Whenever you plan to automate any web application using Webdriver, you usually start by finding the HTML elements on the page. Then, you choose the right strategy to locate them. Webdriver defines two methods for identifying the elements; they are findElement and findElements.
How to Use FindElement and FindElements
We have given many examples of the above Webdriver commands in our previous blog posts. Now, you’ll see a detailed overview of both these methods.
Next, we’ll also help you explore the different locator strategies that you can use along with these methods. Here is the list of topics we are covering today.
1- Find locators using Firefox’s Inspector and FirePath Tool.
Let’s first see an animated GIF to demonstrate Firefox’s Inspector and FirePath Tool, and then we’ll review the key differences between the findElement and findElements methods.
We’ve captured a small set of actions to showcase how can you use Firefox’s internal inspector tool to find a unique locator. Then there is the FilePath add-on demo to determine or verify the XPath of any element.
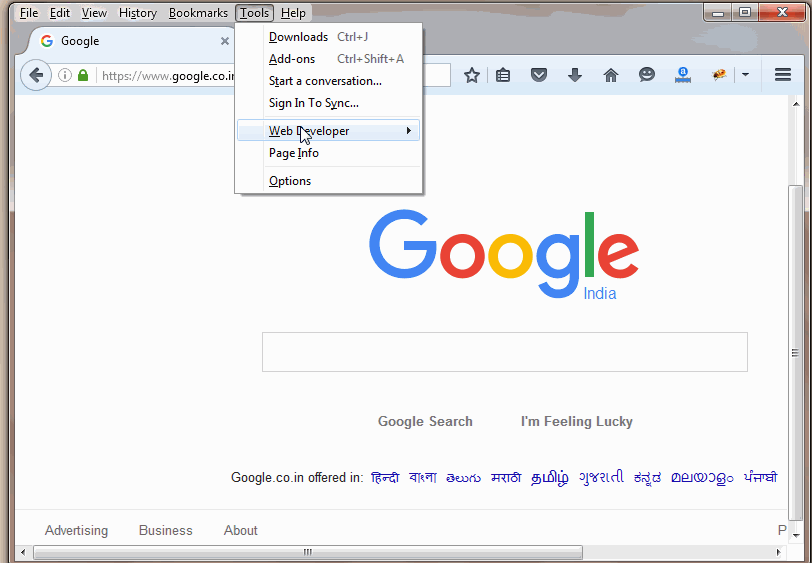
2- The difference between findElement and findElements methods.
findElement() method.
- You can use this command to access any single element on the web page.
- It returns the object of the first matching element of the specified locator.
- It throws a NoSuchElementException exception when it fails to find the element.
- Its syntax is as follows.
WebElement user = driver.findElement(By.id("User"));
findElements() method.
- First of all, we don’t use it frequently as its usage is very limited.
- It gives back the whole list of all the elements matching the specified locator.
- If the element doesn’t exist or is not available on the page then, the return value will be an empty list.
- Its syntax is as follows.
List<WebElement> linklist = driver.findElements(By.xpath("//table/tr"));
Code Example
Now let us provide a straight and practical example of these methods to give you a clear picture.
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; public class findElementTest { public static void main(String[] args) throws Exception { // Start browser WebDriver driver = new FirefoxDriver(); // Maximize the window driver.manage().window().maximize(); // Navigate to the URL driver.get("https://techbeamers.com"); // Sleep for 5 seconds Thread.sleep(5000); // Here, see how the XPath will select unique Element. WebElement link = driver .findElement(By.xpath("'//table/tr[1]/td[1]/a")); // click on the link link.click(); } }
import java.util.Iterator; import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; public class findElementsTest { public static void main(String[] args) throws Exception { // Launch browser WebDriver driver = new FirefoxDriver(); // Maximize window driver.manage().window().maximize(); // Navigate to the URL driver.get("https://techbeamers.com"); // Sleep for 5 seconds Thread.sleep(5000); // Here, the code below will select all rows matching the given XPath. List<WebElement> rows = driver.findElements(By.xpath("//table/tr")); // print the total number of elements System.out.println("Total selected rows are " + rows.size()); // Now using Iterator we will iterate all elements Iterator<WebElement> iter = rows.iterator(); // this will check whether list has some element or not while (iter.hasNext()) { // Iterate one by one WebElement item = iter.next(); // get the text String label = item.getText(); // print the text System.out.println("Row label is " + label); } } }
3- Understand multiple ‘By’ strategies to access locators
Webdriver references the Web elements by using the findElement(By.<locator()>) method. The findElement method uses a locator/query object known as <“By”>. There are various kinds of “By” strategies that you can utilize depending on your requirements. Let’s go through them one by one.
3.1- By ID
Command: By.id(<element ID>)
According to this strategy, the By method will return the first element matching the id attribute value. If it doesn’t find any matching element then, it’ll raise a NoSuchElementException. Using an element ID is the most preferred way to locate an element, as usually, IDs have unique values.
However, in a large project that is in the development phase, the developers may use non-unique IDs or auto-generating IDs. Ideally, they should avoid the occurrence of any of these conditions.
Example:
<input id="TechBeamers">
// Java example code to find the input element by id. WebElement user = driver.findElement(By.id("TechBeamers"));
3.2- By Name
Command: By.name(<element-name>)
By.Name() is another useful way to locate an element but it is also prone to same the issue as we’d seen with the ID. The issue was what if the web developer could’ve used non-unique/auto-generated names on a page.
This strategy states that the <By.Name()> method will return the first element matching the name attribute. If it’s not able to locate it then, it’ll throw a NoSuchElementException.
Example:
<input name="TechBeamers">
// Java example code to find the input element by name. WebElement user = driver.findElement(By.name("TechBeamers"));
3.3- By Class Name
Command: By.className(<element-class>)
This method gives you the element which matches the values specified in the “class” attribute. If the element has more than one class, then this method will match against each one of them.
Example:
<input class="TechBeamers">
// Java example code to find the input element by className. WebElement user = driver.findElement(By.className("TechBeamers"));
3.4- By TagName
Command: By.tagName(<html tag name>)
You can use this method to find the elements matching the specified tag name. You may call this method when you want to extract the content within a tag or wish to perform any action on the tag element.
Example:
<form action="viewuser.asp" method="get" id="userform"> Name: <input type="text" name="name"><br> Age: <input type="text" name="age"><br> </form> <button type="submit" form="userform" value="Submit">Submit</button>
WebElement form = driver.findElement(By.tagName("button")); // You can now perform any action on the form button. form.submit();
3.5- By LinkText or PartialLinkText
Commands: By.linkText(<link text>) and
By.partialLinkText(<link text>)
Using this method, you can track the elements of “a” tags (Link) with the link or partial-link names. Apply this method, when you have the link text that appears within the anchor tag.
Example:
<a href="#test1">TechBeamers-1</a> <a href="#test2">TechBeamers-2</a>
// Java example code to find element matching the link or partial link text. WebElement link = driver.findElement(By.linkText("TechBeamers-1")); assertEquals("#test1", link.getAttribute("href")); // Or WebElement link = driver.findElement(By.partialLinkText("TechBeamers-2")); assertEquals("#test2", link.getAttribute("href"));
3.6- By CSS selector
Command: By.cssSelector(<css-selector>)
With this method, you can use a CSS selector to locate the element. The CSS is a cascading style sheet; it sets the styles for a web page and its elements.
Example:
<input class="email" id="email" type="text" placeholder="your@email.com"> <input class="btn btn-small" type="submit" value="Subscribe to our blog">
// Java code example for using cssSelector. WebElement emailText = driver.findElement(By.cssSelector("input#email")); //Or WebElement emailText = driver.findElement(By.cssSelector("input.email")); WebElement subscribe = driver.findElement(By.cssSelector("input[type='submit'][value='Subscribe to our blog']"));
Apart from the above examples, you can also perform partial matching on HTML attributes.
1) ^= as in input[id^='Tech'] means Starting with the given string. 2) $= as in input[id$='_Beamers'] means Ending with the given text. 3) *= as in Input[id*='techbeamers'] means Containing the given value.
3.7- By XPath
Command: By.xpath(<xpath>)
You can try this method when you want to locate an element using the XPath query. XPath is a way to traverse through the document object model and gives you the ability to select specific elements, attributes, or a section of an XML document.
Example:
// Java code example for XPath. // Absolute path. WebElement item = driver.findElement(By.xpath("html/head/body/table/tr/td")); // Relative path. WebElement item = driver.findElement(By.xpath("//input")); // Finding elements using indexes. WebElement item = driver.findElement(By.xpath("//input[5]")); // Finding elements using attributes values. WebElement item = driver.findElement(By.xpath("img[@alt='banner']")); // XPath starts-with() example. // => input[starts-with(@id,'User')] // XPath ends-with() example. // => input[ends-with(@id,'_name')] // XPath contains() example. // => input[contains(@id,'email')]
The Usage of findElement and findElements
We came out with this post because we wanted you to know the nitty-gritty of the findElement and findElements methods. Another concept we addressed was to choose between the different By.locator() strategies. You can master these concepts only by practicing with real-time web applications. Here is a post where you’ll see a lot of demo websites to practice Webdriver commands.
We expect you to apply this knowledge while you work and create better automation solutions.
Best,
TechBeamers.