In this post, we are describing different ways to merge dictionaries in Python. Some of the methods need a few lines of code to merge while some can combine dictionaries in a single expression.
How to Merge Dictionaries in Python
There is no built-in method to combine dictionaries, but we can make some arrangements to do that. The few options that we’ll use are the dictionary’s update method and Python 3.5’s dictionary unpacking operator also known as **kwargs.
You need to be decisive in selecting which of the solutions suits your condition the best. So, let’s now step up to see the different options to solve our problem.
A Must Read: Python Dictionary | Create, Update, Append
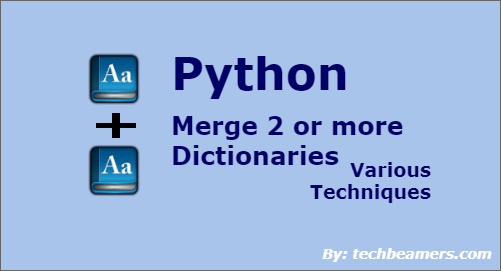
Before we jump on the solutions, let’s define our problem statement first.
Different Ways to Merge Two Dictionaries
Let’s demonstrate the different methods to merge dictionaries in Python. For this purpose, we’ll utilize the following data.
We have two dictionaries representing two BUs (business units) of an IT company. Both include their employee names as keys along with the work ex as values. You can see the structure below.
Business Unit = { Employee Name: Work Experience, ...}
Here are two dictionaries with some sample data that we need to merge.
unitFirst = { 'Joshua': 10, 'Ryan':5, 'Sally':20, 'Martha':17, 'Aryan':15} unitSecond = { 'Versha': 11, 'Tomi':7, 'Kelly':12, 'Martha':24, 'Barter':9}
The company has also set some goals for merging dictionaries, actually the business units.
- If employee names are duplicates, then unit2 values should override the unit1.
- Both units must have valid keys.
- Both units must have valid integer values.
- Creating a new dictionary should not lead to any changes in the original two.
- Any modification in the new dictionary should not impact the data in
unit1
andunit2
.
Let’s now go through the given methods for merging dictionaries and choose which fits best in your case.
By updating cumulatively
The most obvious way to combine the given dictionaries is to call the update() method for each unit. Since we’ve to keep the unit2 values in case of duplicates, we should update it last.
Please note that we can merge even more than two dictionaries using this approach.
Here is the sample code to merge two dictionaries:
""" Desc: Python program to combine two dictionaries using update() """ # Define two existing business units as Python dictionaries unitFirst = { 'Joshua': 10, 'Ryan':5, 'Sally':20, 'Martha':17, 'Aryan':15} unitSecond = { 'Versha': 11, 'Tomi':7, 'Kelly':12, 'Martha':24, 'Barter':9} # Initialize the dictionary for the new business unit unitThird = {} # Update the unitFirst and then unitSecond unitThird.update(unitFirst) unitThird.update(unitSecond) # Print new business unit # Also, check if unitSecond values overrided the unitFirst values or not print("Unit Third: ", unitThird) print("Unit First: ", unitFirst) print("Unit Second: ", unitSecond)
Running the above code gives the following result:
Unit Third: {'Kelly': 12, 'Ryan': 5, 'Joshua': 10, 'Barter': 9, 'Tomi': 7, 'Aryan': 15, 'Sally': 20, 'Martha': 24, 'Versha': 11} Unit First: {'Sally': 20, 'Ryan': 5, 'Joshua': 10, 'Aryan': 15, 'Martha': 17} Unit Second: {'Tomi': 7, 'Kelly': 12, 'Barter': 9, 'Versha': 11, 'Martha': 24}
You can see that the new unit retained an employee named Martha with a value (24) from the second unit. However, the new dictionary has combined all other elements from the two input dictionaries.
Moreover, we first created a new dictionary to keep the merged output of the other two dictionaries. It’ll make sure that changing the new one won’t affect the values of the original containers.
By using the unpacking operator
In Python 3.5 or above, we can combine even more than two dictionaries with a single expression. Check its syntax below:
# Merging two dictionaries using unpacking operator dictMerged = {**dictFirst, **dictSecond}
Alternatively, we can call this approach using the **kwargs in Python. It helps us pass variable-size key-value pairs to a method. It also treats a dictionary as a sequence of key-value pairs.
When used as **kwargs, it decomposes the dictionary into a series of key-value elements. Let’s consider the following dictionary:
sample_dict = { 'emp1' : 22, 'emp2' : 12, 'emp3' : 9, 'emp4' : 14 }
The **kwargs expression would treat the sample_dict as:
**sample_dict => 'emp1' : 22, 'emp2' : 12, 'emp3' : 9, 'emp4' : 14
So, let’s apply **kwargs or unpacking operator to combine the dictionaries. We’ll operate on the two given business units in our problem statement.
""" Desc: Python program to merge two dictionaries using **kwargs """ # Define two existing business units as Python dictionaries unitFirst = { 'Joshua': 10, 'Ryan':5, 'Sally':20, 'Martha':17, 'Aryan':15} unitSecond = { 'Versha': 11, 'Tomi':7, 'Kelly':12, 'Martha':24, 'Barter':9} # Merge dictionaries using **kwargs unitThird = {**unitFirst, **unitSecond} # Print new business unit # Also, check if unitSecond values override the unitFirst values or not print("Unit Third: ", unitThird) print("Unit First: ", unitFirst) print("Unit Second: ", unitSecond)
After you execute the above program, it should give you the below output:
Unit Third: {'Sally': 20, 'Ryan': 5, 'Versha': 11, 'Tomi': 7, 'Martha': 24, 'Joshua': 10, 'Aryan': 15, 'Kelly': 12, 'Barter': 9} Unit First: {'Aryan': 15, 'Martha': 17, 'Joshua': 10, 'Sally': 20, 'Ryan': 5} Unit Second: {'Barter': 9, 'Martha': 24, 'Kelly': 12, 'Versha': 11, 'Tomi': 7}
Also Read: 5 Ways to Convert Python Dictionary to DataFrame
Combine Two or More Dictionaries
Using the **kwargs method to merge the dictionaries was a very straightforward method.
Let’s also see how it groups three given dictionaries.
""" Desc: Python program to merge three dictionaries using **kwargs """ # Define two existing business units as Python dictionaries unitFirst = { 'Joshua': 10, 'Ryan':5, 'Sally':20, 'Martha':17, 'Aryan':15} unitSecond = { 'Versha': 11, 'Tomi':7, 'Kelly':12, 'Martha':24, 'Barter':9} unitThird = { 'James': 3, 'Tamur':5, 'Lewis':18, 'Daniel':23} # Merge three dictionaries using **kwargs unitFinal = {**unitFirst, **unitSecond, **unitThird} # Print new business unit # Also, check if unitSecond values override the unitFirst values or not print("Unit Final: ", unitFinal) print("Unit First: ", unitFirst) print("Unit Second: ", unitSecond) print("Unit Third: ", unitThird)
You would get the following result upon its execution:
Unit Final: {'Tomi': 7, 'Ryan': 5, 'Tamur': 5, 'Versha': 11, 'James': 3, 'Sally': 20, 'Martha': 24, 'Aryan': 15, 'Daniel': 23, 'Barter': 9, 'Lewis': 18, 'Kelly': 12, 'Joshua': 10} Unit First: {'Joshua': 10, 'Ryan': 5, 'Martha': 17, 'Sally': 20, 'Aryan': 15} Unit Second: {'Tomi': 7, 'Barter': 9, 'Martha': 24, 'Versha': 11, 'Kelly': 12} Unit Third: {'Daniel': 23, 'Tamur': 5, 'James': 3, 'Lewis': 18}
Merge Using Custom Action
While consolidating the data of two or more dictionaries, it is also possible to take some custom action. For example, if a person exists in two units with different work-ex values, then you may like to add up his experience while merging.
Let’s see how to add values for the same keys while combining three dictionaries. Here is a simple example for your help:
""" Desc: Python program to merge dictionaries and add values of same keys """ # Define two existing business units as Python dictionaries unitFirst = { 'Joshua': 10, 'Daniel':5, 'Sally':20, 'Martha':17, 'Aryan':15} unitSecond = { 'Versha': 11, 'Daniel':7, 'Kelly':12, 'Martha':24, 'Barter':9} def custom_merge(unit1, unit2): # Merge dictionaries and add values of same keys out = {**unit1, **unit2} for key, value in out.items(): if key in unit1 and key in unit2: out[key] = [value , unit1[key]] return out # Merge dictionaries while adding values of same keys unitFinal = custom_merge(unitFirst, unitSecond) # Print new business unit # Also, check if unitSecond values override the unitFirst values or not print("Unit Final: ", unitFinal) print("Unit First: ", unitFirst) print("Unit Second: ", unitSecond)
The above program provides a custom function that adds values of the same keys in a list. You can cross-check the same by executing the code:
Unit Final: {'Sally': 20, 'Joshua': 10, 'Barter': 9, 'Versha': 11, 'Kelly': 12, 'Daniel': [7, 5], 'Martha': [24, 17], 'Aryan': 15} Unit First: {'Sally': 20, 'Joshua': 10, 'Daniel': 5, 'Martha': 17, 'Aryan': 15} Unit Second: {'Barter': 9, 'Kelly': 12, 'Daniel': 7, 'Martha': 24, 'Versha': 11}
There are two common key entries in unit1
and unit2
dictionaries, namely Daniel and Martha. In the output, you can see that the program added their values to a list.
We hope that after wrapping up this tutorial, you should feel comfortable using dictionaries in Python. However, you may practice more with examples to gain confidence.
Also, to learn Python from scratch to depth, read our step-by-step Python tutorial.