In this C programming class, we’ll cover the C for loop statement, its purpose, syntax, flowchart, and examples. Please note that the loops are the main constructs to implement iterative programming in C.
C For Loop for Beginners
In our previous tutorial, we learned the functioning of while and do-while loops. In this chapter, we will see the for loop in detail.
We’ve taken up an entire chapter on the “for loop” because it is the most used iterative programming construct. And the programmers use it in almost every program. Hence, let’s start with its explanation.
Why is the “For Loop” Used in C Programming?
The For Loop is a loop where the program tells the compiler to run a specific code FOR a specified number of times.
This loop allows using three statements, first is the counter initialization, next is the condition to check it and then there is an increment/decrement operation to change the counter variable. You will understand it once we see some programs.
For starters, this flowchart will help you.
C For Loop Flowchart
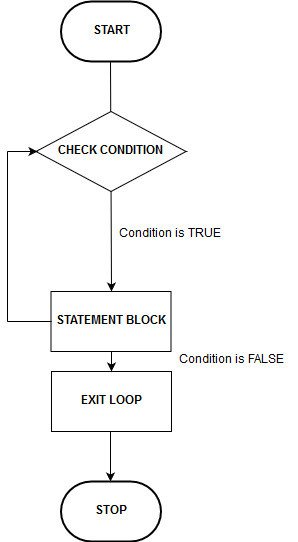
C For Loop Syntax
for( triad statement ) { //statement block }
The for loop’s triad statement has the following structure:
( i=0 ; i < n ; i++ )
First comes the initialization of the counter variable.
For example – If the variable is “i” then it needs to be initialized like the following.
i = 0;
Next, there comes a conditional statement separated by a semicolon.
Example: It can be a specific value, or it can be another variable.
"; i < 0; " or "; i >= a; "
The last is the increment/decrement operation again separated by a semicolon.
Example: It goes like the following.
"; i++" or "; i--"
Finally, the C for loop gets the following structure.
for (i = 0; i <= 10; i++) { //Statement block }
Now we will see some examples of this through some programs.
C For Loop Examples
Program-1: Program to find the factorial of a number
Flowchart:
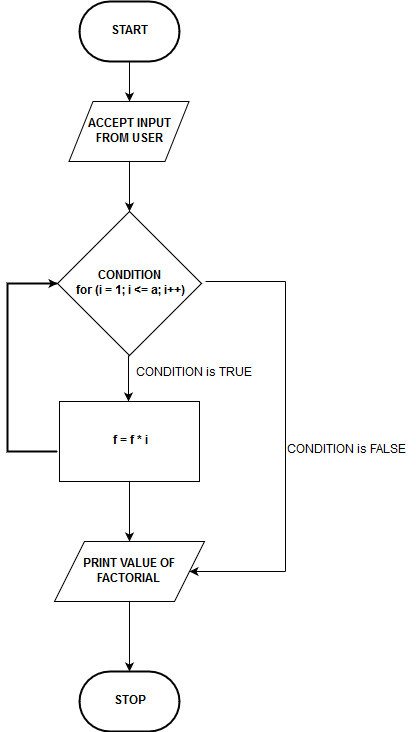
Algorithm:
Step 1: Start. Step 2: Initialize variables. Step 3: Check FOR condition. Step 4: If the condition is true, then go to step 5 otherwise go to step 7. Step 5: f = f * i. Step 6: Go to step 3. Step 7: Print value of factorial. Step 8: Stop.
Code:
#include <stdio.h> #include <conio.h> void main() { int i, a ,f = 1; printf("Enter a number:\n"); scanf("%d", &a); for (i = 1; i <= a; i++) f = f * i ; printf("Factorial of %d is %d\n", a, f); getch(); }
The output of the above example is something like this-
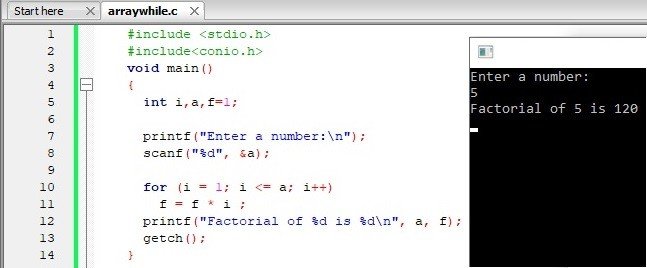
You have two options here. Either you can put {} after for or you cannot. However, if there are multiple statements, then you should put {} so that the code remains organized.
Program-2: Program to find all numbers between 1 to 100 divisible by 4
Flowchart:
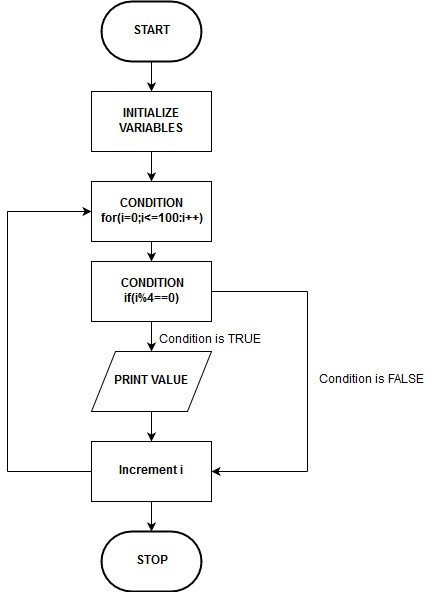
Algorithm:
Step 1: Start Step 2: Initialize variables Step 3: For condition Step 4: If condition. If it is true, then go to step 5 otherwise to step 7 Step 5: Print value Step 6: Increment the value of "i" by 1 Step 7: Go to step 3 Step 8: Stop
Code:
#include<stdio.h> #include<conio.h> void main() { int i; printf("Numbers from 0 to 100 divisible by 4\n"); for(i = 0; i <= 100; i++) { if(i%4 == 0) { printf("%d\t", i); } i++; } getch(); }
Output:

The for loop is very important in C language and will be used very often so you should study this comprehensively. This chapter may have fewer exercises but you’ll find plenty of for loop examples in further tutorials to come.
Happy Coding!