In this tutorial, we will explore three methods to generate all subarrays of an array in Python. Subarrays are contiguous subsets of elements within an array. Let’s find out what these three ways are and how they generate all subarrays of an array in Python. They include using nested loops, list comprehension, and recursive approach.
3 Unique Python Methods to Generate All Subarrays of an Array
Generating all subarrays of an array is one of the basic coding approaches. It allows us to explore different combinations of elements within the array. This technique proves valuable in solving various problems. For instance, it can help identify the largest sum within a group of numbers in the array. It’s also useful for finding the longest common sequence between two strings.
Generating all subarrays of an array was first used to solve the maximum subarray problem. This problem involves finding the largest sum of adjacent numbers in the array. To solve it, you generate all subarrays in the array and then find the one with the highest sum.
Must Read: How to Create Arrays in Python with Examples
What is a subarray?
A subarray of an array is a sequence of contiguous elements from the original array. For example, if the original array is [3, 7, 11, 17]
, then some of its subarrays are [3, 7]
, [11, 17]
, and [
.3, 7, 11, 17
]
Why is it useful to generate all subarrays of an array?
Generating all subarrays of an array can be useful for a variety of tasks, such as:
- Find the maximum or minimum subarray sum
- Get the longest subarray of consecutive elements
- Search the subarray with the most frequent element
- Solving dynamic programming problems
Let’s now begin exploring these three methods to generate all subarrays of an array in Python. The very first method we are going to start with is using the nested loops.
Method 1: Using Nested Loops
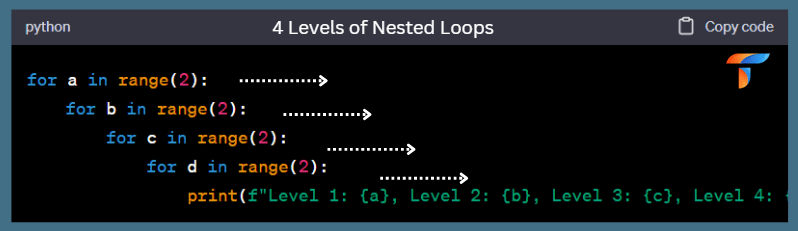
The simplest way to generate all subarrays of an array is by using nested loops. We will use two loops to iterate through the start and end positions of the subarray. Here’s how you can do it:
Python code:
def subarrays(arr):
subs = []
n = len(arr)
for p in range(n):
for q in range(p, n):
sub = arr[p:q + 1]
subs.append(sub)
return subs
num1 = [11, 22, 33]
str1 = ["apple", "banana", "cherry"]
num_subs1 = subarrays(num1)
str_subs1 = subarrays(str1)
print("Using Nested Loops:")
print("Number Subarrays:")
for sub in num_subs1:
print(sub)
print("String Subarrays:")
for sub in str_subs1:
print(sub)
Time Complexity:
The time complexity of the nested loops approach is O(n^3), where ‘n’ is the length of the input array. This is because there are two nested loops to consider all possible start and end positions. Each combination creates a new subarray by slicing the original array.
Space Complexity:
The space complexity of the nested loops approach is O(n^3) as well. This is because it creates a new list for every subarray. In the worst case, there can be n^2 subarrays in the result.
Method 2: Using List Comprehension
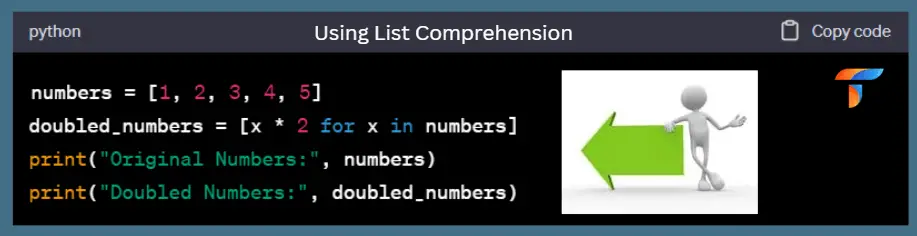
We can achieve the same result more concisely using list comprehensions:
Python code:
def subarrays(arr):
return [arr[p:q + 1] for p in range(len(arr)) for q in range(p, len(arr))]
num2 = [99, 88, 77]
str2 = ["dog", "cat", "elephant"]
num_subs2 = subarrays(num2)
str_subs2 = subarrays(str2)
print("\nUsing List Comprehension:")
print("Number Subarrays:")
for sub in num_subs2:
print(sub)
print("String Subarrays:")
for sub in str_subs2:
print(sub)
Time Complexity:
The time complexity of the list comprehension approach is O(n^3) for the same reasons as the nested loops method, as it essentially performs the same operations.
Space Complexity:
The space complexity for the list comprehension approach is O(n^3) because it creates all subarrays in a single list, similar to the nested loops approach.
Method 3: Using Recursion
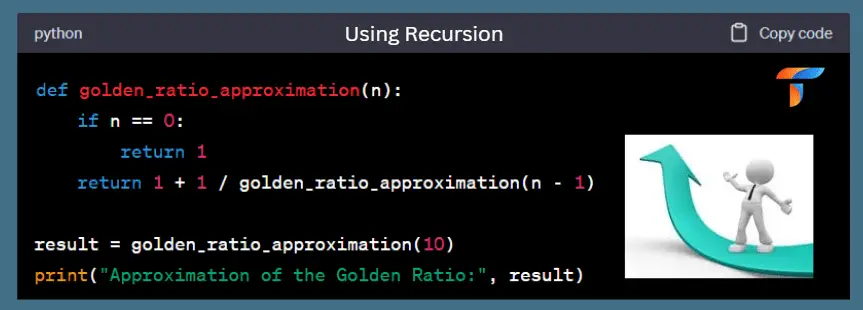
A recursive approach can also be used to generate all subarrays. We will define a recursive function to find subarrays starting from each element in the array:
Python code:
def subarr(arr, p, q, subs):
if p == len(arr):
return
elif q == len(arr):
subarr(arr, p + 1, p + 1, subs)
else:
sub = arr[p:q + 1]
subs.append(sub)
subarr(arr, p, q + 1, subs)
def subarrs(arr):
subs = []
subarr(arr, 0, 0, subs)
return subs
num3 = [5, 10, 15]
str3 = ["apple", "banana", "cherry"]
num_subs3 = subarrs(num3)
str_subs3 = subarrs(str3)
print("\nUsing Recursion:")
print("Number Subarrays:")
for sub in num_subs3:
print(sub)
print("String Subarrays:")
for sub in str_subs3:
print(sub)
Time Complexity:
The recursive approach has a worst-case time complexity of O(n^2). This happens as it inspects subarrays starting from each element. For each starting point, it explores the end positions only once. In terms of time complexity, it’s more efficient than both the nested loops and list comprehension methods.
Space Complexity:
The space complexity of the recursive approach is O(n^2). This occurs because it produces subarrays for each starting point. In the worst case, there can be n^2 subarrays in the result.
Comparing Different Ways to Generate Subarrays of an Array
- The recursive approach is more efficient in terms of time complexity. It is also better in space complexity compared to the nested loops and list comprehension.
- The nested loops and list comprehension methods share the same time and space complexity. However, they are less efficient compared to the recursive approach.
- While the recursive approach is more efficient, it may not be suitable for very large arrays due to potential stack overflow issues. In such cases, an iterative solution using nested loops or list comprehension would be a better choice.
In summary, if you have the choice between these methods and performance is a concern, the recursive approach is the most efficient in terms of time and space complexity for generating all subarrays. However, the choice of method may also depend on readability and specific requirements in your programming task.
Happy coding!