This tutorial outlines the simple steps to write your first C program. You will learn what the fundamental components that make it work are.
Your First C Program in Simple Steps
Below is the C program coding snippet which prints “Hello Computer” on the console. You can use any text editor to write this code. But we recommend and use Codeblocks to create, compile, and execute any C program.
#include<stdio.h> int main() { printf("Hello Computer"); getchar(); return(0); }
C Program Structure
Let’s now see what every individual line in the above code means.
Include Statement – #include<stdio.h>
In the big brain of C, there are some pre-defined instructions to help users execute commands faster. One example of that is the “printf” instruction, which we will see soon. There are specific libraries in C that contain information on these instructions. Therefore, “#include<stdio.h>” tells the computer to include the information of these pre-defined instructions so that you can use them in your programs. “stdio” stands for “standard input-output.”
Note: You can observe what happens when you do not write this line. It will show an error that the function “printf” is not defined. It is because the compiler does not know what it means unless you provide the header file “#include<stdio.h>” which has its definition.
Entry Function – int main()
Every C program has a set of instructions to be executed. We usually write them inside the body of the main() function. It is the entry point routine for all programs to begin execution. From the main function, we call all other functions to perform a set of tasks. So, there has to be a “main” function in a C program.
In C programming, the main always returns an integer type value. The “int” before the main represents the type of value returned.
A C program can also have user-defined functions which we’ll cover in the later tutorials. However, in C programming, using the “main” is compulsory.
Note: You may have noticed that after every function, we give parentheses (). It is because while calling, the program can pass a set of values or parameters to it. They also send back data using the “return” statement. If there is nothing to return, then we may specify the 0 as a return value or not use it at all.
Print a Message – printf()
It is a pre-defined function in C that tells the computer to display the text as it is. It helps the compiler to differentiate between command and actual text intended for printing. The text inside the parentheses should appear as it is on the screen
Note: In the printf() function, the actual text to be displayed should be enclosed in double inverted commas “_.” Something like printf(“Hello Computer”).
Wait for User Input – getchar()
It is a C input function that makes the program wait until the user presses a key.
Exit from the C Program – return(0)
It represents the return code of the main() function. A non-zero value means error whereas the zero indicates the program gets executed successfully.
In our example, we return a zero value which evaluates success.
The curly braces {}
The curly brackets let us create multiple units of code in a C program.
They define the boundaries of a function such as the main() and include instructions for execution in a C program.
A function can have multiple nested blocks of code delineating using curly brackets. Each such section has access to variables from the parent block, but any of its variables will not be visible from the outside.
We’ll provide a detailed description of the variable scope in the next classes.
Execute the Code
Now that we have successfully understood what every line means. Let us type this code on our newly set up IDE and Run it.
When you type this code and run, you should see something like this.
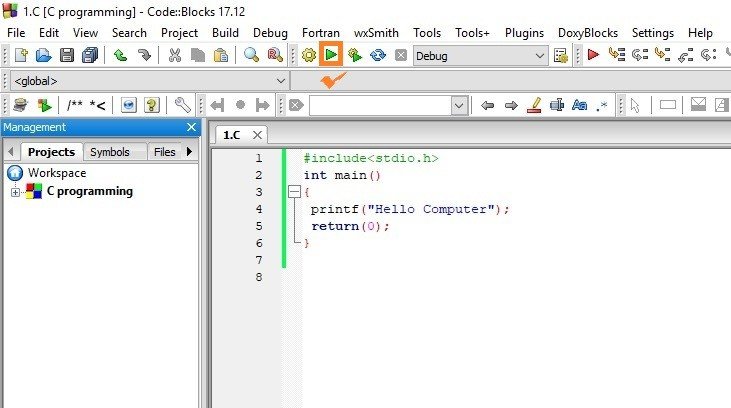
Now click on the upper green triangle, and you should see a screen like this after a few seconds.
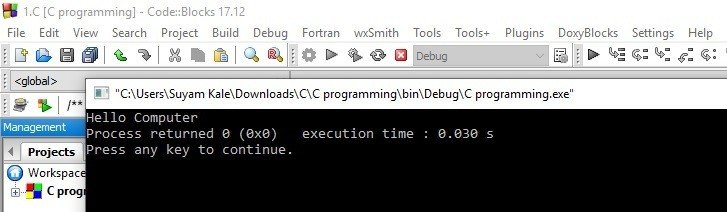
Congratulations, You have successfully written, compiled, and run a C program. By the way, if you love using the Linux command line, then go through our makefile tutorial to compile your first C program.
Lastly, our site needs your support to remain free. Share this post on social media (Linkedin/Twitter) if you gained some knowledge from this tutorial.