Here are the latest Java collection interview questions for your reference. Java Collections Framework is pivotal in developing high-performance, reusable, and reliable Java applications.
Our team prepared this list after analyzing the question pattern of many IT companies hiring Java programmers. If you like to test your Java coding skills, then try to get through the 30 Java coding interview questions.
Fact – Java programming language is the brain-child of James Gosling who designed it in early 1995.
Check out the top Java collection interview questions.
Top Java Collection Interview Questions
If you are preparing for a Java interview, we suggest that you study these topics carefully. You should also be able to answer questions about the specific collection types that are used in the project or company that you are interviewing for.
- The most common Java collection interview questions are about the following topics:
- List
- Set
- Map
- Array
- LinkedList
- Interviewers often ask questions about the following:
- Differences between different collection types
- How to choose the right collection type for a given task
- How to use collection methods such as add(), remove(), contains(), and get()
- How to iterate over collections
Q-1. How does the Collection Framework empower developers with its unique functionality and applications?
Java Collection Framework (JCF) represents a set of interfaces and classes that provide efficient ways to store and handle data in a Java application.
It includes several classes that support the following operations:
- Searching,
- Sorting,
- Insertion,
- Manipulation, and
- Deletion.
Q-2. What are the main benefits of the Collection Framework?
There are actually four things that make Java Collections Framework extremely useful for programmers.
Performance – Collection classes are highly efficient data structures to improve speed and accuracy.
Reusability – Collection classes can intermix with other types to promote reusability.
Maintainability – Supports consistency and interoperability in the implementation and hence makes the code easy to maintain.
Extensibility – Allows customization of primitive collection types as per the developer’s requirements.
Q-3. What is the structure of the Collection Framework in Java?
Below is the diagram picturing the different collection classes and interfaces.
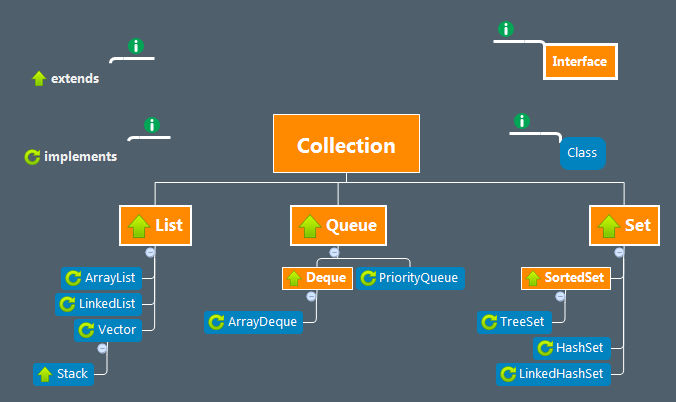
Q-4. What is the difference between Collection and Collections Framework?
Collection – A collection is an object container that can hold multiple elements and functions as a single unit.
Collections Framework – The Collections framework provides a centralized system for managing and representing collections.
Q-5. What is the difference between a Collection
and Collections
?
The following two points clearly state the differences:
- The Collection is an interface while Collections is a Java class.
- Both the Collection and Collections are part of <java.util> package and are the main components of the Java Collection Framework.
Q-6. What is the root interface in the collection hierarchy?
It is the Collection interface which is the root interface in the collection hierarchy. However, a few may disagree and will say that the Collection interface extends the Iterable interface. So Iterable must be the root interface.
But you can make the counter-argument by saying that the Iterable interface is a part of the <java.lang> package, not of the <java.util> package.
Also, Oracle’s Java collections documentation states that the Collection interface is a member of the Java Collections framework which belongs to the <java.util> package.
Q-7. What are the primary interfaces in the Java Collections Framework?
Java Collections Framework is composed of five primary interfaces.
Collection Interface – Most of the collections in Java inherit from the Collection interface. It is the core of the collection framework and stays at the root of the Collection’s hierarchy.
Set Interface – It is a mathematical interpretation of a Set which doesn’t allow duplicate entries. Also, a set doesn’t define an order for the elements and hence doesn’t support the index-based search.
Queue Interface – This interface follows the principles of a queue and stores elements in the same order as they enter. Operations like addition will take place at the rear and removal from the front.
List Interface – A list is an extended form of an array.
Lists are ordered and can also contain duplicate elements. Not only does it allow index-based search, but insertion can also be done independently of the position.
Map Interface – A Map is a two-dimensional data structure that stores data in the form of a Key-Value pair. The map is necessarily a set and can’t have duplicate elements.
In a map, the value represents an element whereas the key is the hash code of the same item.
Q-8. Which are the collection classes that are synchronized or thread-safe?
The Following Collection classes are thread-safe and support synchronization. You can use them safely in a multi-threaded Java application.
Hashtable – A Hashtable is a collection class that stores lists as array elements also known as buckets. It associates the keys with values. None of the keys or values can be null. Instead, the keys are objects that are hashed to compute the hash code. The resultant works as the index to store the value within the table.
Vector – A Vector class serves as a dynamic array. It behaves like ArrayList with two main differences. The first is that a Vector is synchronized and secondly, it brings in some additional methods which are not part of the Collections Framework.
Fact – A study reveals that more than 3 billion devices run on Java-based platforms.
Q-9. What is the primary difference between a List and a Set?
List vs. Set.
- Set holds unique elements whereas List can have duplicate items.
- The Set is an unordered collection whereas the List is the ordered one.
- The List preserves the order of the object addition.
Q-10. What is the principal difference between a Map and a Set?
Map objects use unique keys to hold values whereas Set contains unique values.
Q-11. Which are the classes that implement the List and Set interface?
The following classes implement the List interface.
- ArrayList,
- Vector, and
- LinkedList.
The following classes implement the Set interface.
- HashSet, and
- TreeSet
Q-12. What is an Iterator in the Java Collections Framework?
The Iterator is an interface. You can locate it in the <java.util> Package. It provides methods to iterate over any Collection.
Q-13. What are the principal differences between Iterator and Enumeration?
Iterator:
- Enumeration functions like a read-only interface as it only allows one to traverse and retrieve the object.
- Enumeration is doubly faster than the Iterator and uses very little memory.
- Enumeration is very necessary and fulfills the primary requirements.
Enumeration:
- The Iterator is much safer than Enumeration as it always blocks other threads to alter the collection object.
- Iterator gives the ability to remove elements using the remove() method whereas Enumeration doesn’t.
- Iterator method names are more logical to make their functionality clear.
Q-14. What is the design pattern that Iterator uses?
- It uses the iterator design pattern. The iterator design pattern enables the ability to sail through the collection of objects by using a standard interface abstracting the underlying implementation.
- Enumeration is also an example of the Iterator design pattern.
Q-15. What are the methods to override for you to use any object as a key in HashMap?
To use an object as the key in HashMap, you can implement equals() and hashCode() methods.
Q-16. What is the main difference between Queue and Stack?
The Queue is a type of data structure that follows the FIFO (first in first out) mechanism.
An example of a Queue in the real world is casting your votes in the electoral center.
The Stack is a data structure that follows the LIFO (last in first out) mechanism.
A real-time example of Stack is when a person wears a bracelet set, the last bracelet worn is the first to get removed, and the opening bracelet you would take out in the end.
Fact – 95 percent of Software Enterprises use Java for development.
Q-17. What is the best way to reverse a List in Collections?
The Collections class provides a reverse(List list) method which accepts the list as a parameter.
e.g. Collections.reverse(ListOfObject);
Q-18. Which approach would you choose to convert the array of strings into the list?
There is one method in the Arrays class of <java.util> package as List() which takes the array as its parameter.
List<String> arrayList = new ArrayList<String>(); arrayList.add("techbeamers") String [] strArr = arrayList.toArray(new String[arrayList.size()]);
Q-19. The Collection interface doesn’t have a hasNext() or next() method. Can you explain why?
The Iterator interface does have the hasNext(), and next() methods, but the Collection interface doesn’t have them.
The Collection interface could have the hasNext(), and next() methods but more methods would have needed to reset the “built-in” iterator to allow iteration from the beginning. Such a mechanism would need two iterators while the Collection would only have one at any given time.
Hence, it doesn’t make sense to add it to the interface that everyone has to implement.
Q-20. What is the difference between the Iterator and ListIterator?
- You can use Iterator to traverse the collections like Set and List whereas ListIterator you can utilize with Lists only.
- Using Iterator, you can cross in the forward direction only whereas ListIterator supports traversing in both directions.
- ListIterator inherits from the Iterator interface and brings add-on functionalities like the ones listed below.
- Adding an element,
- Replacing an item, and
- Retrieve the index position for the previous and next.
Q-21. What is the significance of the fail-fast property in Java iterators?
- The iterator fail-fast property verifies any change that occurs in the structure of the underlying collection whenever you try to get the next element.
- If it detects any change, it throws the ConcurrentModificationException.
- All the Iterator implementations in the Collection classes are fail-fast by design except the concurrent collection classes like ConcurrentHashMap and CopyOnWriteArrayList.
Q-22. What is the difference between Fail-fast and Fail-safe?
- Fail-fast is nothing but the mechanism to support the instant reporting of any failure and whenever a problem occurs fail-fast system fails.
- In Java, a fail-fast iterator may throw concurrent modification exceptions whereas a fail-safe iterator won’t.
- The exception can occur due to one of the two reasons given below.
- When one of the threads is iterating through the collection while the other one is trying to modify it
- Or after invoking the remove() method if you attempt to change the collection object
Q-23. What are the different ways to sort a list of Objects?
The following methods can be applied to perform sorting.
- To sort the array of objects, you can use Arrays.sort() method.
- When you need to sort a collection of objects, then use Collections.sort() method.
Q-24. How does HashMap work in Java?
HashMap follows the concept of hashing. It employs the put(key, value) and the get(key) methods to manage (store and retrieve) the objects from the HashMap.
When we call the put() method with a key and value object, the HashMap mechanism triggers the hashCode() method on the principal object and returns the hash value. Then, the hashing function locates a bucket location using the hash value where it can save the key-value pair in the form of a nested class called Entry (Map. Entry).
When you need to fetch a value from the HashMap, you call the get() method with the desired key as the parameter. The critical object produces the same hash value which leads to the same bucket location where the object was stored in the put() method.
Q-25. What is the difference between HashMap and Hashtable?
- Synchronization or Thread Safe
- Null keys and null values
- Iterating the values
- Default Capacity
Q-26. Why doesn’t the Map interface extend the Collection interface?
- The Set is an unordered collection and does not allow duplicate elements.
- The List is an ordered collection that allows duplicate elements whereas the Map is a key-value pair.
- It is viewed as a set of keys and a collection of values.
- A Map is a collection of key-value pairs so by design, they are separated from the collection interface.
Q-27. What is the use of the Properties class?
The Property is a subclass of Hashtable. You can use it to manage the list of values using the keys, both the key and value are of String type.
Fact – Java tops the list of most popular programming languages as per the latest TIOBE index.
Q-28. How to remove the redundant elements from an ArrayList object?
Here is the method to remove the redundant element from the ArrayList.
- Offload all of the ArrayList elements to a LinkedHashSet.
- Empty the ArrayList.
- Copy all elements of LinkedHashSet to an ArrayList.
You can refer to the below example for help.
import java.util.ArrayList; import java.util.List; import java.util.LinkedHashSet; public class DeleteDuplicates { public static void main(String[] args) { /* Creating an ArrayList of Strings and adding * all elements to it */ List<String> arrlst = new ArrayList<String>(); arrlst.add("tech"); arrlst.add("beamers"); arrlst.add("java"); arrlst.add("python"); arrlst.add("selenium"); arrlst.add("shell"); // Displaying the ArrayList elements System.out.println("Before:"); System.out.println("ArrayList contains: "+arrlst); // Creating a LinkedHashSet LinkedHashSet<String> linkhs = new LinkedHashSet<String>(); /* Adding ArrayList elements to the LinkedHashSet * in order to remove the duplicate elements and * to preserve the insertion order. */ linkhs.addAll(arrlst); // Removing the ArrayList elements arrlst.clear(); // Adding the LinkedHashSet elements to the ArrayList arrlst.addAll(linkhs); // Displaying ArrayList elements System.out.println("After:"); System.out.println("ArrayList contains: "+arrlst); } }
Summary – Essential Java Collection Interview Questions
We hope that the preceding Java interview questions have aided you in accelerating your preparation, leading to a favorable outcome.
If you desire to delve deeper into the intricacies of the collection framework, we invite you to partake in the following Java collection quiz and assess your proficiency.
Finally, if you found the Java collection interview questions worth your time, then extend your support and share this post.
Best,
TechBeamers