Presenting you here with a 1-10 Random Number Generator purely developed in JavaScript with just a few lines of code. You can run it and feel it practically. Just, provide a start value and ending number to specify a range. And, the random number generator will deliver a unique value every time. It shows 1 and 10 as the default range but it can actually accept large values. Try it now from the above.
Random Number Generator
Random number generation is a fundamental task in computer science. And, they offer logic to support in a variety of applications, including games, simulations, and cryptography.
In this tutorial, we will learn how to generate a random number between 1 and 10 using JavaScript. We will also discuss some of the different types of random numbers and their uses.
What is a random number?
A random number is a unique number that is impossible to predict by design. In other words, there is no way to know what the next random number will be.
Several mechanisms are now present to generate random numbers. One common method is to use a pseudorandom generator (PRNG). It is a computer algorithm that generates a sequence of numbers that appear to be random. However, PRNGs are not entirely random, as the sequence of numbers uses a seed value for the generation task.
Types of random numbers
There are two main types of random numbers:
- Uniform random numbers: We produce uniform random numbers with an equal likelihood for each value. For instance, when generating a random number between 1 and 10 with a uniform distribution, each number has an equal chance of appearing in the result.
- Non-uniform random numbers: We create non-uniform random numbers with varying probabilities. For example, when generating a random number between 1 and 10 with a non-uniform distribution, number 10 is more likely to appear in the output than number 1.
Uses of random numbers
Random numbers serve a key role in a variety of applications, including:
- Games: Random numbers are part of the business logic in games. For instance, to generate random emails, such as the dice roll in a game of craps or the shuffle of the deck in a game of cards.
- Simulations: Random numbers also have a role in simulations to model real-world phenomena, such as generating random images or predicting the behavior of a financial market.
- Cryptography: Random numbers play a crucial role in cryptography to generate enc. keys and other security parameters.
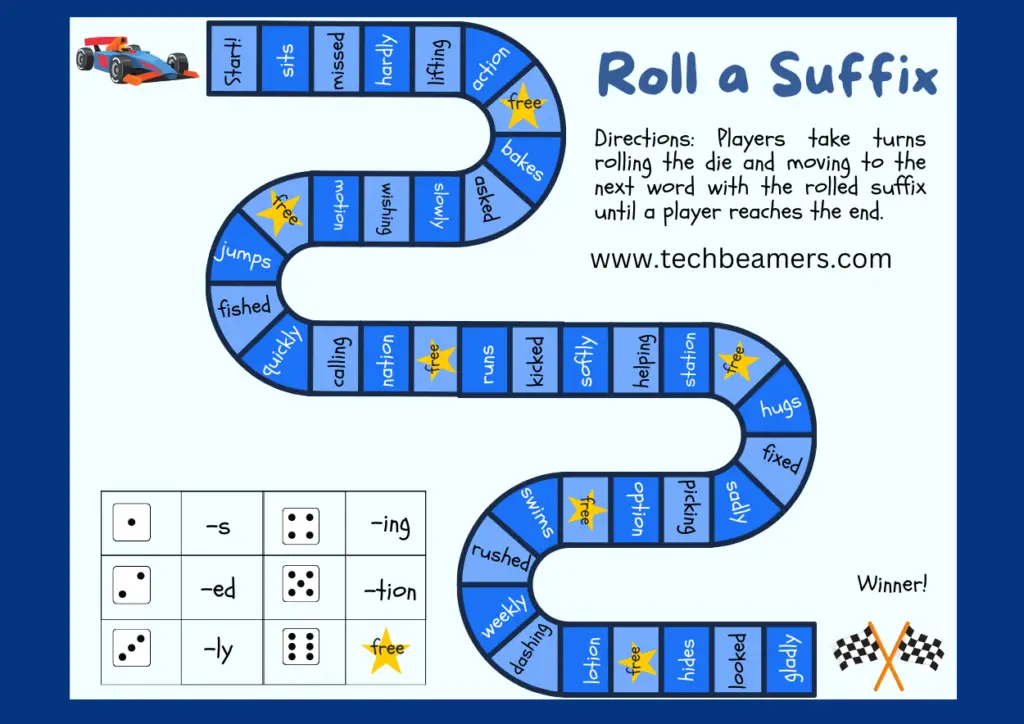
1-10 random number generator JS code
To generate a random number between 1 and 10 in JS, we can use the following methods:
1. Using Math’s random()
JavaScript has many global objects and Math is one of them. It provides many mathematical constants and functions. Check the below code using Math.random()
to generate a random number between 0 and 1:
JS code:
function genRandNum() {
return Math.floor(Math.random() * 10) + 1;
}
The Math.random()
function generates a random number between 0 and 1. We then multiply this number by 10 and round it down to the closest integer. This gives us a random number between 1 and 10.
2. Using Window’s crypto
The window’s crypto object is part of the Web Cryptography API. It offers a number of cryptographic functions. It’s one of the primary use cases is to generate secure random numbers.
An example of using window’s crypto is as follows:
function genRandNum() {
const arr = new Uint32Array(1);
window.crypto.getRandomValues(arr);
return arr[0] % 10 + 1;
}
Finally, here is an example of how to use the genRandNum()
function. You can try any of its definitions from the above.
JS code:
const randNum = genRandNum();
console.log(randNum); // Output: 5 or any value
Data and research about random numbers
Random numbers solve many use cases in a wide variety of fields, including computer science, mathematics, statistics, and physics. There is a large body of research on random number generation and its applications.
Here are some interesting facts about random numbers:
- Have a major role in generating the winning lottery numbers.
- Mimic noise generation in digital images and videos.
- In video games, random numbers are like rolling dice to add surprise and fun.
- In the creation of secure keys and codes to protect our online data.
1-10 random number generator use cases
Here are some use cases for a 1-10 random number generator:
- Generating a random number for a game, such as a dice roll or a coin flip.
- Generating a random sample of data for statistical analysis.
- Simulating a real-world process, such as the spread of a disease or the behavior of a financial market.
- Generating random numbers for cryptography, such as generating encryption keys.
Conclusion
Generating a random number between 1 and 10 is a simple task in JavaScript. The Math.random()
function can be used to generate a random number between 0 and 1, which can then be multiplied by 10 and rounded down to the closest integer.
Given the above points, you must have realized that random numbers have both scientific and business value. They cater to a wide range of apps, including games, simulations, and crypto. Finally, we hope this tutorial was helpful in expanding your knowledge of random numbers.
Happy coding!