From this tutorial, you will be learning about the differences between Shallow Copy and Deep Copy (Shallow Copy vs. Deep Copy) in Python.
Note: The syntax used in the below section is for Python 3. You may change it to use a different version of Python.
Shallow Copy vs. Deep Copy
Must Read – 9 Ways to Copy a File in Python
The difference between Shallow and Deep copy
A shallow copy is one that makes a new object store the reference of another object. While, in deep copy, a new object stores the copy of all references of another object making it another list separate from the original one.
Thus, when you make a change to the deep copy of a list, the old list doesn’t get affected and vice-versa. But shallow copying causes changes in both the new as well as in the old list.
This copy method is applicable in compound objects such as a list containing another list.
The diagram shown below represents the difference between the shallow copy and the deep copy.

How to perform a shallow and deep copy?
When creating a shallow copy, use the assignment operator(=) to create them.
With the Copy module, you can create a shallow copy using the below syntax:
import copy copy.copy(object_name)
For a deep copy, the following code can be used:
import copy copy.deepcopy(object_name)
In the next section, a few programs are implemented to demonstrate the Copy Module in Python 3.
Program Example
Here is a simple program to demonstrate the difference between Shallow and Deep copy.
import copy a = [ [1, 2, 3], [4, 5, 6] ] b = copy.copy(a) c = [ [7, 8, 9], [10, 11, 12] ] d = copy.deepcopy(c) print(a) print(b) a[1][2] = 23 b[0][0] = 98 print(a) print(b) print("\n") print(c) print(d) a[1][2] = 23 b[0][0] = 98 print(c) print(d)
The output will come as:
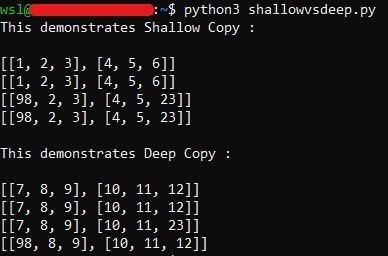
Key Differences
Here is a table summarizing the key differences between shallow copy and deep copy in Python:
Feature | Shallow Copy | Deep Copy |
---|---|---|
What it does | Creates a new object that references the same objects as the original object. | Creates a new object that contains copies of all the objects referenced by the original object. |
When to use it | When you only need to copy the top-level structure of an object. | When you need to copy the entire object hierarchy, including nested objects. |
Speed | Faster | Slower |
Memory usage | Less memory-intensive | More memory-intensive |
Availability | Available in the standard library | Requires the copy module |
Quick Summary
Here is a simple explanation of each feature:
- What it does: A shallow copy creates a new object that references the same objects as the original object. This means that changes made to the original object will also be reflected in the copy. For example, if you have a list of numbers and you shallow copy it, any changes you make to the list in the copy will also be made to the original list.
- When to use it: You should use shallow copy when you only need to copy the top-level structure of an object. This is usually the case when you are copying simple objects, such as lists or strings.
- Speed: Shallow copy is faster than deep copy because it does not need to create copies of all the objects referenced by the original object.
- Memory usage: Shallow copy is less memory-intensive than deep copy because it does not need to create copies of all the objects referenced by the original object.
- Availability: A shallow copy is available in the standard library, so you do not need to install any additional modules to use it. Deep copy requires the copy module, which is a standard library module.
We hope this helps! Let us know if you have any other questions.
Best,
TechBeamers