Automation technology is evolving quite rapidly and so is the Selenium Webdriver. Earlier, we’d Selenium RC, and then came Selenium 2.0 aka Webdriver. And lately, we have seen the official release of Selenium 3.0. Hence, we too have to sync with the trends. So, to continue with the changes, we’ve added a new list of Selenium Webdriver interview questions in this post.
Some of these questions belong to the areas that we haven’t covered in previous posts. And most of them our team recently picked up in real-time from the candidates who shared their views after their interviews. We urge you to read the whole post and do some practice to get the best results.
Our goal is to acquaint you with the new concepts of automation testing in Selenium. In order to boost your skills, you must read the below tutorial at least once after finishing up from here.
Must Read: A Quick and Easy Selenium Python Tutorial
20 Most Recent Selenium Webdriver Interview Questions.
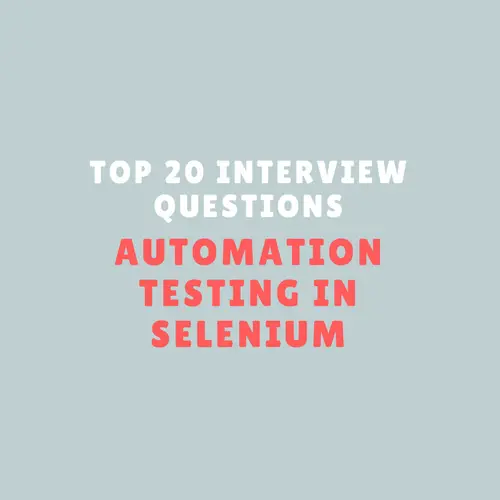
Q-1: Selenium WebDriver and Selenium IDE – Why do you use web driver-backed selenium?
If we have existing Selenium RC test scripts, and we wish to avoid using Selenium RC. Then, instead of using a web driver, we should use web driver-backed selenium which is more realistic in this case.
Q-2: Point out what are the essential features of Selenium Webdriver/ Selenium v2.0.
We should use WebDriver when there is a need to improve the following operations.
- Handling recurring frames/pop-ups, many browser windows, and alerts.
- Page navigation /drag-drop operations.
- Ajax-dependent UI elements.
- Cross-browser testing including improved functionality for the browser (was not fully supported by the Selenium RC).
Q-3: List out the benefits of Webdriver over Selenium RC (1.0) Server.
- If you’ve started using Selenium-WebDriver, then you won’t need the Selenium Server as it uses an entirely different technology.
- Selenium Server (part of 1.0) provides Selenium RC functionality. It intends to support Selenium 1.0 backward compatibility with this feature.
- Selenium Webdriver hits the native browser’s calls for accessing HTML objects whereas the Selenium RC does it via the Selenium server to inject Javascript into the browser.
Q-4: What are the major Browsers that Selenium Webdriver supports?
The Selenium Webdriver framework offers generic APIs that can test your web application with different browsers. We’ve listed some of them next in line.
- Firefox Driver: For Mozilla Firefox browser.
- Internet Explorer Driver: For Internet Explorer browser.
- Chrome Driver: For Google Chrome browser.
- HtmlUnit Driver: GUI-Less(Headless) browser for Java applications.
- Opera Driver: For Opera browser.
Q-5: Selenium WebDriver – What is the difference between “/” and “//” in Xpath?
- Single Slash “/” – With a single slash, we can create an XPath with an absolute path.
- Double Slash “//” – With a double slash, we can build XPath with a relative path, i.e., the XPath would be set up to start selection from anywhere in the document.
Also Read: Latest Selenium Interview Questions for QA Engineers
Q-6: What is the limitation of Webdriver that you may face while adding capabilities for performing tests on a browser that is not supported by the Webdriver?
A major limitation of appending capabilities is that the “findElement” command may not work as expected.
Q-7: Selenium WebDriver – How to check if an element is visible on the web page?
You may need to use the isDisplayed()
method. It returns a boolean type. If the return value is true, it means the element is visible otherwise it’s not.
driver.findElement(By.xpath("XPath of element")).isDisplayed();
Q-8: What are the five different exceptions that the Selenium Webdriver supports?
The five principal exceptions that occur in Selenium Webdriver are.
- WebDriverException
- NoAlertPresentException
- NoSuchWindowException
- NoSuchElementException
- TimeoutException
Q-9: Selenium WebDriver – How do you handle alert popups?
To work with alert dialogs, the first action you should take is to switch the control to alert dialogs then press the “OK” or “Cancel” button. And finally, you can move control back to the web page.
Sample source code.
String webPage = driver.getWindowHandle(); Alert alt = driver.switchTo().alert(); // to move control to alert popup alt.accept(); // to click on ok. alt.dismiss(); // to click on cancel. //Then shift the control back to the main web page driver.switchTo().window(webPage); //to switch back to main web page. String webPage = driver.getWindowHandle(); Alert myAlert = driver.switchTo().alert(); // to switch control to the alert popup myAlert.accept(); // to click on ok. myAlert.dismiss(); // to click on cancel. // Then send the control back to the main web page driver.switchTo().window(webPage); // to switch back to the web page.
Q-10: What is the best approach to switch between frames?
To changeover between the frames, we can use the following Webdriver method.
driver.switchTo().frame();
It can accept any of the below three arguments.
1- A number: It picks the number based on its (zero-based) index.
2- A number or ID: We can choose a frame either by its ID or name.
3- Previously found WebElement: We can use the previously located WebElement to select a frame.
Test Your Skills: Selenium Webdriver Quiz for Software Testers
Q-11: How would you use Selenium Webdriver to upload a file?
You can use the “type” command to write in the file input box of the file upload dialog. Then, you can use the “Robot” class available in JAVA to start the file upload task.
Q-12: In Selenium WebDriver, how do you select an item from a drop-down menu?
We can choose an item from the pull-down menu by Value, by Index, or by Visible Text.
Example:
<select id="metros"> <option value="de">Delhi</option> <option value="mu">Mumbai</option> <option value="ca">Calcutta</option> <option value="ch">Chennai</option> </select>
WebElement metros = driver.findElement(By.id("metros")); Select metro = new Select(metros); //select by value metro.selectByValue("de"); //this will select Delhi from the drop-down //select by index metro.selectByIndex(1); //this will select Mumbai //select by visible text metro.selectByVisibleText("Chennai") //this will select the Chennai
Q-13: Explain how you can find broken images on a page using Selenium Webdriver.
To locate the broken images on a page, you may use the Selenium web driver to retrieve the XPath and fetch all the links on the page using the tag name.
After this, click all the links on the page and check for the 404/500 errors in the target page title.
Q-14: Selenium WebDriver – How do I remove the content of a text box in Selenium 2.0?
WebElement webObject = driver.findElement(By.id("ElementID")); webObject.clear();
Q-15: Describe how you can use the recovery scenario with the Selenium Webdriver.
Recovery scenarios normally depend on the programming language you use. If you are working with Java, then you can try exception handling to overcome the same.
Add the “Try Catch Block” within your Selenium Webdriver Java tests.
Q-16: Is there a way to do drag and drop in Selenium Webdriver?
Yes, you may use the following code to perform the drag-and-drop operation.
Actions dummyAction = new Actions(driver); WebElement startPoint = driver.findElement(By.cssSelector(“div.source”)); WebElement endPoint = driver.findElement(By.cssSelector(“div.target”)); dummyAction.dragAndDrop(startPoint,endPoint).perform();
Q-17: How would you count the Elements on a Webpage with Java & Selenium Webdriver?
Selenium RC (1.0) uses the ‘getXpathCount’ method to identify the number of XPath attributes on the webpage.
But, in Selenium (2.0) webdriver, we can achieve it in the following way.
int xpathCount = webDriver.findElements(By.xpath("Valid_Xpath")).size();
Q-18: Explain how can the Webdriver imitate the double click.
You can perform the “double-click” simulation by using the below command.
Syntax-
Actions actionObject = new Actions (driver); actionObject.doubleClick(webelement);
Try This Test: Selenium Online Practice Test with 30 Questions
Q-19: Selenium WebDriver – What is the recommended method to capture a screenshot using WebDriver?
File screenshot = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE); FileUtils.copyFile(screenshot, new File("c:\\screenshot.png"));
Q-20: Selenium WebDriver – How to login into any site if it is showing any authentication pop-up for username and password?
Pass the username and password with the URL.
Syntax-
http://username:password@url e.g.- http://techbeamers:password@somesite.co.in
If you love solving programming challenges, then why not try these 10 Selenium coding exercises? These exercises will test your knowledge of Selenium WebDriver and help you improve your automation skills.
Summary – Selenium Webdriver Interview Questions for Testers.
Hopefully, the above list of top Selenium Webdriver interview questions will help you prepare for your interview in the new year.
However, there is a lot of other Selenium learning stuff available on our blog; it will certainly help you in improving your Selenium test automation skills.
Best,
TechBeamers.