We’ve already posted many useful Selenium Webdriver tutorials covering various topics like the Selenium components, working with locators, Firefox add-ons for finding unique locators, setting up Webdriver projects in Eclipse, and much more. Apart from Selenium, we’ve exclusively talked about the TestNG framework and its usage with full-fledged examples. In today’s post, we’ll list down some of the necessary Selenium Webdriver commands that you can directly use in your projects.
We’ve divided the Selenium Webdriver commands into logical sections so that you can identify and prioritize the ones you want to learn first and leave those that you would consider later. The point here is that you need to know most of them as they will help in making big test automation projects. So as a starter, you can refer to the ones that you’ll currently use and come back to finish reading the remaining commands.
Here is the list of sections grouping the Webdriver commands.
Selenium Webdriver Commands Essential for Test Automation.
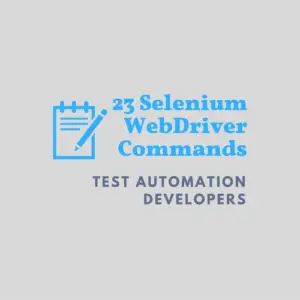
1- Browser Commands.
1.1- Command to Create a Webdriver Object.
Before you write any code to use the Selenium Webdriver commands, you must know how to create a Webdriver instance.
Please refer to the below snippet for creating the Webdriver instance so that you can call any of its functions.
// Creating Firefox driver object. WebDriver driver = new FirefoxDriver(); // Creating Chrome driver object. System.setProperty("webdriver.chrome.driver","C:\\<ChromeDriverPath>\\chromedriver.exe"); WebDriver driver = new ChromeDriver(); // Creating IE driver object. System.setProperty("webdriver.ie.driver","C:\\<IEDriverPath>\\IEDriverServer.exe"); WebDriver driver = new InternetExplorerDriver();
1.2- Close Command.
void close() – This method kills the current window which the WebDriver has control over. It doesn’t allow any parameter and its return type is void.
Command - driver.close();
It’ll terminate the browser if it’s the only window currently open.
driver.close();
1.3- Quit Command.
void quit() – This method Closes all windows opened by the WebDriver. It doesn’t allow any parameter and its return type is void.
Command – driver.quit();
It’ll automatically close all of the opened windows and terminate the browser.
driver.quit();
2- Get Commands.
These are some of the key Selenium Webdriver commands that you’ll frequently need to use in your projects.
2.1- Get command.
void get(String arg0) – This method launches a new web page in the browser window. It allows String as a parameter and returns a void i.e. nothing.
Command - driver.get(siteUrl);
Where siteUrl is the website address to launch. It is the best practice to pass a fully qualified address.
driver.get(""); //Or you can use the following style. String strURL= ""; driver.get(strURL);
2.2- Get Title Command.
String getTitle() – This method returns the title string of the current page in the browser. It doesn’t allow any parameter and its return type is a String.
Command - driver.getTitle();
Since it returns a String value, it’s appropriate to save it in a String object/variable.
driver.getTitle(); //Or write in the following style. String strTitle = driver.getTitle();
2.3- Get Current URL Command.
String getCurrentUrl() – This method returns the string containing the URL currently opened in the browser window. It doesn’t allow any parameter and its return type is a String. It’s one of the rarely used Selenium Webdriver commands, but sometimes it is helpful to use for logging purposes.
Command – driver.getCurrentTitle();
Since it returns a String value, it’s appropriate to store it in a String object/variable.
driver.getCurrentUrl(); //Or use the following style. String strUrl = driver.getCurrentUrl();
2.4- Get Page Source Command.
String getPageSource() – This method retrieves the Source Code of a web page. It doesn’t allow any parameter and its return value is a String.
Command - driver.getPageSource();
Since it returns a String value, you must save it to a String object/variable.
driver.getPageSource(); //Or adpat the below style. String strSource = driver.getPageSource();
3- Navigation Commands.
These Selenium Webdriver commands are very useful and allow you to control the navigation flows in the web browser.
3.1- Navigate To Command.
void to(String arg0) – This method launches a new web page in the browser window. It allows to pass a String parameter, and its return type is a void.
Command - driver.navigate().to(siteUrl);
It is almost similar to the driver.get(siteUrl) method. Here siteUrl is the website URL to open. It is the best practice to use a fully qualified string address.
driver.navigate().to("");
3.2- Forward Command.
void forward() – This method simulates the browser’s forward button action. It doesn’t allow any parameter and its return type is void.
Command - driver.navigate().forward();
It moves you forward by a single page into the browser’s history data.
driver.navigate().forward();
3.3- Back Command.
void back() – This method simulates the browser’s back button action. It doesn’t allow any parameter and its return type is void.
Command - driver.navigate().back();
It moves you back by a single page into the browser’s history data.
driver.navigate().back();
3.4- Refresh Command.
void refresh() – This method simulates the browser’s refresh button action. It doesn’t allow any parameter and its return type is void.
Command - driver.navigate().refresh();
It triggers the same action as does the F5 shortcut in the browser.
driver.navigate().refresh();
4- WebElement Commands.
First, we need to understand what is a web element.
It is the smallest possible HTML entity that helps to form a fully functional web page. In Webdriver terminology, it is a Java class that represents an object that holds the reference to an HTML element.
e.g. <br/> is a Web element or an HTML element. Let’s consider a more complex example.
<div id=’employee’>…</div>
In the above HTML code, the whole div including the opening and closing tags forms an HTML element. Now let’s see the Selenium Webdriver commands associated with the web elements.
4.1- Clear Command.
void clear( ) – This method will clear the value of any text type element. It doesn’t allow any parameter and its return type is also void.
Command – element.clear();
This method doesn’t impact any HTML element other than the field of text type. Example – INPUT and TEXTAREA elements.
WebElement user = driver.findElement(By.id("User")); user.clear(); //Or use the following code. driver.findElement(By.id("User")).clear();
4.2- SendKeys Command.
void sendKeys(CharSequence… keysToSend ) – This method types in the values passed in the argument into the associated web element object. It allows a CharSequence as a parameter, and its return type is void.
Command – element.sendKeys(“enter text”);
This method supports elements like INPUT and TEXTAREA elements.
WebElement user = driver.findElement(By.id("User")); user.sendKeys("TechBeamers"); //Or follow the below coding style. driver.findElement(By.id("User")).sendKeys("TechBeamers");
4.3- Click Command.
void click( ) – This method performs the click action at the web element. It doesn’t accept any parameter, and its return type is void.
Command – element.click();
It is one of the most common methods which interacts with web elements like links, checkboxes, buttons, etc.
WebElement link = driver.findElement(By.linkText("TechBeamers")); link.click(); //Or try to use the below code. driver.findElement(By.linkText("TechBeamers")).click();
4.4- IsDisplayed Command.
boolean isDisplayed( ) – This method checks the visibility of a web element. It doesn’t allow any parameters but its return type is a boolean value.
Command - element.isDisplayed();
This method will return true if the element is present and visible on the page. It’ll throw a NoSuchElementFound exception if the element is not available. If it is available but kept hidden, then the method will return false.
WebElement user = driver.findElement(By.id("User")); boolean is_displayed = user.isDisplayed(); //Or write the code in the below style. boolean is_displayed = driver.findElement(By.id("User")).isDisplayed();
4.5- IsEnabled Command.
boolean isEnabled( ) – This method returns true/false depending on the state of the element (Enabled or not).
Command – element.isEnabled();
This method will usually return true for all items except those which are intentionally disabled.
WebElement user = driver.findElement(By.id("User")); boolean is_enabled = user.isEnabled(); //Or write the code in the below style. boolean is_enabled = driver.findElement(By.id("User")).isEnabled(); //Or choose the following way to write code. WebElement user = driver.findElement(By.id("User")); boolean is_enabled = element.isEnabled(); // Test if the text input field is enabled. If true then enter the value. if(is_enabled){ user.sendKeys("TechBeamers"); }
4.6- IsSelected Command.
boolean isSelected( ) – This method tests if an element is active or selected. It doesn’t allow any parameter, but it does return a boolean status.
Command – element.isSelected();
This method is only applicable to input elements like Checkboxes, Radio Button, and Select Options. It’ll return true when it finds the element is currently selected or checked.
WebElement lang = driver.findElement(By.id("Language")); boolean is_selected = lang.isSelected(); //Or you can re-write the code as below. boolean is_selected = driver.findElement(By.id("Language")).isSelected();
4.7- Submit Command.
void submit( ) – This method initiates the submission of an HTML form. It doesn’t allow any parameter and its return type is void.
Command – element.submit();
If the method is leading the current page to change, then it would wait until the new page gets loaded.
WebElement submit_form = driver.findElement(By.id("Submit")); submit_form.submit(); //Or write the code in the below style. driver.findElement(By.id("Submit")).submit();
4.8 – GetText Command.
String getText( ) – This method will give you the innerText value of the element. You must note that the innerText property is CSS aware and the getText() method would return a blank if the text is hidden.
Command – element.getText();
This method provides the inner text of the associated element. The output includes the sub-elements and leaves any leading or trailing whitespace aside.
WebElement link = driver.findElement(By.xpath("MyLink")); String strlink = link.getText();
4.9- getTagName Command.
String getTagName( ) – This method provides the tag name of the associated element. It doesn’t allow to pass any parameter and returns a String object.
Command – element.getTagName();
Don’t confuse this method to return the attribute name. It’ll return the tag name i.e. “input“ of the element. e.g. <input name=”Password”/>.
WebElement pass = driver.findElement(By.id("Password")); String strTag = pass.getTagName(); //Or style the code as below. String strTag = driver.findElement(By.id("Password")).getTagName();
4.10- getCssValue Command.
String getCssValue( String propertyName) – This method provides the value of the CSS property belonging to the given element.
Command – element.getCssValue(“font-size”);
This method may return an unpredictable value in a cross-browser setup. Like, you would have set the “background-color” property to “green” but the method could return “#008000” as its value.
WebElement name = driver.findElement(By.id("Name")); String strAlign = name.getCssValue("text-align");
4.11- getSize Command.
Dimension getSize( ) – This method returns the height and width of the given element. It doesn’t allow to pass any parameter, but its return type is the Dimension object.
Command – element.getSize();
This method provides the size of the element on the web page.
WebElement user = driver.findElement(By.id("User")); Dimension dim = user.getSize(); System.out.println(“Height # ” + dim.height + ”Width # "+ dim.width);
4.12- getLocation Command.
Point getLocation( ) – This method locates the location of the element on the page. It doesn’t allow to pass any parameter, but its return type is the Point object.
Command – element.getLocation();
This method provides the Point class object. You can easily read the X and Y coordinates of a specific element.
WebElement name = driver.findElement(By.id("Name")); Point point = name.getLocation(); String strLine = System.getProperty("line.separator"); System.out.println("X cordinate# " + point.x + strLine + "Y cordinate# " + point.y);
Final Word – Selenium Webdriver Commands
We hope the above blog post will be helpful for you. And you should be able to use these Selenium Webdriver commands in your ongoing projects.
If need any technical help on the functions explained above, please do let us know.
Just to update that in our next posts, we’ll address the following set of Selenium webdriver commands.
- FindElement VS. FindElements Commands.
- CheckBox and Radio Button Commands.
- Dropdown and Multi-Select Commands.
All the Best,
TechBeamers.