We are today presenting a detailed overview of Java coding guidelines to help programmers and automation testers. Whether you are into Java development or using Java for automation testing, you can use this post to improve your coding style. With the help of these Java coding guidelines, you’ll be able to write code that is robust, readable, rectifiable, and reusable.
- Robust – Error-free and efficient
- Readable – Easily read and understood
- Rectifiable – Properly documented
- Reusable – Capable of being used again
Our objective is to provide a path to consistent practice while coding in Java language. These guidelines apply to all types of software coding activity using the Java language.
Java Coding Guidelines: Your Key to Better Code
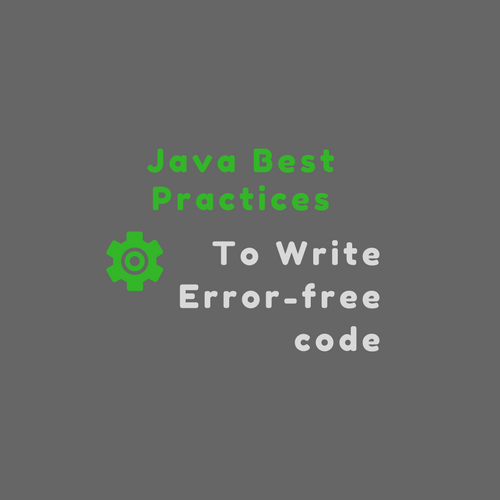
1.1- File Structure for Java Source Files
Let’s first go through what Java coding guidelines say about file management.
1.1.1- File naming guidelines.
Java programs should use the following file suffixes.
File Type | Suffix |
Java Source | .java |
Class Files | .class |
Archive Name | .jar or .zip |
Note: The Java source file name must be the same as the class or interface name contained in that file.
1.1.2- Directory structure
/***************** * Project Catalog: ***************** | | |------------- Docs => for project related document. | | |------------- Source => for all the source files. | | | |-------- packageA => for source files related to packageA. | | | |-------- packageB => for source files related to packageB. | | |------------- Target => for all the class files. | | | |-------- packageA => for class files related to packageA. | | | |-------- packageB => for class files related to packageB. | | ******* * Note: A file should contain only one public class in it. ******* */
1.1.3- File structure
As per Java coding guidelines, the project must include the following sections.
- File Header.
- Package Name.
- Imports.
- Class definition.
1.1.3.1- File Header
Include a file header as provided below.
/*********************************************************************** #File header layout. File Name : Principal Author : Subsystem Name : Module Name : Date of First Release : Author : Description : #Change History. Version : Date(DD/MM/YYYY) : Modified by : Description of change : ***********************************************************************/
1.1.3.2- Package Name
Package names should occur on the first non-commented line of the source file and should follow the naming conventions defined in this document.
1.1.3.3- Imports
Immediately following the package name should be the imported class names.
1.1.3.4- Class definition
Immediately following the imports should be the definition of the class. The organization of the class is described in the next section.
1.2- Class Structure for Java Source Files
A Java class should comprise the following sections.
1- Class Header.
2- Static/Instance variable field declarations.
3- Static initializer.
4- Static member inner class declarations.
5- Method declarations.
6- Instance initializer.
7- Instance constructor declarations.
Note: The class instance, static variables, and methods should fall in public, protected, default, and then private accessibility orders. All public fields should have documentation comments.
1.2.1- Class Header
The class header has to be included as given in the below format.
/** * Java class layout. * @deprecated * @see ClassName * @see ClassName#memberFunctionName * @version text * @author authorName * @since */
1.2.2- Static/Instance variable field declarations
Static variables should come first and begin their declaration with the keyword <static>. Instance variables don’t require to get prefixed with the <static> keyword.
Example
static private int counter, serial; // Incorrect static private int counter; // Correct static private long serial; // Correct
Some of the important points you should note.
- Always get the field declarations in separate lines.
- A field or class which doesn’t change after initialization should be declared final. This approach allows the compiler to generate better code.
- Make sure to align the field names so that they all start in the same column.
- Don’t leave any variable without the access specifiers.
1.2.3- Static initializer
A static initializer, if any, comes next. It must have the following form.
static { statements; }
1.2.4- Static member inner class declarations
The inner classes which are static should come next. Such classes should follow the following structure.
public class Outer { static class Inner { // static inner class } }
Must Read: Learn String Formatting in Java with Examples
1.2.5- Method Declarations
Every Java method should have a linked description in <javadoc> format. Here is a <javadoc> sample to use for public methods.
/** * Description about the Method. * * @param name desc * @exception name desc * @return desc * @see ClassName * @deprecated * @version text * @author authorName * @since */
Standard methods may avoid a description if grouped using any of the following logical groupings.
1- Factory
2- Private
3- Protected
4- Interfaces
5- Accessor
6- Temporal
7- I/O
8- Debugging
Example
/* ======================================================= * Factory Methods (usually static). * ======================================================= */ /** brief summary.*/ public testClass initbject(void){ } /* ======================================================= * Accessor Methods. * ======================================================= */ public int getObjectState(void); public void setObjectState(int value); /* ======================================================= * Standard Methods. * ======================================================= */ // anything it could be. /* ======================================================= * Debugging Methods. * ======================================================= */ void doRunTests(void);
1.2.6- Instance initializer
An instance (non-static) initializer, if any, comes next.
1.2.7- Constructor declarations
Constructor declarations, if any, come next.
Example
public testClass(); public testClass(testClass source);
If there are multiple constructors and some have more parameters, then they should appear after those with fewer parameters. It means that a constructor with no arguments should always be the first one.
1.3- Naming Guidelines
It’s one of the Java coding guidelines which depends on the context you are in. Let’s read more about this.
1.3.1- General Concepts in Naming
1- Follow the domain-related naming strategy.
2- Use sentence cases to make names readable.
3- Be reluctant while using abbreviations.
4- Prevent using redundant names that differ only in case.
1.3.2- Article Naming Convention
1.3.2.1- Arguments or parameters
Use a related name for the value/object being passed, and prefix it with <arg> or <param>.
e.g. argEmpName, paramSalary, etc.
1.3.2.2- Fields and Variables
Start the field/variable name in lowercase and then continue in sentence case.
e.g. clickCheckBox, viewInfo, openWindow.
Don’t use underscores to start or separate the words.
1.3.2.3- Constants
Use upper case and underscores to form constants.
e.g. static final int MAX_SIZE = 256;
static final string BROWSER_TYPE = “Chrome”;
1.3.2.4- Classes and Interface
Always begin class/interface names with a capital letter.
e.g. Class Name: PageFactory or PageObject.
Interface Name: IPageObjectModel
1.3.2.5- Compilation Unit Files
Use the name of the class or interface prefixed with <.java> to represent it as a source code file.
e.g. TestPage.java, UIMap.java, LoginPage.java.
1.3.2.6- Component
Use a meaningful name with a proper suffix.
e.g. categoryMenu, listView, etc.
1.3.2.7- Packages
Start package name with unique top-level domain names like com, edu, gov, etc. Follow the ISO Standards 3166, 1981. The remaining part may vary according to an organization’s internal naming structure.
e.g. com.techbeamers.testpackage.
1.3.2.8- Member Function
Have a function name that relates to the task is meant for. Start it with an active verb whenever possible.
Some good naming practices
Good method names
showStatus(), drawCircle(), addLayoutComponent().
Bad method names
menuButton() – noun phrase; doesn’t describe function.
OpenTable () – starts with an upper-case letter.
click_first_menu() – uses underscores.
Boolean getter member functions
It’s a good practice to prefix boolean getter functions with <is>.
e.g. isVisible(), isChecked(), isNumeric().
Getter member functions
Usually, all getter functions should start with the <get> prefix.
e.g. getLocalDate(), getMonth(), getDayOfMonth().
Setter member functions
Usually, all setter functions should start with the <set> prefix.
e.g. setLocalDate(), setMonth(), setDayOfMonth().
Note: Getter/Setter functions should follow strict guidelines for Java Bean classes.
1.4- Source Code Style Guidelines
1.4.1- Line Spacing
1- Limit each line to under 80 characters.
2- Limit comment length up to 70 characters.
3- Keep tab sizes equal to 4 spaces.
1.4.2- Blank Space
Allow one space between operators and expressions.
average = average + sum; while ( average += sum ) { }
1.4.3- If/else
The if…else statement must adhere to the following format.
if (expression) if (expression) { { statement; statement; } else } elseif(expression) { { statement; statement; } }
1.4.4- For Loop
A for-loop statement must conform to the following format.
for (initialization; condition; update) { statements; }
In the JDK 1.5 release, there is a new feature introduced related to enhanced for loop. In this, the array index is not necessary for the retrieval of an array element.
int numArr[] = { 12, 14, 18, 16, 32 }; for (int n: numArr) { System.out.println("Result: " + n); }
1.4.5- While.
A while loop must adhere to the following format.
while (expression) { statement; }
1.4.6- Do…While Loop
A do-while loop must adhere to the following format.
do { statement; } while (expression);
1.4.7- Switch
A switch statement must adhere to the following format.
switch (expression) { case n: statement; break; case x: statement; // Continue to default case. default: // Always add the default case. statement; break; }
1.4.8- Try/Catch/Finally
The try/catch statement must adhere to the following format.
try { statement; } catch (ExceptionClass e) { statement; }
A try-catch statement may also be followed by finally, which executes regardless of the execution status.
finally { statement; }
1.5- Comments
Here are the Java coding guidelines for quality comments.
1- Use comments before the declarations of interfaces, classes, member functions, and fields. Adopt the Javadoc format for commenting.
2- Apply C-style comments to outline code that is no longer applicable.
3- Limit comments to a single line for member functions, sections of code, and declarations of temporary variables.
4- Write comments to improve the clarity and readability of the code.
5- Don’t add duplicate information while giving comments.
6- Limit the Comment length up to 70 characters per line.
In Java, there are four ways of adding comments.
1.5.1- Block Comments
All data structures and algorithms within the function can be explained through block comments. Block comments should be indented to the same level as the code.
/* * This is an example for Block Comments. * ... * ... */
1.5.2- Trailing Comments
Mostly used to describe the small size code-like conditions. Make sure the comment should be short as well.
if (javaVersion == newVersion) /* Special condition for version */ { // code... }
1.5.3- Single Line comments
Use such comments within the member functions to document logic, sections of code, and declarations of temporary variables. Also, this comment can be used to indicate the end of iterative statements when it is nested.
if (size > 1) { if (revision > 2) { // Sequence of statements } // End of inner if statement } // End of main if statement.
1.5.4- Documentation comments
1- Documentation comments describe Java Classes, Interfaces, Constructors, Methods, and Fields.
2- This type of comment should appear before declarations.
3- Make sure that these comments are not inside a method or constructor block.
4- Documentation comments start with /** and end with */.
5- JavaDoc processes documentation comments.
/** * This Class Contains details about a blog post. * It contains the number of words written and author of the post. * */ Public class BlogPost { int noOfWords; char author[256]; };
1.6- Standard Java Coding Conventions
1.6.1- Declarations
1- Limit one declaration per line for objects and variables.
2- Avoid declaring different types of the same line.
3- Set default values for local variables at the time of declaration.
4- Best to have all declarations at the outset of the block.
5- Don’t use declarations that override other variables having identical names.
6- Make sure to eliminate warnings if there are any.
1.6.2- Statements
1- Write only one statement per line.
2- Don’t initialize more than three variables with a comma inside a “for” loop.
3- Don’t forget to end a switch case with a break statement.
4- Make sure the switch statement must have a default case.
5- Do not hard-wire any number in the code instead, use a Macro to define constants.
6- While comparing always keep the constant on the left-hand side to avoid any unpredictable assignments.
7- While returning from a function, follow the single and single exit approach.
8- Make it a practice to check for null while accessing any object or data structure.
9- Limit the no. of arguments to five for functions and methods.
10- Also, don’t extend the no. of characters from 80 characters per line.
1.6.3- Import Declaration
1- Begin an import statement starting from the first column and use a single space to separate the <import> keyword from the package name.
2- Group all import statements using the package name.
3- Use a blank line to separate groups of import statements.
4- Sort the import statements as per the dictionary order.
5- Prevent from using an open import statement like <import java.io.*;> as it’ll lead to unused imports.
1.6.4- Blank Spaces
1.6.4.1- Adopt blank space Reject tabs
1- Always set a single blank space to use in the editor. Using tabs isn’t wise as the tab size varies editor by editor.
2- Add a single space between a keyword and the opening parenthesis. This applies to keywords like the <catch, for, if, switch, synchronized, and while>. Don’t do this for <super and this>.
if (obj instanceof Post) { // Correct. if (obj instanceof(Post)) { // Incorrect.
3- Add a space after the comma in a list and after the semicolons inside a “for” loop.
int num[5] = {10, 20, 30, 40, 50}; for (expr1; expr2; expr3) { }
1.6.4.2- Avoid using blanks
1- Between a function name and its opening parenthesis.
2- Before or after a “.” (dot) operator.
3- Between a unary operator and its operand.
4- Between a cast and the expression.
5- After an opening parenthesis or before a closing parenthesis.
6- After an opening square bracket “[” or before a closing square bracket “]”.
// Pseudo code. int test = arr1[x + y] + arr2[z]; test = (x + y) / (x * y); if (((a + b) > (y - x)) || (x != (y + 3))) { return dummy.area(x, y); }
7- Do not use special characters like form feeds or backspaces.
1.6.5- Indentation
Line indentation is always 4 spaces for all the indentation levels. You may indent using tabs (which you should avoid) to reduce the file size. However, you shouldn’t alter the hard tab settings to accomplish this. They must be set to eight spaces.
1.6.6- Continuation Lines
Lines should be limited to 80 columns except for non-ASCII encoding. If they go more than 80 chars, then split them into one or more continuation lines. All the continuation lines should be aligned and indented from the first line of the statement. The amount of indentation depends on the type of statement. The same indentation rule you should follow for nested blocks like <try…catch>, <switch…case>, or loops. See the below examples.
// Correct. long_function_name(long_expression1, long_expression2, long_expression3, long_expression4); // Correct - blank line follows continuation line because same indent if (long_logical_test_1 || long_logical_test_2 || long_logical_test_3) { statements; } // Incorrect. while (long_expression1 || long_expression2 || long_expression3) { } // Incorrect. while (long_expression1 || long_expression2 || long_expression3) { }
1.6.7- Member documentation comments
1- All public members must be preceded by a documentation comment.
2- The programmer can choose to add a description for protected and default access members.
3- Private units don’t need a documentation comment.
4- The fields that don’t have a documentation comment should have single-line comments describing them.
1.6.8- Class and instance variable field declarations
1- Variables defined using the keyword static are class variables.
2- Variables defined without the “static” keyword are instance variables.
3- You should declare a class variable first if there is any.
4- Next, you should declare an instance variable after the class variable.
A field declaration looks like the following. Elements in square brackets “[]” are optional.
[FieldModifiers] Type FieldName [= Initializer];
The <FieldModifiers> can be any valid combination of the following keywords, in this order:
public protected private static final transient volatile
Place all the field declarations on separate lines. Don’t club them with each other in a single line.
static private int usageCount, index; // Incorrect static private int usageCount; // Correct static private long index; // Correct
1.7- Exception handling
You must follow the below Java coding guidelines for implementing effective exception handling.
1- Always write a catch block for handling exceptions.
2- Make sure to add a logging message or the stack trace in the catch block.
3- Avoid catching the general exception and have a specific exception.
4- The cleanup code should be added in the <finally> block.
5- This provides a single location for the cleanup, and it’s guaranteed to run.
1.8- Eclipse Plugins for Code Styling
To implement Java coding guidelines, you need several tools. And it’s easy to integrate these with Eclipse IDE. Some of them are listed below.
1- Checkstyle plugin.
2- Spell-checker plug-in tool.
3- Find bugs plugin.
4- Code profiler tool – to detect the violation of the guidelines.
5- TestNG or JUnit for unit testing.
6- ANT/Maven for building the project.
Final Thought – Java Coding Guidelines
We tried to cover the most common Java coding guidelines that we thought could be useful for both Java developers and automation testers. In case, there is something you would like to add/update to this post, please don’t hesitate to share it with us.
Our readers often give their valuable suggestions which we promptly bring through new posts on this blog. Please remember that your interest drives us to deliver better content.
Best,
TechBeamers