SQL table creation is the process of creating a new table in a SQL database. A table is a collection of data organized into rows and columns. Each row represents a single record, and each column represents a single attribute of that record.
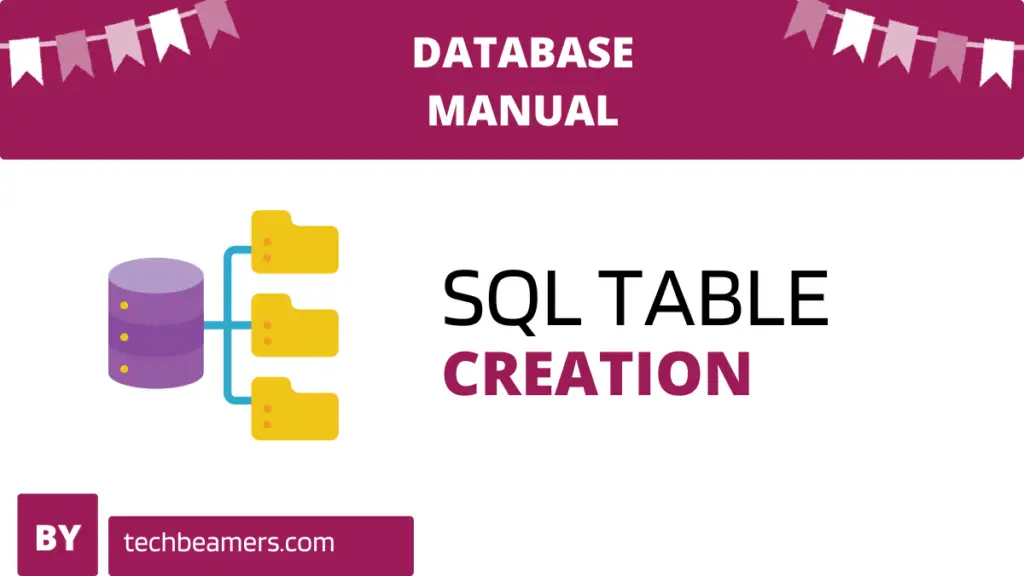
SQL Table Creation
To create an SQL table, you use the CREATE TABLE command. This statement requires you to specify the name of the table, its column names, and their data types. Here’s the basic SQL syntax for table creation:
Syntax
CREATE TABLE table_name ( column_name1 data_type constraint_name1, column_name2 data_type constraint_name2, ... );
Input Parameter | Description |
---|---|
table_name | It is the name of the table you want to create. |
column_name1 and column_name2 | These are the names of the columns in the table. |
data_type | It is the data type of the column. |
constraint_name1 and constraint_name2 | These are the names of the constraints you want to apply to the columns. |
Here are some examples of common SQL constraints:
Constraint | Description |
---|---|
PRIMARY KEY | Combines NOT NULL and UNIQUE to create a unique identifier for rows. |
UNIQUE | Ensures that all values in a column are different. |
NOT NULL | Guarantees a column always has a value, disallowing NULL entries. |
CHECK | Validates values in a column against specified conditions. |
DEFAULT | Sets a default value for a column if no value is provided. |
FOREIGN KEY | Maintains referential integrity between linked tables. |
INDEX | Improves the speed of data retrieval operations on a table. |
CHECK CONSTRAINT | A named constraint that specifies a condition for data integrity. |
Must Try: SQL Programming Test to Check Your Skills Now
Variations of Create Table in SQL
As we have shown above, you can make use of the create table syntax in many ways.
For example, you can specify a primary key in the following manner:
CREATE TABLE TableName ( id INT PRIMARY KEY, ... );
Add a foreign key like this:
CREATE TABLE TableName ( id INT PRIMARY KEY, other_table_id INT, FOREIGN KEY (other_table_id) REFERENCES OtherTable(id) );
You can provide default values for the table fields:
CREATE TABLE TableName ( column1 datatype DEFAULT default_value, ... );
Take a step ahead and set the constraints (e.g., NOT NULL):
CREATE TABLE TableName ( column1 datatype NOT NULL, ... );
You can even conditionally check the constraints:
CREATE TABLE TableName ( column1 datatype, column2 datatype CHECK (column2 >= 0), ... );
One of the most common practices is to assign a unique key constraint.
CREATE TABLE TableName ( column1 datatype, column2 datatype, ... CONSTRAINT constraint_name UNIQUE (column1, column2) );
The above cases cover various aspects of table creation in SQL, including specifying columns, data types, constraints, and references. The specific syntax may vary depending on the DBMS you are using, so be sure to consult the documentation for your database system for precise details.
Examples
Let us now give you some full-fledged examples of table creation.
For example, the following statement creates a table that stores information about sensors, such as their name, type, and location. The UNIQUE constraint ensures that each sensor is uniquely identified by its name, type, and location.
CREATE TABLE sensors ( id INT PRIMARY KEY, name VARCHAR(255) NOT NULL, type VARCHAR(255) NOT NULL, location VARCHAR(255) NOT NULL, UNIQUE (name, type, location) );
Similarly, the following statement creates a table called financial_transactions with five columns: id, account_id, amount, type, and date. The id column is the primary key, account_id is the foreign key, and the subsequent columns are non-null.
CREATE TABLE financial_transactions ( id INT PRIMARY KEY, account_id INT NOT NULL, amount DECIMAL(10,2) NOT NULL, type VARCHAR(255) NOT NULL, date DATETIME NOT NULL, FOREIGN KEY (account_id) REFERENCES accounts (id) );
SQL constraints help you ensure the accuracy and integrity of your data. By using constraints, you can prevent invalid data from being inserted into your database.
Also Read: Upsert in MySQL
Important Facts on SQL Table Creation
Fact | Explanation |
---|---|
A SQL table is a collection of data organized into rows and columns. | Each row represents a single record, and each column represents a single attribute of that record. |
To create a SQL table, you use the CREATE TABLE statement. | This statement specifies the name of the table and the names and data types of the columns. |
Each column in a SQL table must have a unique name. | However, two or more tables can share the same column name. |
Once you have created an SQL table, you can start inserting data into it using the INSERT statement. | This specifies the type of data that can be stored in the column. Common data types include INT , VARCHAR , and DATE . |
You can also specify constraints on the data in a SQL table. | For example, you can specify that a column must be unique, or that it must contain a value greater than zero. |
Once you have created a SQL table, you can start inserting data into it using the INSERT statement. | You can also retrieve data from the table using the SELECT statement. |
5 Most Complex SQL Table Creation Exercises
Here are 5 of the most complex SQL creation exercises with proper explanation:
Exercise 1
Create a table called users
with the following columns:
id
(INT, PRIMARY KEY)name
(VARCHAR(255), NOT NULL)email
(VARCHAR(255), UNIQUE, NOT NULL)role
(ENUM(‘admin’, ‘user’), NOT NULL, DEFAULT ‘user’)body
(TEXT, NOT NULL)created_at (DATETIME, NOT NULL, DEFAULT CURRENT_TIMESTAMP)
Explanation: This exercise is relatively straightforward, but it is a good example of how to use common SQL constraints. The id
column is the primary key, which means that each row in the table must have a unique value for this column. The name
and email
columns are also required, and the email
column must be unique. The role
column is an enumerated value, which means that it can only be one of two values: admin
or user
. The default value for this column is user
. The created_at
column represents the current timestamp that is automatically populated with the current date and time when a new row is inserted into the table.
Exercise 2
Create a table called posts
with the following columns:
id
(INT, PRIMARY KEY)user_id
(INT, NOT NULL, FOREIGN KEY REFERENCES users (id))title
(VARCHAR(255), NOT NULL)body
(TEXT, NOT NULL)published
(BOOLEAN, NOT NULL, DEFAULT FALSE)created_at
(DATETIME, NOT NULL, DEFAULT CURRENT_TIMESTAMP)
Explanation: This exercise is more complex than the first exercise because it includes a foreign key constraint. The user_id
column is a foreign key that references the id
column in the users
table. This means that each post in the posts
table must be associated with a valid user in the users
table.
Exercise 3
Create a table called comments
with the following columns:
id
(INT, PRIMARY KEY)post_id
(INT, NOT NULL, FOREIGN KEY REFERENCES posts (id))user_id
(INT, NOT NULL, FOREIGN KEY REFERENCES users (id))body
(TEXT, NOT NULL)created_at
(DATETIME, NOT NULL, DEFAULT CURRENT_TIMESTAMP)
Explanation: This exercise is similar to the previous exercise, but it includes two foreign key constraints. The post_id
column is a foreign key that references the id
column in the posts
table. The user_id
column is a foreign key that references the id
column in the users
table. This means that each comment in the comments
table must be associated with a valid post in the posts
table and a valid user in the users
table.
Exercise 4
Create a table called tags
with the following columns:
id
(INT, PRIMARY KEY)name
(VARCHAR(255), UNIQUE, NOT NULL)
Explanation: This exercise is relatively straightforward, but it is a good example of how to use a unique constraint. The name
column must be unique, which means that no two tags can have the same name.
Exercise 5
Create a table called post_tags
with the following columns:
post_id
(INT, NOT NULL, FOREIGN KEY REFERENCES posts (id))tag_id
(INT, NOT NULL, FOREIGN KEY REFERENCES tags (id))
Explanation: This exercise is similar to the previous exercise, but it includes two foreign key constraints. The post_id
column is a foreign key that references the id
column in the posts
table. The tag_id
column is a foreign key that references the id
column in the tags
table. This means that each row in the post_tags
table represents a relationship between a post and a tag.
These are just a few examples of complex SQL exercises. Once you have mastered the basics of SQL table creation, you can start to experiment with more complex exercises to challenge yourself and improve your skills.
Database-Specific Differences
At first, the SQL syntax of creating a table sounds similar amongst different databases. This doesn’t lessen the need to know the database-specific differences. Let’s check out some key points.
MySQL:
- Supports a variety of storage engines
- Uses AUTO_INCREMENT to create automatically incrementing primary keys
- Offers ENGINE and CHARACTER SET options
PostgreSQL:
- Offers advanced data types, such as SERIAL for auto-incrementing primary keys
- Supports table inheritance
SQLite:
- Uses dynamic typing
- Supports auto-incrementing primary keys with INTEGER PRIMARY KEY or AUTOINCREMENT
Oracle:
- Requires double quotes for case-sensitive identifiers
- Offers complex data types
- Uses sequences to create automatically incrementing primary keys
SQL Server:
- Uses INT IDENTITY to create automatically incrementing primary keys
- Supports clustered and non-clustered indexes
- Offers extensive security features
Summary
SQL table creation is a fundamental concept in SQL. By understanding the basics of table creation, you can start to build and manage your own databases.
Our given SQL creation exercises demonstrate key concepts, including primary keys, foreign keys, unique constraints, and default values. we strongly recommend going through the below tutorial to take your practice to the next level.
Top SQL Queries Asked in Interviews
Check out the 50 most-asked SQL query interview questions.
We need your support to run this blog, so share this post on your social media accounts like Facebook / Twitter. This is how you will encourage us to come up with more informative stuff.
Happy Learning!