Python is one of the most popular object-oriented scripting languages with a programmer-friendly syntax and a vast developer community. This tutorial explains the concept of networking programming with the help of Python classes. Here, we’ll showcase how to write a TCP server and client in Python and implement them using classes.
In our previous Python socket programming tutorials, we’ve already explained the bit-by-bit details of sockets and writing a socket server/client application. Hence, we’ll keep our focus only on the workflow and example code of the Python TCP server and client.
The sample contains the source code for a TCP server and client. For practice, you can extend it to build a small chat system or a local attendance tracking system.
If you are new to socket programming, then you would certainly benefit from reading from the below Python tutorials.
Create TCP Server and Client in Python
To understand the topic in detail, let’s first have a quick look at the socket classes present in the Python SocketServer module. It is a framework that wraps the Python socket functionality. For your note, this component has a new name socketserver
in Python 3.
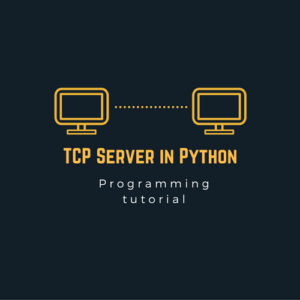
There are two types of built-in classes in the socketserver
module.
Synchronous socket entities
TCPServer class – It follows the (Internet) TCP protocol that allows continuous streams of data between the server and client.
UDPServer class – It makes use of datagrams that contains discrete packets of information. They may go out of order or get dropped in transit.
UnixStreamServer And UnixDatagramServer classes – These classes are similar to the TCP and UDP classes but use Unix domain sockets. Both of them don’t support non-Unix platforms.
The above four classes process the calls synchronously; they accept and treat the requests in a strict sequence. This behavior doesn’t scale if each call takes a long time to complete. It returns a lot of data that the client is not able to process instantly.
The solution is to allow multiple threads to take care of each request. Below is the list of classes to manage each connection on separate threads.
Asynchronous socket entities
- ForkingMixIn class
- ThreadingMixIn class
The socketserver
module has more classes to handle sockets, but we’ve mentioned the most relevant ones to the topic.
Now let’s see the example of the Python TCP Server.
Python-TCP-Server.py
import socketserver class Handler_TCPServer(socketserver.BaseRequestHandler): """ The TCP Server class for demonstration. Note: We need to implement the Handle method to exchange data with TCP client. """ def handle(self): # self.request - TCP socket connected to the client self.data = self.request.recv(1024).strip() print("{} sent:".format(self.client_address[0])) print(self.data) # just send back ACK for data arrival confirmation self.request.sendall("ACK from TCP Server".encode()) if __name__ == "__main__": HOST, PORT = "localhost", 9999 # Init the TCP server object, bind it to the localhost on 9999 port tcp_server = socketserver.TCPServer((HOST, PORT), Handler_TCPServer) # Activate the TCP server. # To abort the TCP server, press Ctrl-C. tcp_server.serve_forever()
In the next example code, you’ll see the Python TCP client module code to communicate with the TCP server.
Python-TCP-Client.py
import socket host_ip, server_port = "127.0.0.1", 9999 data = " Hello how are you?\n" # Initialize a TCP client socket using SOCK_STREAM tcp_client = socket.socket(socket.AF_INET, socket.SOCK_STREAM) try: # Establish connection to TCP server and exchange data tcp_client.connect((host_ip, server_port)) tcp_client.sendall(data.encode()) # Read data from the TCP server and close the connection received = tcp_client.recv(1024) finally: tcp_client.close() print ("Bytes Sent: {}".format(data)) print ("Bytes Received: {}".format(received.decode()))
Execution of Python TCP Server and Client modules
You can run both the server and client in separate Python instances. We recommend that you use Python version 3 for executing the above modules.
Next, you would first run the server module followed by the client. See below the output of both the client and the server.
Python 3.5.1 (v3.5.1:37a07cee5969, Dec 6 2015, 01:54:25) [MSC v.1900 64 bit (AMD64)] on win32 Type "copyright", "credits" or "license()" for more information. RESTART: C:\Users\Techbeamers\AppData\Local\Programs\Python\Python35\Python-TCP-Server.py 127.0.0.1 sent: b'Hello how are you?'
Python 3.5.1 (v3.5.1:37a07cee5969, Dec 6 2015, 01:54:25) [MSC v.1900 64 bit (AMD64)] on win32 Type "copyright", "credits" or "license()" for more information. RESTART: C:\Users\Techbeamers\AppData\Local\Programs\Python\Python35\Python-TCP-Client.py Bytes Sent: Hello how are you? Bytes Received: ACK from TCP Server
Final Word – Create a TCP Server-Client in Python
We always share what we think is useful for our readers like this blog post and other ones in the series of Python socket programming. We hope this Python tutorial and the TCP server example would have served your purpose to visit our blog.
So, do give us your support and share this post using the sharing icons given below.
Lastly, we believe that Python is powerful and it works to the expectations.
Best,
TechBeamers