In this post, we’ll learn how to handle date time picker controls like jQuery date picker and Kendo date picker using Selenium Webdriver.
Many large portals from the travel and hospitality domain commonly use date-time picker calendars for scheduling hotel and flight bookings. It is important for this control to work properly so that the end user won’t face any trouble while booking his trip.
Hence, it is important for the UI testers to get acquainted with such web elements. They should at least know about the standard controls that sites use frequently.
It will help them in selecting the right strategy to handle controls like the date time picker calendar.
Today, we’ll cover this topic in detail and will provide a sample code for a deeper understanding. Following is the list of main points that we’ll explain in this tutorial.
- What is a date-time picker control?
- What are the different types of date time picker controls?
- How to automate the date time picker calendar in Selenium?
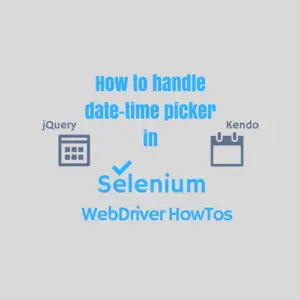
Introduction to date-time picker control
A date-time picker (aka DTP) control provides a simple and user-friendly interface for exchanging date and time information with a user. For example, you can use a DTP control to ask the user to input a date and then quickly fetch the selection. Most of the time, it appears as an input field in an HTML form. When the user selects a date, the feedback gets shown as the input’s value.
Different types of date time picker controls
Usually, there are following two types of date picker controls used in web applications that you may need to handle in Webdriver.
- jQuery DatePicker.
- Kendo UI DatePicker.
jQuery date picker is a part of the open source project at jQuery.org whereas the Kendo date picker is the proprietary work by Telerik.com. Though both these widgets offer a common functionality. Here are some of the main differences.
- jQuery control doesn’t have a validation of its own while the latter has it.
- Kendo date picker is mobile compatible while the jQuery isn’t.
- jQuery requires IE8+ while Kendo is good to work with IE7+.
Automating the date time picker calendar
The main challenge with the date picker control which most testers face is when it shows a calendar that includes dates either from the previous or the next month. There can be many versions of the same problem, e.g. when dates from both the previous and next months appear on the calendar.
Hence, we’ll provide you with code to handle the three types of date-time picker calendars in Selenium Webdriver.
1- Date time picker to show dates from multiple months
Since handling multidate calendars is a bit trickier, we’ll elaborate on the main steps before throwing in the full sample code.
First, you need to open the date time picker demo website then, get the date picker object using the ‘Id’ and click on it.
@BeforeTest public void startTest() { // Launch the demo site to handle date time picker. browser = new FirefoxDriver(); browser.manage().window().maximize(); browser.navigate().to(demosite); } @Test public void jQueryCalendarMultipleMonths() throws InterruptedException { // Start the date time picker demo website. browser.navigate().to(demosite); // click to open the date time picker calendar. WebElement calElement = browser.findElement(By.id("datepicker")); calElement.click(); // Provide the day of the month to select the date. SelectDayFromMultiDateCalendar("3"); }
Now, add a method to handle the date-time picker and its calendar which shows similar date values from different months.
Here is the code to play with the multi-date calendar.
// Function to select the day of month in the date picker. public void SelectDayFromMultiDateCalendar(String day) throws InterruptedException { // We are using a special XPath style to select the day of current // month. // It will ignore the previous or next month day and pick the correct // one. By calendarXpath = By .xpath("//td[not(contains(@class,'ui-datepicker-other-month'))]/a[text()='" + day + "']"); browser.findElement(calendarXpath).click(); // Intentional pause for 2 seconds. Thread.sleep(2000); }
In the method given above, we’ve selected the date of the current month with the help of a special XPath style. In the above XPath expression, we’ve tried to exclude the “other month” element which holds the next month’s dates.
By calendarXpath = By.xpath("//td[not(contains(@class,'ui-datepicker-other-month'))]/a[text()='" + day + "']");
If we enter a date value as 2 then, the above expression will evaluate as the one given below.
//td[not(contains(@class,'ui-datepicker-other-month'))]/a[text()='2']
The multidate handler method would select the “2nd May” (or whatever value you gave) in the calendar and ignore the same date value of the other month.
Sample code to select multiple dates
Now let’s collect all the above pieces of code together, and see the full working code below.
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.support.ui.WebDriverWait; import org.testng.annotations.AfterTest; import org.testng.annotations.BeforeTest; import org.testng.annotations.Test; public class MultiDateDateTimePicker { private String demosite = "http://jqueryui.com/resources/demos/datepicker/other-months.html"; WebDriver browser; WebDriverWait wait; @BeforeTest public void startTest() { // Launch the demo site to handle date time picker. browser = new FirefoxDriver(); browser.manage().window().maximize(); browser.navigate().to(demosite); } @Test public void jQueryCalendarMultipleMonths() throws InterruptedException { // Start the date time picker demo website. browser.navigate().to(demosite); // click to open the date time picker calendar. WebElement calElement = browser.findElement(By.id("datepicker")); calElement.click(); // Provide the day of the month to select the date. SelectDayFromMultiDateCalendar("3"); } // Function to select the day of month in the date picker. public void SelectDayFromMultiDateCalendar(String day) throws InterruptedException { // We are using a special XPath style to select the day of current // month. // It will ignore the previous or next month day and pick the correct // one. By calendarXpath = By .xpath("//td[not(contains(@class,'ui-datepicker-other-month'))]/a[text()='" + day + "']"); browser.findElement(calendarXpath).click(); // Intentional pause for 2 seconds. Thread.sleep(2000); } @AfterTest public void endTest() { browser.quit(); } }
You can test the above code by creating a project in Eclipse and adding the above file. If you want to learn it from scratch, then read tutorials from the Selenium Webdriver Tutorials section on our blog.
2- Handle jQuery date time picker in an IFRAME
jQuery data picker is quite popular among large numbers of websites that use it to improve their user experience. It’s also very common when you need to handle the date time picker in an IFRAME.
So, we are taking on this problem in the below example. Here, the date picker appears in an IFRAME, so you need to switch to the frame first before hitting any Webdriver command. After that, you click on the date picker to open the calendar. Then, you loop through the array of date values to spot the expected date and click to select it.
At the end of the test, there is a little trick given which sets the -ve Y-coordinates and scrolls the page in the upward direction.
Sample code to automate inside an IFRAME
import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; import org.testng.annotations.AfterTest; import org.testng.annotations.BeforeTest; import org.testng.annotations.Test; public class jQueryDateTimePicker { private String demosite = "http://jqueryui.com/datepicker/"; WebDriver browser; WebDriverWait wait; @BeforeTest public void startTest() { // Launch the demo site to handle date time picker. browser = new FirefoxDriver(); browser.manage().window().maximize(); wait = new WebDriverWait(browser, 5); } @Test public void SelectDateFromJQueryCalendar() throws InterruptedException { // Start the date time picker demo website. browser.navigate().to(demosite); // click to open the date time picker calendar. WebElement frameElement = browser.findElement(By .className("demo-frame")); browser.switchTo().frame(frameElement); By dtp = By.xpath(".//*[@id='datepicker']"); wait.until(ExpectedConditions.presenceOfElementLocated(dtp)); browser.findElement(dtp).click(); // Provide the day of the month to select the date. HandleJQueryDateTimePicker("22"); } // Function to select the day of the month in the date picker. public void HandleJQueryDateTimePicker(String day) throws InterruptedException { wait.until(ExpectedConditions.presenceOfElementLocated(By .id("ui-datepicker-div"))); WebElement table = browser.findElement(By .className("ui-datepicker-calendar")); System.out.println("JQuery Calendar Dates"); List<WebElement> tableRows = table.findElements(By.xpath("//tr")); for (WebElement row : tableRows) { List<WebElement> cells = row.findElements(By.xpath("td")); for (WebElement cell : cells) { if (cell.getText().equals(day)) { browser.findElement(By.linkText(day)).click(); } } } // Switch back to the default screen again and scroll up by using // the negative y-coordinates. browser.switchTo().defaultContent(); ((JavascriptExecutor) browser).executeScript("scroll(0, -250);"); // Intentional pause for 2 seconds. Thread.sleep(2000); } @AfterTest public void endTest() { browser.quit(); } }
3- Handle Kendo date time picker
Kendo is a stylish date time picker and provides a much simpler interface than the jQuery control. So it was also simple to write the code for handling the Kendo date picker.
Here, the difference is in the choice of the locators. Instead of XPath, we are using the <cssSelector> and the <className> locators to access the date time picker control. Now, go through the below Selenium Webdriver example and try it out yourself.
Sample code to automate the Kendo date time picker
import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; import org.testng.annotations.AfterTest; import org.testng.annotations.BeforeTest; import org.testng.annotations.Test; public class KendoDateTimePicker { private String demosite = "http://demos.telerik.com/kendo-ui/datetimepicker/index"; WebDriver browser; WebDriverWait wait; @BeforeTest public void startTest() { // Launch the demo site to handle date time picker. browser = new FirefoxDriver(); browser.manage().window().maximize(); wait = new WebDriverWait(browser, 5); } @Test public void SelectDateFromKendoCalendar() throws InterruptedException { // Start the date time picker demo website. browser.navigate().to(demosite); // click to open the date time picker calendar. WebElement kendodtp = browser.findElement(By .cssSelector(".k-icon.k-i-calendar")); kendodtp.click(); // Provide the day of the month to select the date. HandleKendoDateTimePicker("11"); } // Function to select the day of the month in the date time picker. public void HandleKendoDateTimePicker(String day) throws InterruptedException { wait.until(ExpectedConditions.presenceOfElementLocated(By .className("k-content"))); WebElement table = browser.findElement(By.className("k-content")); System.out.println("Kendo Calendar"); List<WebElement> tableRows = table.findElements(By.xpath("//tr")); for (WebElement row : tableRows) { List<WebElement> cells = row.findElements(By.xpath("td")); for (WebElement cell : cells) { if (cell.getText().equals(day)) { browser.findElement(By.linkText(day)).click(); } } } // Intentional pause for 2 seconds. Thread.sleep(2000); } @AfterTest public void endTest() { browser.quit(); } }
Summary
From this post, you might now know that the calendar controls come in various forms and structure a little differently from each other.
Also, with the above examples and sample code, we expect that you should be able to handle date picker controls in your automation projects.
However, if you come across any issue or need help with a new type of calendar control, then do write to us. We’ll try to respond at the earliest.
Keep Learning,
TechBeamers.