From this tutorial, you will learn about the Python Shutil module. It facilitates operations on files which makes it one of the essential modules to learn. Read on to know more.
Note: The syntax used in the below section is for Python 3. You may change it to use a different version of Python.
Python Shutil Module
Must Read – 9 Ways to Copy a File in Python
What is the Shutil module?
The shutil in Python is a module that offers several functions to deal with operations on files and their collections. It provides the ability to copy and remove files.
In a way, it is similar to the OS Module; however, the OS Module does have functions dealing with collections of files.
How does the Python Shutil module work?
The syntax to use the shutil module is as follows:
import shutil shutil.submodule_name(arguments)
Some examples of its functions are copytree(), make_archive(), which(), rmtree(), copy(), copy2(), copystat(), move(), etc.
In the next section, you will learn to use the few common functions frequently used in everyday Python programming.
Program Examples
which() to show the directory of an exe
Here is a simple program to demonstrate which() method.
import shutil shutil.which("python") shutil.which("python3") shutil.which("ls")
The output will come as:
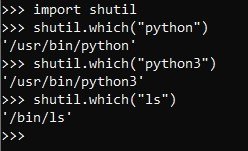
copystat() on a file
Check another program to use the copystat() function.
import shutil shutil.copystat("file_path", "path_to_file_where_you_want_to_copy")
The output will come as:

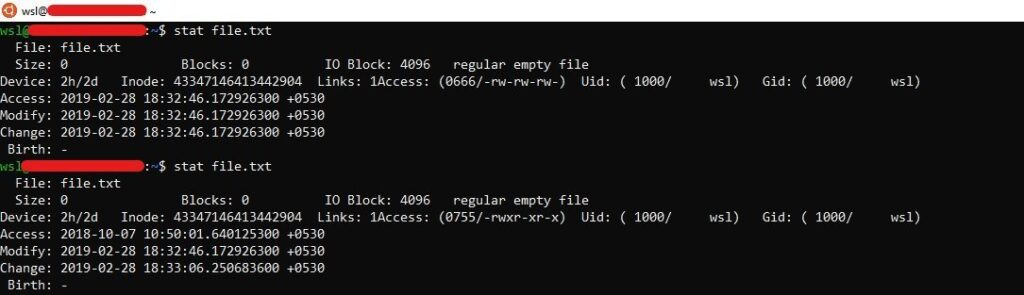
move() to move a file
Below is the program to use move() to relocate a file from one location to another.
import shutil shutil.move("file_path", "path_to_where_you_want_to_move_file")
The output will come as:

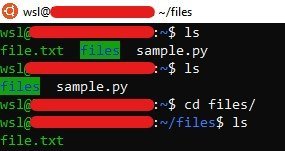
copy() to copy a file
Here is the program to copy a file from one location to another using copy().
import shutil shutil.copy("file_path", "path_to_directory_where_you_want_to_copy_to")
The output will come as:

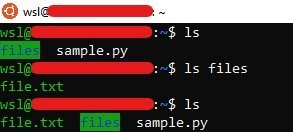
rmtree() to delete files from dirs and subdirs
This function removes all the contents of a directory recursively.
import shutil shutil.rmtree("dir_path")
The output will come as:
import shutil targetdir = '/tmp/reports/'; # Clear a directory using rmtree() try: shutil.rmtree(targetdir) except: print("Operation failed!")
Summary
Here are some key usages and important points of the shutil module in Python, summarized in point form:
- The shutil module offers high-level file operations, such as copying, moving, deleting, and creating files and directories.
- It is a part of the Python standard library, so you do not need to install any additional modules to use it.
- It is a powerful tool for automating file management tasks.
- It should be used with caution, as it can delete files and directories without warning.
Here are some additional important points to keep in mind:
- The shutil module does not support all file operations. For example, it cannot rename files in Python or change their permissions.
- The shutil module is not thread-safe. This means that you should not use it in a multithreaded application.
- The shutil module can be used to perform dangerous operations, such as deleting files and directories. You should use it with caution.
I hope this helps! Let me know if you have any other questions.
Best,
TechBeamers