Structs in C are a powerful feature of the C programming language. They allow you to create complex data types by grouping different variables under a single name. In this tutorial, we’ll guide you through the syntax of C structs, providing a detailed understanding of their usage and demonstrating unique and situational examples.
How to Use Structs in C Programming Language
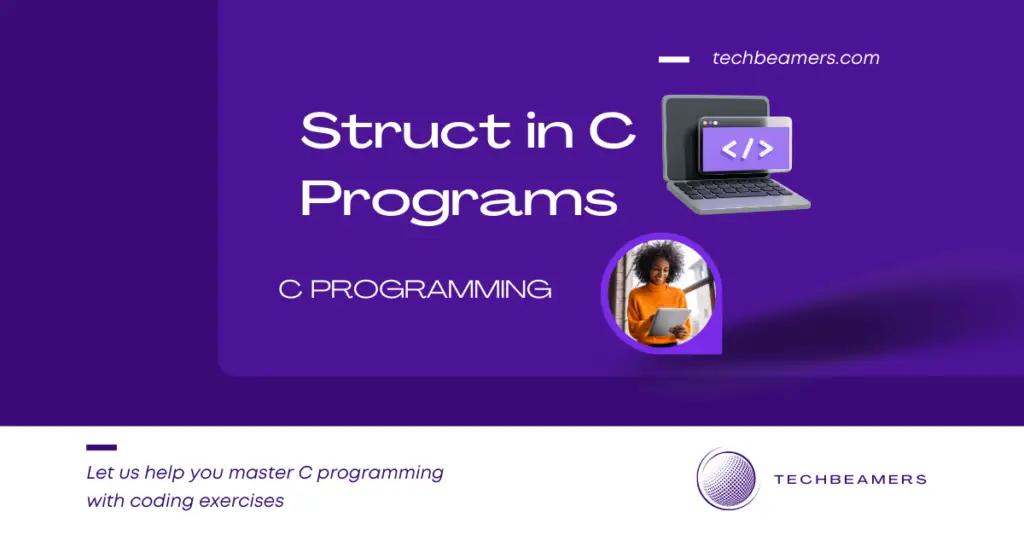
What is a Struct in C?
In C programming language, a struct, or “structure,” is a custom composite data type. It lets you group variables of different types under one name. A struct in C allows you to create a record, which groups different but related pieces of information. This record serves as a container for things that belong together.
Syntax of C Structs
In C, the syntax for declaring a struct involves using the struct
keyword followed by the struct’s name and a block of members enclosed in curly braces. Each member is defined with its data type and an optional name.
Creating a Struct
The syntax starts with the keyword struct
followed by the struct’s name and a block of members enclosed in curly braces.
struct Person {
char name[50];
int age;
float height;
};
Here, we create a Person
structure to store information about an individual, including their name, age, and height.
Defining Struct Members
Inside the curly braces, you define the members of the structure, each with its data type.
struct Book {
char title[100];
char author[50];
int pages;
float price;
};
In this example, the Book
struct has members like title
, author
, pages
, and price
to represent book details.
Declaring Struct Variable
After defining a struct, you can declare variables of that type and initialize them.
struct Image {
int width;
int height;
};
struct Image testImage = {100, 200};
Here, we define a Image
struct to represent coordinates and initialize a testImage
variable with values (100, 200).
Accessing Struct Members
To access members of a structure, you need to use the dot (.
) operator to specify the struct variable and its member.
struct Car {
char brand[20];
int year;
float price;
};
struct Car myCar = {"Toyota", 2022, 25000.50};
printf("Car Brand: %s\nYear: %d\nPrice: $%.2f", myCar.brand, myCar.year, myCar.price);
Nested Structs
Structs can be nested, allowing you to create more complex data structures. Check the below example:
struct Address {
char street[50];
char city[30];
int zipCode;
};
struct Employee {
char name[50];
struct Address empAddress;
float salary;
};
In this scenario, a Employee
struct includes an embedded Address
struct, providing a way to represent an employee’s details along with their address.
Why Use Structs in C Programs?
In C programming, structs act as organized containers for your data. Picture a structure as a way to group related information efficiently. Here’s why structs are essential, especially for beginners:
Custom Data Types
With structs, you craft your custom data types in C. It’s akin to creating a specialized data superhero for your program.
struct Student {
char name[50];
int age;
float grade;
};
Organize Your Data
Structs systematically arrange your data. Instead of managing loose variables, you consolidate related information. Think of it as compartmentalizing your toolkit.
struct Float {
int fractional;
int integer;
};
Easy Data Access
Once you define a structure, accessing data becomes straightforward. It’s akin to reaching for a specific tool in a well-organized toolbox.
struct Student newStudent;
strcpy(newStudent.name, "Soumya");
newStudent.age = 20;
newStudent.grade = 3.8;
Enhance Code Clarity
Structs improve the clarity of your code. When someone reads your code, they swiftly grasp the purpose of each “container.”
Prevent Confusion
No more confusion with scattered variables. If it’s a student, you know it will have a name, age, and grade. Imagine labeling your storage boxes.
Write Better Programs
Structs make coding clear and simple. They organize code for better understanding and management, contributing to straightforward programming practices.
Sample C Struct Examples
Here are some sample C structure examples for illustration purposes.
Simple C Structure Program
Let’s create a simple program that defines a structure (Car
) to represent car details. It creates a car variable (myCar
) and assigns values to its members. After that, it prints the car details, including the brand, year, and price.
#include <stdio.h>
#include <string.h>
// Declaration of a simple structure
struct Car {
char brand[20];
int year;
float price;
};
int main() {
// Creation of a struct var
struct Car myCar;
// Fetch structure members
strcpy(myCar.brand, "Toyota");
myCar.year = 2022;
myCar.price = 25000.50;
// Print struct members
printf("Car Details: %s %d, $%.2f\n", myCar.brand, myCar.year, myCar.price);
return 0;
}
Copy the above code and run it in our online C programming compiler.
Program to Track Inventory Items
Consider a situation where you need to manage inventory items in a store. Utilize the keyword struct
and create a structure representing each item.
#include <stdio.h>
// Declaration of a struct for inventory items
struct InventoryItem {
int itemID;
char itemName[50];
float price;
int quantity;
};
int main() {
// Initialize a struct representing an inventory item
struct InventoryItem laptop = {101, "Laptop", 1200.50, 20};
// Accesse and print inventory item info
printf("Item ID: %d\nItem Name: %s\nPrice: $%.2f\nQuantity: %d\n", laptop.itemID, laptop.itemName, laptop.price, laptop.quantity);
return 0;
}
This code defines a structure (InventoryItem
) to model inventory items. It sets up a variable (laptop
) with specific values for an item, including its ID, name, price, and quantity. The program then accesses and prints the details of the inventory item, such as ID, name, price, and quantity.
Copy the above code and run it in our online C programming compiler.
Student Information System Program
Let’s develop a simple system to manage student info. Make use of the struct
keyword to create a structure representing student details.
#include <stdio.h>
// Declaration of a struct for student info
struct Student {
int studentID;
char studentName[50];
int age;
float GPA;
};
int main() {
// Initialize a struct representing a student
struct Student stud1 = {201, "Soumya", 20, 3.8};
// Access and print student info
printf("Student ID: %d\nName: %s\nAge: %d\nGPA: %.2f\n", stud1.studentID, stud1.studentName, stud1.age, stud1.GPA);
return 0;
}
This code creates a digital student record. It defines a student structure with details like ID, name, age, and GPA. The program creates a student named Soumya, assigns her info, and prints it out. It’s like a simple digital student ID card.
Copy the above code and run it in our online C programming compiler.
C Struct Exercises for Practice
Here are some sample C programs for you to practice the concept of structure in the C programming language.
Exercise#1
Create a structure named Employee
to represent details such as employee ID, name, position, and salary.
Exercise#2
Declare an array of structures to store information about three different products, including their names, prices, and quantities in stock.
Exercise#3
Extend the Car
structure to include information about the color and fuel efficiency of the car.
Exercise#4
Define a structure called Point to represent a point in a 2D space with x and y coordinates. Create two Point variables and find the distance between them using the distance formula.
Exercise#5
Create a structure named Book to store details about a book, including its title, author, publication year, and genre. Initialize an array of Book structures to store information about five different books.
Exercise#6
Enhance the InventoryItem structure to include an expiration date for perishable items. Initialize a structure variable for a perishable item and print its details.
Exercise#7
Design a structure named Rectangle to represent the dimensions of a rectangle (length and width). Write a function that calculates and returns the area of the rectangle.
Exercise#8
Implement a structure called Time to represent the time of day (hours, minutes, seconds). Write a program that takes two Time structures as input and calculates the time difference between them.
Exercise#9
Create a structure named Movie to store information about a movie, such as title, director, release year, and duration. Initialize an array of Movie structures to represent a collection of movies.
Exercise#10
Extend the Student structure to include an array of grades for different subjects. Calculate the average grade for a student and print their overall performance.
Passing Structs to Functions
Functions in C can accept structs as parameters, enabling modular and organized code. This section demonstrates the syntax for passing structs to functions and using them within the function’s scope.
Example:
#include <stdio.h>
struct Rectangle {
int length;
int width;
};
// Function to calculate the area of a rectangle
int calculateArea(struct Rectangle r) {
return r.length * r.width;
}
int main() {
// Initialization of a struct variable
struct Rectangle rect = {10, 5};
// Calling a function with a struct parameter
int area = calculateArea(rect);
printf("Rectangle Area: %d\n", area);
return 0;
}
This example defines a struct Rectangle
and a function calculateArea
that accepts a struct parameter. The program then initializes a rectangle and calculates its area using the function.
Copy the above code and run it in our online C programming compiler.
The Array of Structs in C
Arrays of structs provide a way to manage collections of related data. This section explores the syntax of declaring, initializing, and accessing elements in an array of structs.
Example:
#include <stdio.h>
struct Student {
char name[50];
int age;
};
int main() {
// Declare and initialize an array of structs
struct Student students[3] = {{"Soumya", 20}, {"Harsh", 22}, {"Meenakshi", 21}};
// Accesse elements in the array of structs
printf("Student 1: %s, Age: %d\n", students[0].name, students[0].age);
printf("Student 2: %s, Age: %d\n", students[1].name, students[1].age);
printf("Student 3: %s, Age: %d\n", students[2].name, students[2].age);
return 0;
}
In this example, an array of Student
structs is declared and initialized, and its elements are accessed and printed.
Copy the above code and run it in our online C programming compiler.
Dynamic Memory Allocation for Structs
Dynamically allocating memory for structs is crucial for flexibility in managing resources. This section covers the use of malloc
and free
functions for dynamic memory allocation and deallocation.
Example:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
struct Person {
char name[50];
int age;
};
int main() {
// Dynamic memory allocation for a struct
struct Person *person = (struct Person*)malloc(sizeof(struct Person));
// Assigning values to dynamically allocated struct
strcpy(person->name, "David");
person->age = 25;
// Accessing and printing values
printf("Person: %s, Age: %d\n", person->name, person->age);
// Freeing dynamically allocated memory
free(person);
return 0;
}
In this example, dynamic memory allocation is used to create a Person
struct. After that, it assigns values, accesses, and prints them. Finally, the program frees up the allocated memory.
Copy the above code and run it in our online C programming compiler.
File I/O with Structs
Reading and writing structs to files enables persistent storage for structured
data. This section covers file I/O functions in C and demonstrates their use with structs.
Example:
#include <stdio.h>
struct Book {
char title[100];
char author[50];
int pages;
};
int main() {
// Write struct data to a file
FILE *file = fopen("book_data.txt", "w");
struct Book book = {"The C Programming Language", "K&R", 272};
fwrite(&book, sizeof(struct Book), 1, file);
fclose(file);
// Get the struct data from the file
file = fopen("book_data.txt", "r");
struct Book readBook;
fread(&readBook, sizeof(struct Book), 1, file);
fclose(file);
// Print the data you read from the file
printf("Book Title: %s\nAuthor: %s\nPages: %d\n", readBook.title, readBook.author, readBook.pages);
return 0;
}
This example showcases writing a struct Book
to a file, reading it back, and displaying the retrieved data, illustrating file I/O with structs.
Copy the above code and run it in our online C programming compiler.
Bit Fields in Structs
Bit fields within structs allow for efficient memory utilization, representing and manipulating data at the bit level. This section explores the use of bit fields in structs.
Example:
#include <stdio.h>
// Struct with bit fields
struct Flags {
unsigned int isSet : 1;
unsigned int isEnabled : 1;
unsigned int hasPermission : 1;
};
int main() {
// Initialize struct with bit fields
struct Flags flags = {1, 0, 1};
// Fetch and print bit fields
printf("isSet: %d\n", flags.isSet);
printf("isEnabled: %d\n", flags.isEnabled);
printf("hasPermission: %d\n", flags.hasPermission);
return 0;
}
This example introduces a struct Flags
with bit fields, showcasing their usage in representing binary data.
Copy the above code and run it in our online C programming compiler.
Enum Inside a Struct
Enums within structs provide a way to create more expressive and organized code. This section covers the syntax and advantages of using enums inside structs.
Example:
#include <stdio.h>
// Enum for Days of the Week
enum Days {
MON, TUE, WED, THU, FRI, SAT, SUN
};
// Struct with enum
struct Meeting {
enum Days day;
int startTime;
};
int main() {
// Initialize struct with enum
struct Meeting teamMeeting = {WED, 14};
// Read and display enum inside struct
printf("Meeting Day: %d\n", teamMeeting.day);
printf("Meeting Start Time: %d:00\n", teamMeeting.startTime);
return 0;
}
This example introduces an enum Days
and uses it inside a Meeting
struct. It showcases the combination of enums and structures for improved code organization.
Copy the above code and run it in our online C programming compiler.
Conclusion – Struct in C Programming Language
In conclusion, structures in the C programming language offer a robust mechanism for organizing and managing related data. This guide has provided an in-depth look at the syntax of C structs, covering various scenarios and demonstrating their unique and situational applications. Whether dealing with nested structs, passing structs to functions, working with dynamic memory allocation, or exploring advanced features like bit fields and enums, understanding C structs is essential for creating well-organized and efficient programs.