python 2.7 don’t require enclosing braces.
- None of Python 2.7’s socket functions,
send()/recv()
need to decode their return values whereas Python 3 requires it.
Suggested Readings
The following are a few tutorials dedicated to the application of socket programming in Python.
1. How to Create a TCP Server-Client in Python
2. Multithreaded Python Server with Full Code
Next, we have several Python tutorials/quizzes/interview questions on this blog. If you like to try them, please click any of the given links.
1. Python Programming Quiz Part-1
2. Python Programming Quiz Part-2
Summary – Python Socket Programming
We hope that the above tutorial will lead you to learn and experiment with Python socket programming. If you indeed found this post useful, then help us spread this knowledge further. To learn more about sockets in Python, you may check from Python’s official website.
Below is a message that defines the relationship between learning and sharing.
Learning leads to success and Sharing brings you recognition.
So, do lend us your support and use the below share buttons to distribute this article on social media.
Happy coding!