Python integers are not just basic arithmetic types like integers or real numbers in C, they are objects with an internal data structure for storage. This is why Python’s integer size is different from other languages.
In fact, Python integers are variable-length, meaning they can grow as large as the available memory allows. This allows for precision and accuracy when dealing with large numbers.
But why is the size of Python integers larger than those in C? Let’s dive deeper into this topic to understand the inner workings of Python integers.
Why is Python Integer Size Different from Integers in C?
Whenever Python finds an assignment like <x=1>, it tries to construct an integer object. In Python, an integer object is seeded using the following data structure.
PyIntObject
typedef struct { PyObject_HEAD long ob_ival; } PyIntObject;

The <PyObject_HEAD> is a macro that expands to a reference counter of type <Py_ssize_t> and a pointer of <PyTypeObject> type.
Py_ssize_t ob_refcnt; PyTypeObject *ob_type;
Next, Python reserves a pre-allocated block of Integer objects. And use it to serve new integer requests instead of making allocations on every assignment. Here is the block structure used to hold integer objects. But it’ll still not suffice the need for large integer size in Python.
PyIntBlock
struct _intblock { struct _intblock *next; PyIntObject objects[N_INTOBJECTS]; }; typedef struct _intblock PyIntBlock;
You can verify from the above structure that it can hold up to 40 PyIntObject objects on a 64-bit system. It’s the maximum of integer objects that can fit in a block of 1K bytes.
#define N_INTOBJECTS ((BLOCK_SIZE - BHEAD_SIZE) / sizeof(PyIntObject))
Once a block’s integer object pool gets depleted, a new block becomes available to fulfill the new requests for integer objects. All these blocks are chained together in the form of a single linked list.
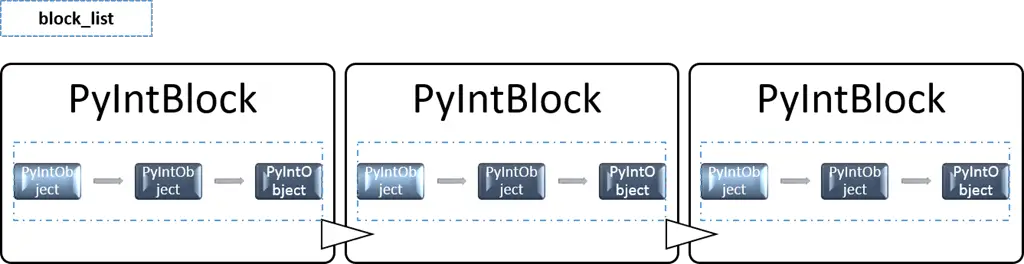
We should also mention that Python handles small integers in a little different fashion than big integers. It uses a type array of 262 pointers to store the small integers falling in the range from -5 to 256. That’s the primary reason Python has bigger integer sizes.
static PyIntObject *small_ints[NSMALLNEGINTS + NSMALLPOSINTS];
It means the small integers won’t get served from the object pool. Instead, they will use the list of pointers given above. Hence, the small integers will end up using the same PyIntObject integer object but with a different reference count.
On the contrary, the big integers get their allocation from the integer object pool. And each of them would have its own <PyIntObject> type object.
Another interesting fact that you should know is the difference between the lifetime of small and big integers. Unlike a big integer, small integers stay in memory as long as the Python interpreter is running. Observe this fact from the example given below.
small_int1=-5 => None small_int2=-5 => None small_int1 is small_int2 => True big_int1=257 => None big_int2=257 => None big_int1 is big_int2 => False
The above tests would have given you a fair idea of why integer sizes in Python are bigger than ints in C. Let’s conclude this topic now.
Summary – Python Using a Bigger Integer Size
Till now, you’ve learned that Python integers are entirely different from the integer type available in C. And more importantly, you would now know the answer to your question- “Why is Python Integer Size Different from Integers in C?”.
We hope you enjoyed this post and have a clear idea of the integer object pool, its structure, and its application in Python.
But that’s not all! Our website offers a range of additional resources to help you build your skillset.
- Generate a List of Random Integers in Python
- Python Program to Find Sum of Two Numbers
- Python Program to Generate Random Integer Numbers
- Master Python List Comprehension in 2 Minutes
- Core Java Quiz on Strings
Best,
TechBeamers.