In this Selenium tutorial, you will learn to interact with iFrame elements.
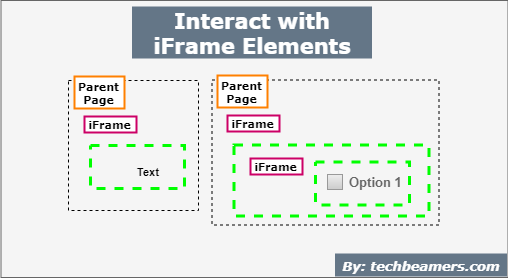
Interacting with iFrame Elements
An iFrame is a unique HTML entity that opens a web page inside another page. Once you get the focus on the iFrame, you might need to work with its elements.
Since it is a page within another page, you need first to select the target iFrame and then access its elements via standard Selenium locator strategies. Let’s try to understand all this with the help of real-time examples.
In the first example, you will find code and explanation to interact with one iFrame and iFrame elements. The second one is addressing when the web page has multiple iFrames.
To learn more check out – Handle iFrame in Selenium
Before you start working with either single or nested iFrames on a web page, you should first search for their presence.
Count no. of iFrames on a web page
Following is the Selenium command to help you locate iFrame:
List<WebElement> web_iframe = driver.findElements(By.tagName("iframe"));
Next, you can count the number of iFrames available on the web page. Check out below the code:
import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class HandlingIframes { public static void main(String[] args) { // set the geckodriver.exe property System.setProperty("webdriver.gecko.driver", "geckodriver.exe"); WebDriver browser = new Firefoxbrowser(); // Open the web page url browser.get("web page url having iframes"); //Searching for iframes List web_iframe = browser.findweb_iframe(By.tagName("iframe")); int iFrameCount = web_iframe.size(); System.out.println("No. of Iframes: " + iFrameCount); browser.quit(); } }
In the above test code, the very first step is to create a Firefox WebDriver object. After object creation, we can refer to it as a browser.
Using this browser object, you can provide the link and open the web page.
After this, we are locating the list of iFrames available on the web page. The following syntax is used:
List web_iframe = browser.findweb_iframe(By.tagName("iframe"));
In this line, we are using the tagName property to find an iFrame tag. After that, we are providing this as an input to the findElements method. It returns a list of iframes as Web elements.
Finally, we used the size() of the List interface and printed the number of iFrames found on the web page onto the console.
Interact with elements from a single iFrame
If the web page has a single iFrame, then we first switch to it. After that, we find the target element and perform the desired operation.
Check out the below code:
public class SingleIFrame { public static void main(String[] args) throws Exception { // Set the geckodriver.exe property System.setProperty("webdriver.gecko.driver", "geckodriver.exe"); // Launch firefox WebDriver browser = new FirefoxDriver(); browser.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Navigate to the webpage browser.get("ulr containing iframes"); // Set the text value String strText = "Some Demo Data"; // Switch to iframe browser.switchTo().frame("my_iframe"); // Assign the text value to target iframe text element browser.findElement(By.xpath("//input[@type='text']")).sendKeys(strText); } }
Interact with elements of Nested iFrames
In some situations, we have multiple and nested iFrames on a web page. If an iFrame has another iFrame, then we call it a nested iFrame. In such a case, we need to switch to iFrame within the iFrame.
The content of a nested iFrame is only available from inside an iFrame. We can’t access elements from outside the iFrame.
In our example, we are checking for a checkbox that is present in a nested iFrame.
Below is the fully working code that you can utilize while working on a nested iFrame assignment.
public class NestediFrame { public static void main(String[] args) throws Exception { // Set the geckodriver.exe property System.setProperty("webdriver.gecko.driver", "geckodriver.exe"); // Launch firefox WebDriver browser = new FirefoxDriver(); browser.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Navigate to the webpage browser.get("ulr containing iframes"); // Find the outer iframe WebElement outer_iframe = browser.findElement(By.id("outer_iframe")); // Switch to outer iframe browser.switchTo().frame(outer_iframe); // Locate the nested iframe WebElement nested_iframe = browser.findElement(By.xpath("//iframe[@id='nested_iframe']")); // Switch to nested iframe browser.switchTo().frame(nested_iframe); // Locate the checkbox and select the option WebElement option = browser.findElement(By.xpath("//input[@type='checkbox']")); // if option is not selected then click the checkbox if( !option.isSelected() ){ option.click(); } } }
Wait for an iFrame to load
Sometimes you face a problem when switching to a specific iFrame fails because it hasn’t loaded. You can address such a case using the following webdriver-backed selenium code:
WebDriverWait waitX = new WebDriverWait(browser, 15); waitX.until(ExpectedConditions.frameToBeAvailableAndSwitchToIt(By.Id("target_frame"));
The above statement ensures that you get to the desired iFrame without any error. Simultaneously, you should be checking while accessing elements inside an iFrame.
WebDriverWait waitY = new WebDriverWait(browser, 15); waitY.until(ExpectedConditions.ElementIsVisible(By.Id("target_element"));