In automation testing, waiting makes the test execution pause for a certain amount of time before continuing with the next step. Selenium WebDriver provides various commands for implementing wait. In this post, we are going to discuss Webdriver Wait commands supplied by the Selenium WebDriver.
However, if you want to do some serious automation projects, then you must refer to these essential Selenium Webdriver commands from our blog. With every command, you’ll see a related code snippet to describe its usage in real-time situations. To step up the learning ladder, you should also know about the advanced usage of Webdriver Wait commands. For this purpose, please read advanced Selenium Webdriver tutorials from our blog.
Waits help the user troubleshoot issues observed while re-directing to different web pages and during loading of new web elements. After refreshing the page, a time lag appears during the reloading of the web pages and the web elements.
Webdriver Wait commands help here to overcome the issues that occur due to the variation in time lag. Webdriver does this by checking if the element is present or visible, enabled, clickable, etc. WebDriver provides two types of waits for handling recurring page loads, invocation of pop-ups, alert messages, and the reflection of HTML objects on the web page.
Apart from the above waits, you should also read about the Webdriver fluent wait from the below post. We’ve added a small demo there that uses fluent wait and ignores exceptions like <StaleElementReferenceException> or <NoSuchElementException>.
Also Read: Using Webdriver Fluent-Wait with Example and Sample Code.
Let us now discuss implicit and explicit waits in detail.
Learn Webdriver Wait Commands
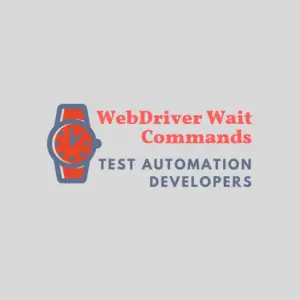
1- Implicit WebDriver Wait Commands.
Implicit waits are used to provide a default waiting time between each consecutive test step/command across the entire test script. Once you’d defined the implicit wait for X seconds, then the next step would only run after waiting for the X seconds.
The drawback with implicit wait is that it increases the test script execution time. It makes each command wait for a stipulated amount of time before resuming the execution. Thus implicit wait may slow down execution of your test scripts if your application responds normally.
1.1- Import Packages.
For using implicit waits in the test scripts, it’s mandatory to import the following package.
import java.util.concurrent.TimeUnit;
1.2- Syntax of Implicit Wait.
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
You need to add the above line of code to the test script. It’ll set an implicit wait soon after the instantiation of the WebDriver instance variable.
1.3- Example of Implicit WebDriver Wait Commands.
Package waitExample; import java.util.concurrent.TimeUnit; import org.openqa.selenium.*; import org.openqa.selenium.firefox.FirefoxDriver; import org.testng.annotations.AfterMethod; import org.testng.annotations.BeforeMethod; import org.testng.annotations.Test; public class WaitTest { private WebDriver driver; private String baseUrl; private WebElement element; @BeforeMethod public void setUp() throws Exception { driver = new FirefoxDriver(); baseUrl = "http://www.google.com"; driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS); } @Test public void testUntitled() throws Exception { driver.get(baseUrl); element = driver.findElement(By.id("lst-ib")); element.sendKeys("Selenium WebDriver Interview questions"); element.sendKeys(Keys.RETURN); List<WebElement> list = driver.findElements(By.className("_Rm")); System.out.println(list.size()); } @AfterMethod public void tearDown() throws Exception { driver.quit(); } }
2- Expected Condition.
It’s a Webdriver concept to play around with the wait commands. With this, you can explicitly build conditional blocks to apply to wait. We’ll read more about these explicit Webdriver Wait commands in the next section.
wait.until(ExpectedConditions.visibilityOfElementLocated(By.xpath("//div[contains(text(),'COMPOSE')]"))); driver.findElement(By.xpath("//div[contains(text(),'COMPOSE')]")).click();
The above command waits either for a stipulated amount of time (defined in the WebDriver Wait class) or for an expected condition to occur whichever occurs first.
In the given code example, we used the <wait> reference variable of the <WebDriverWait> class created in the previous step. Also, added the <ExpectedConditions> class which mentions an actual condition that will most likely occur. Thus whenever the expected condition occurs, the program control will move to the next step for execution instead of forcefully waiting for the entire 30 seconds (defined wait time).
2.1- Types of Expected Conditions.
This class includes several conditions that you can access with the help of a <WebDriverWait> reference variable and the <until()> method.
Let’s discuss a few of them below.
2.1.1- elementToBeClickable().
It can wait for an element to be clickable i.e. it should be present/displayed/visible on the screen as well as enabled.
Sample Code.
wait.until(ExpectedConditions.elementToBeClickable(By.xpath(“//div[contains(text(),’COMPOSE’)]”)));
2.1.2- textToBePresentInElement().
It waits for an HTML object that contains a matching string pattern.
Sample Code.
wait.until(ExpectedConditions.textToBePresentInElement(By.xpath(“//div[@id= ‘forgotPass'”), “text to be found”));
2.1.3- alertIsPresent().
This one waits for an alert box to appear.
Sample Code.
wait.until(ExpectedConditions.alertIsPresent()) !=null);
2.1.4- titleIs().
This command waits for a page with a matching title.
Sample Code.
wait.until(ExpectedConditions.titleIs(“gmail”));
2.1.5- frameToBeAvailableAndSwitchToIt().
It waits for a frame until it is available. After that frame becomes available, the control switches to it automatically.
Sample Code.
wait.until(ExpectedConditions.frameToBeAvailableAndSwitchToIt(By.id(“newframe”)));
3- Explicit WebDriver Wait Commands.
As the name signifies, by using an explicit wait, we explicitly instruct the WebDriver to wait. By using custom coding, we can tell Selenium WebDriver to pause until the expected condition occurs.
What is the need to use the explicit wait when an implicit wait is in place and doing well already? It must be the first question juggling your mind.
Well, there may be instances when some elements take more time to load. Setting implicit wait in such cases may not be wise because the browser will needlessly wait for the same amount of time for every element. All of this would delay the test execution. Hence, the WebDriver brings the concept of Explicit waits to handle such elements by passing the implicit wait. This wait command gives us the ability to apply wait where required. And avoids the force of waiting while executing each of the test steps.
3.1- Import Packages.
For accessing explicit Webdriver Wait commands and to use them in test scripts, it is mandatory to import the following packages into the test script.
import org.openqa.selenium.support.ui.ExpectedConditions import org.openqa.selenium.support.ui.WebDriverWait
3.2- Initialize a Wait object using the WebDriverWait class.
WebDriverWait wait = new WebDriverWait(driver,30);
We created a reference variable named <wait> for the <WebDriverWait> class. Instantiated it using the WebDriver instance. Set the maximum wait time for the execution to layoff. We measure the max wait time in “seconds”.
3.3- WebDriver Code using Explicit Wait.
In the following example, the given test script is about logging into “gmail.com” with a username and password. After a successful login, it waits for the “compose” button to be available on the home page. Finally, it clicks on it.
package waitExample; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; import org.testng.annotations.AfterMethod; import org.testng.annotations.BeforeMethod; import org.testng.annotations.Test; public class ExpectedConditionExample { // created reference variable for WebDriver WebDriver drv; @BeforeMethod public void setup() throws InterruptedException { // initializing drv variable using FirefoxDriver drv=new FirefoxDriver(); // launching gmail.com on the browser drv.get("https://gmail.com"); // maximized the browser window drv.manage().window().maximize(); drv.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); } @Test public void test() throws InterruptedException { // saving the GUI element reference into a "element" variable of WebElement type WebElement element = drv.findElement(By.id("Email")); // entering username element.sendKeys("dummy@gmail.com"); element.sendKeys(Keys.RETURN); // entering password drv.findElement(By.id("Passwd")).sendKeys("password"); // clicking signin button drv.findElement(By.id("signIn")).click(); // explicit wait - to wait for the compose button to be click-able WebDriverWait wait = new WebDriverWait(drv,30); wait.until(ExpectedConditions.visibilityOfElementLocated(By.xpath("//div[contains(text(),'COMPOSE')]"))); // click on the compose button as soon as the "compose" button is visible drv.findElement(By.xpath("//div[contains(text(),'COMPOSE')]")).click(); } @AfterMethod public void teardown() { // closes all the browser windows opened by web driver drv.quit(); } }
In this code, Selenium WebDriver will wait for 30 seconds before throwing a TimeoutException. If it finds the element before 30 seconds, then it will return immediately. And in the next step, it’ll click on the “Compose” button. The test script will not wait for the entire 30 seconds in this case thus saving the wait time.
Footnote – Webdriver Wait Commands
Hope this blog post on the most essential “Webdriver Wait Commands” will help in implementing waits efficiently in your projects. If the above concepts and the examples were able to fetch your interest, then please like this post and share it further on social media.
We always believe in making tutorials that are useful for our readers. And what could be better than that you tell us what you want to see in the next blog post?
If you have any queries about the post, then do write to us.
All the Best,
TechBeamers.