This post is next in the sequence of our last article on working with Python sockets. In the previous post, we demonstrated a TCP server in Python accepting and responding to requests from a single TCP client. Now, we want to share the implementation of a Multithreaded Python server that can work with multiple TCP clients.
Develop a Multithreaded Server in Python
The Multithreaded Python server uses the following main modules to manage the multiple client connections.
1. Python’s threading module.
2. SocketServer‘s ThreadingMixIn.
The 2nd class out of the above two modules enables the Python server to fork new threads to take care of every new connection. It also makes the program to run the threads asynchronously.
Before we move on to checking the code of the threaded socket server, we suggest you read our previous post. It gives full information on Python sockets and illustrates a single-threaded server program.
Must Read: Python Socket Programming with Examples
Now let’s come back to today’s topic. In this section, we’ll show you the threaded socket server code followed by the source code of two TCP clients.
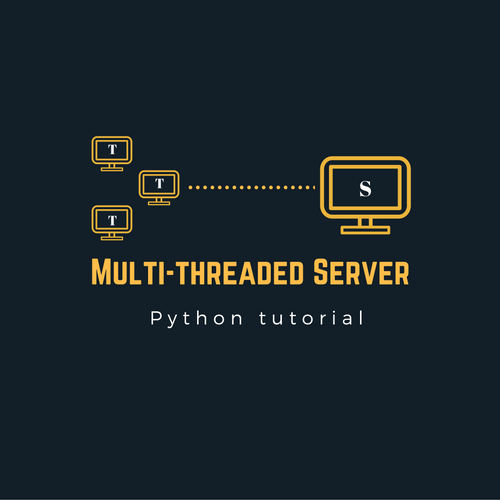
This Multithreaded Python server program includes the following three Python modules.
1. Python-Server.py
2. Python-ClientA.py
3. Python-ClientB.py
Python-Server.py
import socket from threading import Thread from SocketServer import ThreadingMixIn # Multithreaded Python server : TCP Server Socket Thread Pool class ClientThread(Thread): def __init__(self,ip,port): Thread.__init__(self) self.ip = ip self.port = port print "[+] New server socket thread started for " + ip + ":" + str(port) def run(self): while True : data = conn.recv(2048) print "Server received data:", data MESSAGE = raw_input("Multithreaded Python server : Enter Response from Server/Enter exit:") if MESSAGE == 'exit': break conn.send(MESSAGE) # echo # Multithreaded Python server : TCP Server Socket Program Stub TCP_IP = '0.0.0.0' TCP_PORT = 2004 BUFFER_SIZE = 20 # Usually 1024, but we need quick response tcpServer = socket.socket(socket.AF_INET, socket.SOCK_STREAM) tcpServer.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) tcpServer.bind((TCP_IP, TCP_PORT)) threads = [] while True: tcpServer.listen(4) print "Multithreaded Python server : Waiting for connections from TCP clients..." (conn, (ip,port)) = tcpServer.accept() newthread = ClientThread(ip,port) newthread.start() threads.append(newthread) for t in threads: t.join()
Python-ClientA.py
# Python TCP Client A import socket host = socket.gethostname() port = 2004 BUFFER_SIZE = 2000 MESSAGE = raw_input("tcpClientA: Enter message/ Enter exit:") tcpClientA = socket.socket(socket.AF_INET, socket.SOCK_STREAM) tcpClientA.connect((host, port)) while MESSAGE != 'exit': tcpClientA.send(MESSAGE) data = tcpClientA.recv(BUFFER_SIZE) print " Client2 received data:", data MESSAGE = raw_input("tcpClientA: Enter message to continue/ Enter exit:") tcpClientA.close()
Python-ClientB.py
# Python TCP Client B import socket host = socket.gethostname() port = 2004 BUFFER_SIZE = 2000 MESSAGE = raw_input("tcpClientB: Enter message/ Enter exit:") tcpClientB = socket.socket(socket.AF_INET, socket.SOCK_STREAM) tcpClientB.connect((host, port)) while MESSAGE != 'exit': tcpClientB.send(MESSAGE) data = tcpClientB.recv(BUFFER_SIZE) print " Client received data:", data MESSAGE = raw_input("tcpClientB: Enter message to continue/ Enter exit:") tcpClientB.close()
How to run the Multithreaded Python server program.
For your note, please run the above three modules with the Python 2.7 version. Because the above code is compatible with Python 2.7.
However, you can always convert this demo to run with Python 3. You need to make a few changes as mentioned below.
- The print function in Python 3 requires wrapping the input arguments in brackets.
- The string methods accept input either in a decoded or encoded format.
Footnote – A Multithreaded Server in Python
Threading can make any program run faster. But it also increases the code complexity. So if you are finding it hard to understand, then adding more logs will help to check what’s happening inside.
Next, please share your feedback about the post. Also, if you know a better way of creating a Multithreaded Python server then do write to us.
In case you want more micro-level details, follow our Python socket programming guide or refer to the online documentation.
Lastly, if you liked the above tutorial, then help us reach a larger audience. Follow our social media accounts and share our tutorials with yours.
Keep learning,
TeachBeamers