In this tutorial, you’ll get to see how to handle IFrame in Selenium Webdriver. You can learn from the Java sample code called Selenium APIs to communicate with the IFrame element.
Introduction
An IFrame is a container that can load a web page inside another page. It is also known as inline frames. On a web page, the iframe HTML tag represents the IFrame.
The iframe tag has an src attribute along with height and width to specify the URL of another page to load. It serves many purposes, such as loading an advert, dynamic content, etc. Please note that an IFrame can also have frames inside frames.
To learn Selenium from scratch – Selenium Webdriver Tutorial
Handling IFrames in Selenium Webdriver
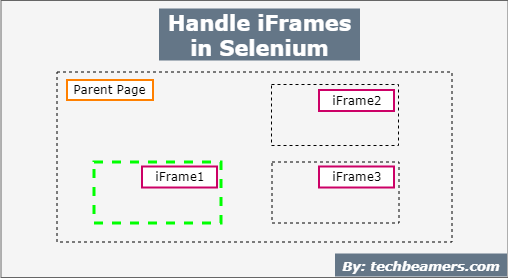
Selenium provides multiple functions to work with iFrames. We can choose different ways to handle IFrame relating to our needs. Please check out the following ways:
Selenium methods to handle IFrames
Mainly, Selenium provides the driver.switchTo().frame() to interact with an IFrame. However, it comes with different variations based on the argument types.
Index to manage IFrame
It selects a frame based on the index value. If the web page has more than one frame, then the first frame appears at the zeroth index, the second at 1, and so on.
After the frame is set, the WebDriver sends all calls to that frame. The driver’s focus moves to the selected frame. In such a situation, any operation on the parent page will fail and throw elements not found as we switched to one of the inner frames.
This function has the following specifications.
/*** Function: driver.switchTo().frame(int arg0) Parameter: Index (starting from 0) Return value: currently selected frame Exception value: NoSuchFrameException (The frame isn't available) ***/
See the below example using the switchTo() function to select a frame based on its id.
public void switchToIFrameByIndex(int frame_index) { try { driver.switchTo().frame(frame_index); System.out.println("Navigated to frame with index: " + frame_index); } catch (NoSuchFrameException ex) { System.out.println("Unable to locate frame with index: " + frame_index + ex.getStackTrace()); } catch (Exception ex) { System.out.println("Unable to navigate to frame with index: " + frame_index + ex.getStackTrace()); } }
Name or ID to handle IFrame
Another form of the switchTo() function allows a string to pass the frame name. It locates the frames by comparing their name attributes and gives precedence to those matched by ID.
/*** Function: driver.switchTo().frame(String arg0) Parameters: Name Or ID - Name of the frame or the ID attribute of the frame tag. Return value: The driver sets the focus to the given frame Exception value: NoSuchFrameException - If the desired frame is not available. ***/
We’ve provided a sample code snippet demonstrating the frame name usage.
public void switchToIFrameByName(String frame_name) { try { driver.switchTo().frame(frame_name); System.out.println("Navigated to frame having name " + frame_name); } catch (NoSuchFrameException ex) { System.out.println("Unable to find a frame having ID " + frame_name + ex.getStackTrace()); } catch (Exception ex) { System.out.println("Unable to locate a frame having ID " + frame_name + ex.getStackTrace()); } }
WebElement to locate IFrame
This function gets us to an IFrame based on the previous value of its WebElement.
/*** Function: driver.switchTo().frame(WebElement iframe) Parameters: iframe - The target frame element. Return value: The driver sets the focus to the given frame Exception value: NoSuchFrameException - If the target frame is not available. StaleElementReferenceException - If the WebElement is stale. ***/
Find out the below example which passes a WebElement and performs the switch.
public void switchToIFrameByWebElement(WebElement web_iframe) { try { if (isElementPresent(web_iframe)) { driver.switchTo().frame(web_iframe); System.out.println("Navigated to iframe matching web element "+ web_iframe); } else { System.out.println("Unable to navigate to the desired iframe "+ web_iframe); } } catch (NoSuchFrameException ex) { System.out.println("Unable to find a iframe matching web element " + web_iframe + ex.getStackTrace()); } catch (StaleElementReferenceException ex) { System.out.println("Web Element with " + web_iframe + "is not bind to the page document" + ex.getStackTrace()); } catch (Exception ex) { System.out.println("Unable to navigate to iframe matching web element " + web_iframe + ex.getStackTrace()); } }
Navigate to the Parent of an IFrame
You might need to switch to the parent page of an IFrame. It is the main page which has all the child IFrames inside. After you finish working with the IFrames, then you would want to switch back to the parent page.
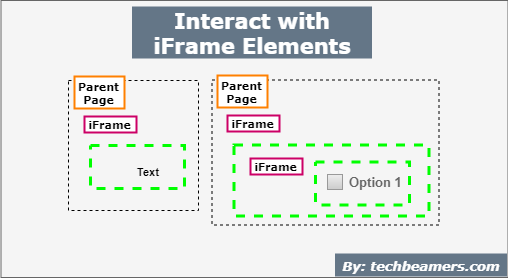
For this purpose, you can call Webdriver’s switchTo().defaultContent() method. It will take you to the main page.
// Navgiate to your test URL page driver.get(""); // Let's find the iframe using a locator strategy WebElement web_iframe = driver.findElement(By.id("sample_iframe")); // Next, perform the switch by index driver.switchTo().frame(0); // Finish your tasks in iframe 0 // Finally, get back to the parent page driver.switchTo().defaultContent(); // Quit from the webdriver driver.quit();
Now, you have learned to handle iFrame and know how to switch between iFrames. The next topic is how you interact with different elements embedded in an iframe.
We’ll cover the iFrame and its element interaction in the next tutorial. Check out – How to Interact with Elements in iFrames