From this tutorial, you will be learning to use and run Pylint for Python files. It is a static code analysis tool to find coding errors in your Python code.
Understanding Pylint
Pylint is an essential tool for Python programmers. Initially, it was created to focus on styling checks. However, later it brought more checks and features to detect style and logical issues.
The creator of Pylint namely Sylvain Thénault derived its name from the Unix tool lint. It too analyzes source code for potential errors. Hence, Thénault wanted to create a similar utility specifically for Python.
Note: The syntax used in the below section is for Python 3. You may change it to use a different version of Python.
Find the Best Python IDE for Programming
Check out the 10 best IDEs for Python programming. Choose the one that helps you code faster while making testing and debugging easier.
What is Pylint?
It is a static code analysis tool to identify errors in Python code and helps programmers enforce good coding styles. This tool enables them to debug complex code with less manual work.
It is one of the tools that gets used for test-driven development (TDD). The coding style that Pylint applies to the code is known as PEP8.
For more information, about PEP8 visit the link: PEP8 Style Guide for Python
Other products which are similar to Pylint are Pyflakes, Mypy, etc. It is a must-have tool for every beginner as well as advanced users. It scans and rates programs with a score according to the rules outlined in the PEP8 style guide.
How to install Pylint?
To install it on systems such as Windows 10, Mac OS, and Linux, use the following command:
pip install pylint
You can also use alternative methods such as:
1. On Debian, Kali Linux, and Ubuntu-based systems such as Ubuntu, Elementary, etc.
# Debian, Kali Linux, Ubuntu sudo apt install pylint
2. On Fedora
# Fedora sudo dnf install pylint
3. On OpenSUSE
# OpenSUSE sudo zypper install pylint
or
# OpenSUSE sudo zypper install python3-pylint
You can even integrate Pylint into various IDE (Integrated Development Environment) such as Eclipse, Visual Studio Code, etc. However, here, we will focus on Pylint usage without using IDE integration.
How to use and run?
To run Pylint, use the command line and specify its name as the command. It can be run in many cases like run on a single or multiple files or even a directory.
Run Pylint for a single file
The command to use Pylint on a Python file is:
# Check for style errors pylint filename.py
It returns output consisting of semantic errors, syntax errors, errors in coding style, bugs in the code, excessive and redundant code, etc. It also assigns a score that indicates whether the Python code is an ideal one to use and maintains a history of scores obtained while running over a Python file as well as after each edit.
Run on multiple files
You can type multiple Python files separated by spaces after the Pylint command. It processes each file sequentially, scanning them one by one. So, it will analyze each Python file in the order they are provided.
This is the default Pylint command to run on multiple files in the default sequential way.
pylint task1.py task2.py task3.py
Run on a directory
To run Pylint on a directory, use the following command. It will analyze all the Python files inside the dir and subdirectories.
pylint code_dir/
Analyze files concurrently
Pylint provides a way to scan multiple files in parallel to speed up the process.
You can run Pylint for multiple files concurrently using the following command.
pylint --jobs=4 task1.py task2.py task3.py task4.py
Simultaneously, the same can be done for the files in a directory.
pylint --jobs=4 code_dir/
In both cases, the –jobs option controls the number of parallel jobs Pylint will run for analysis.
Define output format
When you run Pylint, it prints the results in plain text on the console. However, it accepts various output formats. For example, follow the below command.
pylint --output-format=json task1.py
Using .pylintrc config
To use the consistent coding standard across projects or teams, you must use the .pylintrc file. When you run Pylint, it looks for a configuration file named .pylintrc in the current working directory. It reads the settings from it and applies them during the analysis of Python files.
Here is a .pylintrc file incorporating the most common options you should configure.
[MASTER]
# Specify the maximum line length
max-line-length = 100
# Specify the output format (default is text)
output-format = colorized
# Specify additional paths to look for modules
init-hook='import sys; sys.path.append("path/to/your/modules")'
# Set the default score threshold for generating a report (0 to disable)
evaluation = 10.0
[MESSAGES CONTROL]
# Disable certain warnings or errors
disable =
invalid-name, # Disable warnings about invalid variable names
missing-docstring, # Disable warnings about missing docstrings
# Enable additional checks
enable =
unused-import, # Enable warnings about unused imports
unused-variable # Enable warnings about unused variables
[BASIC]
# Enforce PEP 8 style conventions
pep
In the next section, you can check out sample programs demonstrating its usage.
Run Pylint with examples
Here is simple Python code where we ran Pytlint. In the output, you can see the -10 rating and find the suggestions. After addressing the issues, the final code is clean and rated 10/10.
Sample program with style issues
Here is a simple program (sample.py) that has some styling issues.
a = 23 b = 45 c = a + b print(c)
Run pylint
Below is the output after you pass the above sample to Pylint. It lists multiple styling issues in the program.
Check This: The Best 30 Python Questions on Lists, Tuples, and Dictionaries
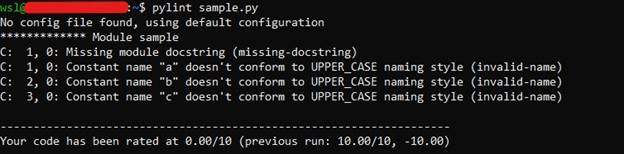
Fix errors and re-run
After fixing the code, the modified version looks like this:
""" Code to add two numbers """ a = 23 b = 45 c = a + b print(c)
The output will come as:

Python Functions Quiz – A Refresher
Do check out this quiz, if you want to test your comfort level with Python functions.
Enjoy Code Cleanup,
TechBeamers