Hello Dear Readers, today we are going to tell you about the JNA (Java Native Access) concept used in Java applications. In this tutorial, you will create a JNA program to call the “C” library functions using the JNA library from Java code.
You can apply the JNA concept in various applications like a test automation framework where you can use JNA to integrate your C code with the automation framework.
if you are creating web browser extensions where you need to interact with the file system, then you can use JNA to call system Apis to meet your requirements.
In this JNA tutorial, we’ll give you a basic idea of JNA via a code sample. You can directly reuse the code of this JNA example in your project.
JNA Tutorial – Write a Simple JNA Program in Java
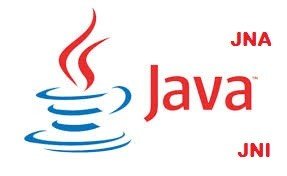
What is JNA (Java Native Access)?
JNA is a platform-independent technology for invoking Native C APIs from the Java code. It supports multiple platforms and the following C library types.
1- DLL (Dynamic Link Library) on Windows platforms.
2- SO (Shared Object) on Linux platforms.
There is an alternative method known as JNI which can also load a C/C++ DLL. It’s also quite popular but requires writing additional Java wrapper code to handle data types and methods of the underlying C/C++ library.
We’ll cover the JNI method at length sometime later. For now, let’s focus on learning the JNA concept and its application.
JNA, if you compare it with JNI is far easier to use and implement. It will step up your coding speed if you are working on a Java project making use of C Libraries.
Create a Sample JNA Program
You will need to perform the following steps to set up a JNA project.
Download JNA Jars
The first step for you is to download and import JNA (Java Native Access).
After that, you need to create a Java project and import the JNA jar files.
Create a Java Class to Load C Library
Next, create a Java class file that loads the C library as mentioned below-
It is the interface file that contains the definition of the JNA functions defined in the “C” library.
package JNAApiInterface; import com.sun.jna.Library; public interface JNAApiInterface extends Library { JNAApiInterface INSTANCE = (JNAApiInterface) Native.loadLibrary((Platform.isWindows() ? "msvcrt" : "c"), JNAApiInterface.class); void printf(String format, Object... args); int sprintf(byte[] buffer, String format, Object... args); int scanf(String format, Object... args); }
Create another Java Class to Call C API
The second Java file is the implementation file which imports the Interface class and calls its API for validation.
package JNABucket; import JNAApiInterface; import com.sun.jna.Native; public class JNABucket { public static void main(String args[]) { JNAApiInterface jnaLib = JNAApiInterface.INSTANCE; jnaLib.printf("Hello World"); String testName = null; for (int i = 0; i < args.length; i++) { jnaLib.printf("\nArgument %d : %s", i, args[i]); } jnaLib.printf("\Please Enter Your Name:\n"); jnaLib.scanf("%s", testName); jnaLib.printf("\nYour name is %s", testName); } }
Also Read: Java Collection Interview Questions
Footnote – JNA Program Tutorial
We hope that this JNA tutorial will give you the desired solution to your problem. If it did, then please leave us your feedback in the comment section.
Your input will encourage our team to deliver better content every time we decide to post.
In the end, if you find this JNA tutorial useful, then don’t miss to share this post with your friends and on the social media of your choice.
Best,
TechBeamers