In this C programming class, we’ll cover the C while and do…while loop statements. The loops are the main constructs to implement iterative programming in C.
During your C coding journey, there will be instances when you will need to run a statement more than once. It could be required for taking multiple inputs or processing output. You can achieve this by using loops in C.
We also have a tutorial on “Setting up C Environment” available to help you get started quickly.
Loops in C Programming
A loop is an instruction given to the computer that it has to run a specific part of the code for a given number of times.
To perform a particular task or to run a specific block of code several times, the concept of LOOP comes into the picture.
Basically, there are three types of loops in C language. In this tutorial, we will see the first two loops in detail.
While Loop in C
The while loop begins by first checking the terminal condition. If the condition is true, the control enters the loop else not.
If the underlying condition is true, then it goes ahead and executes the block of code in the loop. After the first iteration, it again checks with the changed (increased/decreased) values of the variables (the condition operands) and decides the further course of execution.
Flowchart
The below flowchart will help you understand the functioning of the while loop.
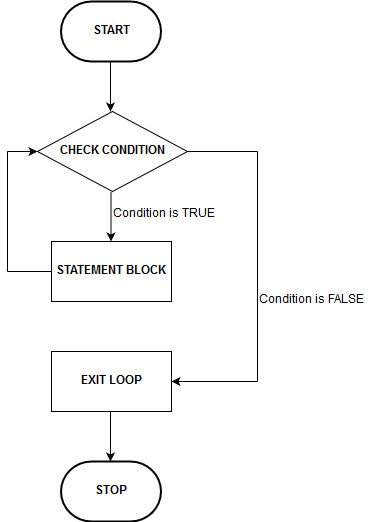
Syntax
While (condition) { //Statement block //Increment/Decrement operation }
While Loop Example
Here is a simple example of how a while loop works. This program prints numbers from 1 to 10 without actually using the ten printf
statements but a while loop.
#include<stdio.h> #include<conio.h> void main() { int n = 1; while(n <= 10) { printf("%d\n\n", n); n++; } getch(); }
Here, the “\n” in the printf call is used to move to the next line.
Its output should look something like this-
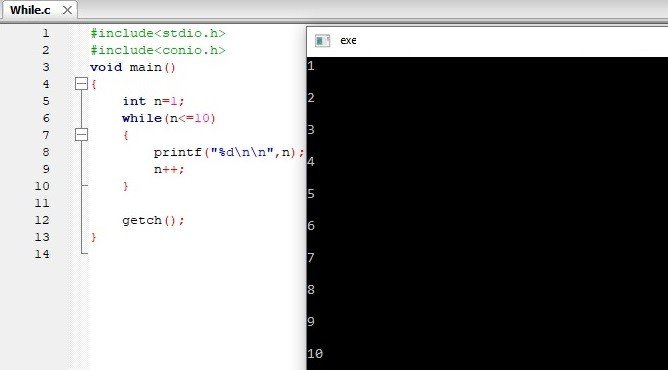
Do…While Loop in C
In this loop, the statement block gets executed first, and then the condition is checked.
If the underlying condition is true, then the control returns to the loop otherwise exit it.
Flowchart
The below flowchart will help you understand the functioning of the do-while loop.
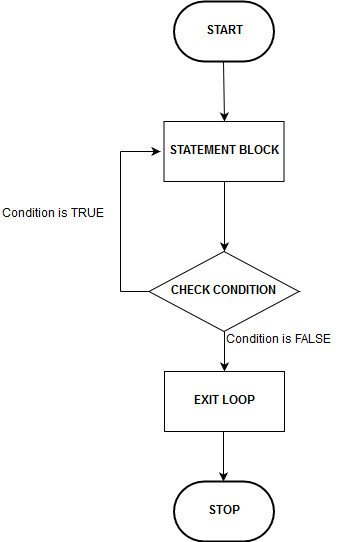
Syntax
do { //statement block } While(condition);
C Do…While Loop Example
Here is a simple example to find the sum of 1 to 10 using the do-while loop
#include<stdio.h> #include<conio.h> void main() { int i = 1,a = 0; do { a = a + i; i++; } while(i <= 10); printf("Sum of 1 to 10 is %d",a); getch(); }
Its output should be something like this-
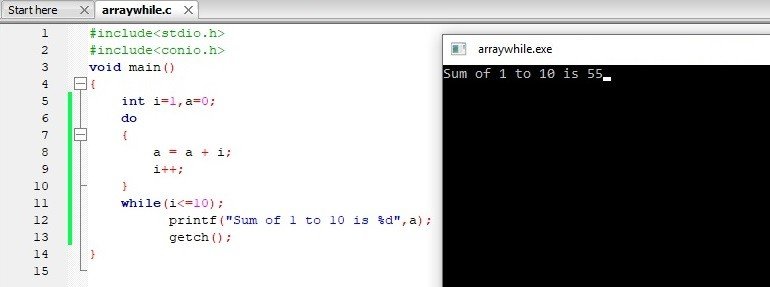
Generally, the do-while loop is not preferred in applications as it first executes the block of statements and then checks the condition. It risks security which is like allowing an unauthorized person into a facility and then asking for his ID.
In the above sections, we gave an explanation of the while and do-while loops. We will see the for loop in detail in the next chapter.
There is an exercise you can perform on the next page which will help you understand these two loops nicely.
C Loops Exercises for Practice
Write a program in C to multiply two numbers without actually using the * operator but have to use both the while and do-while loops.
While loop Sample Program
#include<stdio.h> #include<conio.h> void main() { int a, b, i = 0, c = 0; printf("Enter two numbers to multiply : "); scanf("%d %d",&a, &b); while(i < b) { c = c + a ; i++; } printf("The product of these numbers is %d", c); getch(); }
Do…While Loop Sample Program
#include<stdio.h> #include<conio.h> void main() { int a, b, i = 0, c = 0; printf("Enter two numbers to multiply : "); scanf("%d %d",&a, &b); do { c = c + a ; i++; } while(i < b); printf("The product of these numbers is %d", c); getch(); }
Keep in mind that in a do-while loop, the semicolon always comes after a while statement but not in a while loop.
The output for both the following programs is the same, check from the below screenshot.
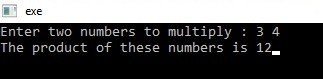