Welcome to the Python tutorial, today we’ll dive into the concept of serializing Python objects. Serialization is a universal concept and almost all the programming languages provide the mechanism to implement it. In this post, we’ll thoroughly explain the step-by-step process for enabling the Serialization of Python objects.
Introduction to Serialization
In object-oriented programming, Serialization is the process of transforming data structures or objects into a format that can be offloaded to a file, or memory cache or transmitted over the network connection and the same object can be reconstructed later in the same or different environment.
Serialization is converting the object into a stream of bytes also called marshaling an object, and the reverse process of rebuilding the object back from the stream of bytes is deserialization or unmarshalling.
The process of Serializing Python Objects
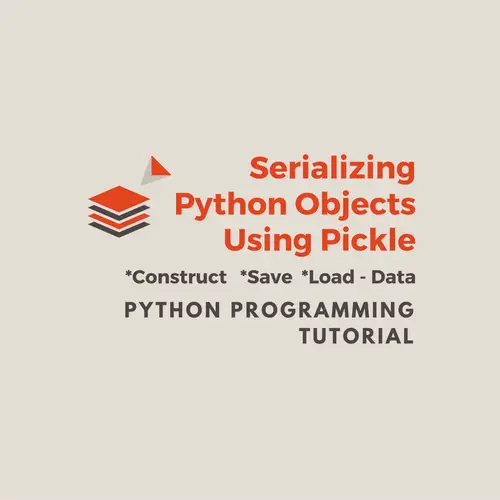
In Python, serialization and deserialization are achieved through the “Pickle” library.
What is Pickle and how to use it?
Pickle library is developed using the C programming language like the Python interpreter is. It can save arbitrarily complex Python data structures. Pickle is extensible, cross-version, and not very secure (not secure against erroneous or maliciously constructed data).
What data type can the Pickle module store?
The pickle module stores the following data types:
- All the native data types that Python maintains: Booleans, integers, floating point numbers, complex numbers, strings, byte objects, byte arrays, and None.
- Lists, tuples, dictionaries, and sets holding any sequence of native data types.
- Lists, dictionaries, tuples, and sets with the following variations.
- Sets carrying any combination of lists/tuples/dictionaries, and
- Sets enclosing any combination of native data types (and so on, to the maximum nesting level that Python allows).
- Functions, classes, and the instances of classes (with limitations).
Pickle has two primary methods. The first one is a dump that drops an object to a file. The second method is the load that loads the object from a file object.
How to use the Pickle library for serializing Python objects?
Step#1 Construct Pickle Data.
We will use dictionary-type data for pickling that contains the information related to our website:
website = {'title' : 'Techbeamers', 'site_link' : '/','site_type': 'technology blog','owner':'Python Serialization tutorial','established_date':'Sep2015'}
Step#2 Saving data as a pickle file
Now, we have a dictionary that has all the information about the website. Let’s save it as a pickle file:
import pickle with open ('website.pickle','wb') as f: pickle.dump(website,f)
- We’ve used the “wb” file mode to open the file in binary mode for the write operation.
- Enclose it using a “with” statement to make sure the file is automatically closed after we are done with it.
- The dump() method in the pickle module takes a serializable Python data structure, in this case, the dictionary created by us and performs the following operation.
- Serializes it into a binary format using the latest version of the pickle protocol.
- Saves it to an open file.
- For your information, the pickle is a protocol that is Python-centric. There is no surety of cross-language compatibility.
- The most recent version of the pickle protocol requires a binary format. So, please make sure to open the pickle files only in binary mode. Otherwise, the data will get corrupted while writing.
Step#3 Loading data from the Pickle file
Following is a piece of code that will load the data from the pickle file.
import pickle with open ('website.pickle', 'rb') as f: data = pickle.load(f) print (data)
Output: {'site_link': '/', 'title': 'Techbeamers', 'owner': 'Python Serialization tutorial', 'established_date': 'Sep2015', 'site_type': 'technology blog'}
- From the above code fragment, you can check that we’ve opened the
‘website.pickle’
file that was created after formatting the Python dictionary data type. - Since the pickle module supports the binary data format, we’ve opened the pickle file in binary mode.
- The
pickle.load()
method accepts the stream object as a parameter and performs the following operations.- Scans the serialized buffer from the stream.
- Instantiate a brand-new Python object.
- Rebuilds the new Python object using the serialized data, and returns the renewed object.
- The
pickle.dump()
and pickle.load() cycle forms a new data structure that is identical to the original data structure.
In the end, we’ve consolidated all the pieces of the code mentioned above and presented the unified structure below.
import pickle website = {'title' : 'Techbeamers', 'site_link' : '/','site_type': 'technology blog','owner':'Python Serialization tutorial','established_date':'Sep2015'} with open ('website.pickle','wb') as f: pickle.dump(website,f) with open ('website.pickle', 'rb') as f: data = pickle.load(f) print (data)
So, that was all we wanted to convey about the Serialization concept in Python. Hope you would have liked it.
Next, we had a number of Python tutorials/quizzes/interview questions on this blog. If you like to try them, please just go ahead.
You might wanna check out our tutorial on Python Class Methods.
Final word
We always try to cover concepts that are important from the language perspective and crucial for the interview purpose. That’s why we delivered this post on serializing Python objects. If you want us to cover any topic of your choice, then please send us your request using the comment box section.
If this post was able to gain your attention, then please do share this article on social media and with your friends.
Keep Reading & Continue Improving,
TechBeamers