In this C programming class, we’ll cover C datatypes, their purpose, and their limits. We’ll enhance understanding through the use of flowcharts and code examples for better explanations.
The data types are the core building blocks in C. Hence, please read with full focus and concentration.
Let’s understand C Datatypes from scratch.
What are Data Types in C Programming?
In C programming, data types are exactly what their name suggests. They represent the kind of data that can be stored. They are used to declare functions and variables in a program.
There are three main categories of data types: Basic/Primitive, Derived, and User-Defined.
This diagram would help you.
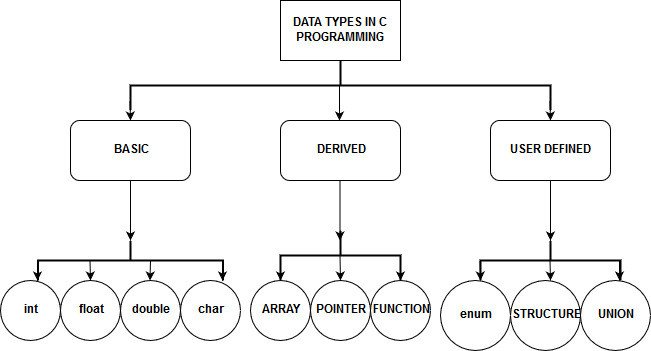
Now, we will see what each of them means individually.
Int
It is used to store the integer data type.
The regular integer that we use has a size of 2 bytes (16 bits) on a 16-bit machine. However, most modern systems have 32 or 64-bit configurations. The size of an integer in such an environment is 4 bytes with a starting value of -2,147,483,648 and an ending value of 2,147,483,647. Any operations involving a number out of this range will return either garbage or an error.
Note: Garbage value is nothing but waste or unused data in a program’s memory. It could also be related to variables that are no longer in use. So, it is always best to initialize to avoid any undefined behavior.
Unsigned int
An integer could also be of an unsigned type. Such a type (unsigned int) on 32-bit will have a range from 0 to 4,294,967,295 and 0 to 65535 on a 16-bit system.
Long
It also stores the integer data with a higher range. The long type is 4 bytes on 32-bit systems and 8 bytes in 64-bit setups.
The 32-bit long range is the same as an integer while on 64-bit, it can hold a minimum of -9,223,372,036,854,775,808 and goes up to 9,223,372,036,854,775,807.
Unsigned long
In some cases, we require only positive numeric values; then it’s better to use the unsigned type. It not only makes sure that you get one but also enhances the range.
The unsigned long is 4 bytes and ranges from 0 to 4,294,967,295.
Float
This data type is also numeric but allows numbers with decimal places. It has a size of 4 bytes and has a range of 3.4E-38 to 3.4E+38.
The float type has a precision of up to 7 digits.
Double
It is the same as float but with a higher precision range that is 8 bytes which gives it the ability to store more numerals after the decimal places, double to be exact.
It has a length of 8 bytes and a range of 1.7E +/- 308. The double data type has a precision of up to 15 digits.
Char
Char data type by default allows a single character such as a letter from the alphabet. It has the size of just 1 byte with a range of -128 to 127 or 0 to 255.
However, with an array [] suffix, it can hold string values, a combination of alphanumeric characters.
Unsigned char
Also, there is an unsigned char type that is similar but doesn’t allow the (-ve) values. It has the same size of 1 byte with a range of 0 to 255.
Short
The length of the short type is two bytes. It allows the range of values equal to 2 to the power 16, i.e., up to 16 bits. Since it is a signed one, hence, contains both the positive and negative values.
It has a range of values between -32768 and 32767.
Unsigned short
The unsigned short type contains only +ve numbers and also has a size of 2 bytes. It can store a minimum value of 0 and goes up to a maximum of 65 535.
Array
A C array is a contiguous sequence of elements of a similar data type. It is primarily to create a collection of the primitives like numbers, chars, struct, and unions.
We have a dedicated chapter for the Arrays which you can go through later.
Pointers
Pointers are used to point and access the values stored at an address in the program’s dynamic memory known as the heap.
The pointer type variables get stored on the heap while the other types are on the stack.
Like an array can have multiple elements, the pointer too can hold several values depending upon the amount of memory it gets allocated.
This data type also has a chapter dedicated to it so we will see more of it later.
Enum
The enum is used to assign a name to a variable to a constant. We will also are this in further chapters.
Structure (struct)
The structure type is used to create a data type that can hold multiple data types in it.
Union
A union is a data type that has all values under it stored at a single address.
In the below C data types example, we’ll see the use of the two fundamental C datatypes.
Data Types Range and Sizes
We’ve captured all the fundamental C datatypes along with their size in bytes and the range of values in the below table.
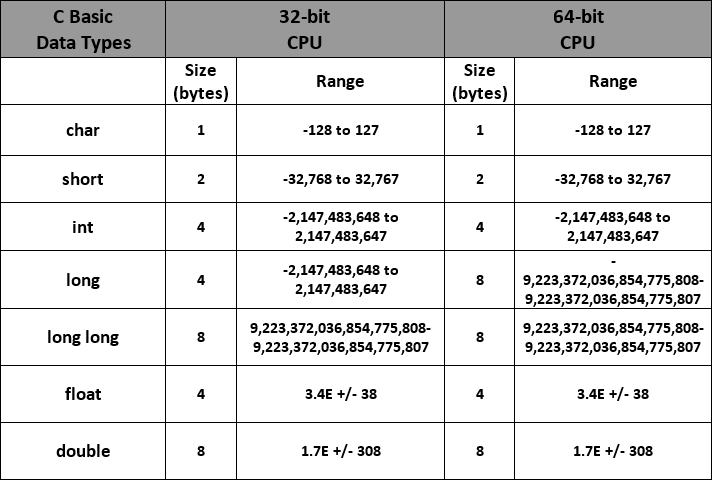
Sample Program: Print Quotient of Two Numbers
In this example, we will perform the arithmetic division operation on two integer numbers. After that, the same result will get assigned to two variables of the following types.
- Integer
- Float
Let’s first understand the flow of this program with the help of its flowchart.
Flowchart:
Algorithm:
Step 1: Start. Step 2: Initialize variables. Step 3: Take input from the user. Step 4: Get the quotient c=d=a/b Step 5: Print output of the integer as well as the float. Step 6: Stop.
Code:
#include<stdio.h> #include<conio.h> void main() { int a, b, c; float d; printf("Enter two numbers to divide : \n"); scanf("%d %d", &a, &b); c = a/b; d = a/b; printf("Quotient of %d and %d in the form of int is %d",a , b, c); printf("\nQuotient of %d and %d in the form of float is %f",a ,b ,d); getch(); }
Note: float data type is declared in printf using %f just as the int is declared using %d.
The output should look something like this.
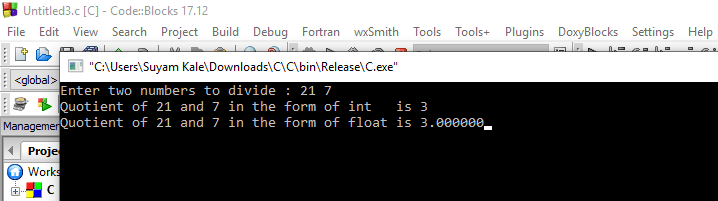
Note: If we use the double type, then it would also give the same output, but it would matter for programs where accuracy is needed.
We have just covered the basics here. You shall learn more about the C data types and their advanced usage in the next C programming tutorials.