In this tutorial, we’ll compare MySQL vs MongoDB, the two most popular databases. You must be using them as a backend in your projects. Knowing about how these two differ would be useful for you. Please read the database comparison carefully. For any query, do let us know.
Also Read-
MySQL vs PostgreSQL Comparison
Difference Between 3 Python SQL Libraries
MongoDB Database vs MySQL
While comparing the MongoDB database with MySQL, we considered many factors. For example, how they store data, what approach they take to fetch records, how they handle schema changes, etc. So, let’s begin to explore the difference between the two most commonly used databases.
Data Storage Comparison
The fundamental difference between MySQL and MongoDB lies in how they store data. This distinction has a significant impact on their strengths and weaknesses.
MySQL: Structured Tables
MySQL is a relational database, which means it follows a structured approach. Data resides in fixed tables with rows and columns, adhering to a predefined schema. Each column represents a specific data type, ensuring consistency. Imagine a spreadsheet with labeled columns and rows holding specific data points.
Here’s a MySQL example:
CREATE TABLE customers (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(255) NOT NULL,
email VARCHAR(255) UNIQUE,
city VARCHAR(255)
);
This SQL code creates a customers
table in MySQL with columns for id
, name
, email
, and city
. The id
is the primary key, a unique identifier for each customer record (row).
This structure allows for efficient storage of relational data with well-defined relationships between tables. For instance, you could have a orders
table with a foreign key referencing the customer_id
in the customers
table, establishing a connection between customer and their orders.
MongoDB: Flexible Documents
MongoDB is a NoSQL database that stores data in JSON-like documents. They can have different structures within a group. This schema-less approach allows for easy accommodation of diverse data types and evolving data models. Imagine storing info in self-contained envelopes, each with its unique contents and organization.
Here’s a MongoDB example:
{ "_id" : ObjectId("5f4e2b38b421211824000001"), "name" : "T-Shirt", "price" : 19.99, "sizes" : [ "S", "M", "L" ], "reviews" : [ { "author" : "John Doe", "rating" : 5 }, { "author" : "Jane Smith", "rating" : 4 } ] }
This document has fields for name
, price
, sizes
, and an array of reviews
. The structure can differ from other products in the collection. This flexibility is beneficial for data that doesn’t fit neatly into a predefined schema.
Accessing Data in MySQL vs MongoDB
The way you retrieve data from MySQL and MongoDB differs significantly due to their underlying structures. Let’s delve into how data access works in each system.
MySQL: Structured Query Language (SQL)
MySQL utilizes the power of SQL, a standardized language specifically designed for interacting with relational databases. SQL offers a robust set of commands for data manipulation (CRUD – Create, Read, Update, Delete), querying, and managing user privileges.
Here’s an example of a basic SQL query to fetch all customers from the customers
table:
SELECT * FROM customers;
This query retrieves all columns (*
) from all rows in the customers
table. SQL allows for complex queries that can join data from multiple tables based on defined relationships.
For instance, you could join the customers
table with an orders
table to retrieve a customer’s order history:
SELECT c.name, o.order_date, o.total
FROM customers c
INNER JOIN orders o ON c.id = o.customer_id;
This query retrieves customer names (c.name
), order dates (o.order_date
), and order totals (o.total
) by joining the customers
table (c) with the orders
table (o) based on the common customer_id
field.
MongoDB: Ad-hoc Queries with MQL
MongoDB employs a query language inspired by JSON (JavaScript Object Notation). It allows for querying documents based on specific criteria and performing various operations like filtering, sorting, and aggregation.
Here’s a MongoDB query to find products priced below $20:
db.products.find({ price: { $lt: 20 } });
This query finds all documents in the products
collection where the price
field is less than $20 ($lt
is the less than operator). MQL offers flexibility for querying documents with complex inner structures and performing aggregations to summarize data.
For instance, you could calculate the average rating for a product:
db.products.aggregate([ { $match: { name: "T-Shirt" } }, { $unwind: "$reviews" }, { $group: { _id: null, avgRating: { $avg: "$reviews.rating" } } } ])
This query filters for the “T-Shirt” product ($match
), then “unwinds” the reviews
array into separate documents, and finally calculates the average rating ($avg
) using aggregation.
While MQL offers flexibility, it can’t perform complex joins across collections (similar to MySQL tables) as efficiently.
In essence:
- MySQL excels at querying data across relational tables with well-defined structures using SQL.
- MongoDB shines at querying documents with diverse structures within a collection using MQL.
Adapting to Change: MySQL vs MongoDB
As your application evolves, your data structures might need to change. Let’s see how MySQL and MongoDB handle these changes.
MySQL: Stick to the Plan (Schema Modifications)
MySQL is a bit rigid, so changing things requires careful steps, often known as schema migrations.
- Adding a new column: Use ALTER TABLE to adjust the table and maybe fill in default values for existing data.
- Removing a column: Dropping a column means adjusting the table structure and deciding what to do with the existing data.
- Changing data types: If you change a data type, you might need to convert the existing data to match the new type.
These changes can take time and need careful planning to avoid issues with downtime and data consistency.
MongoDB: Go with the Flow (Flexible Schema)
MongoDB is more flexible, making it easier to adapt to changes in how your data is structured.
- Adding a new field: Just add the new field to new documents; old ones won’t have it, but queries still work.
- Removing a field: Keep the data in existing documents as is, and new ones won’t have the field. You can clean up the old ones later.
- Changing data types: MongoDB tries to handle this for you, but testing is essential to catch any issues.
While MongoDB gives you agility in changing data structures, you need to manage consistency across documents.
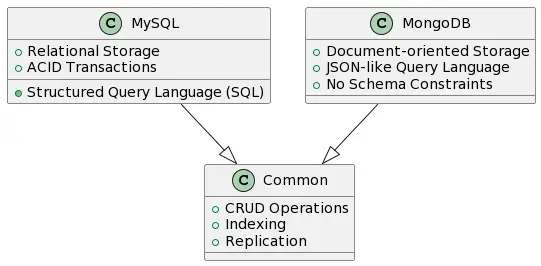
Data Integrity: MySQL vs. MongoDB
Keeping data accurate and reliable is crucial for any app. Let’s see how MySQL and MongoDB make sure your data stays in good shape.
MySQL’s ACID Approach
MySQL follows the ACID rules to keep things reliable:
Atomicity | In MySQL, atomicity ensures that all operations within a transaction are treated as a single unit. If any part of the transaction fails, the entire transaction is rolled back, and no changes are applied. |
Consistency | In MySQL, consistency means the data must stick to the rules you set. For instance, if you say a column can’t be empty, MySQL ensures no one tries to sneak in an empty value. |
Isolation | It means that when multiple things are happening at the same time, they won’t interfere with each other. |
Durability | It means that once something is done (like a transaction), it sticks around, even if there’s a hiccup in the system. MySQL ensures that committed changes are permanent. |
Simple Transaction Example
Creating an order in MySQL goes like this:
START TRANSACTION; INSERT INTO orders (customer_id, order_date) VALUES (123, NOW()); -- Similar steps for order items and updating the cart... COMMIT;
This makes sure an order is created completely, or nothing happens.
MongoDB is Flexible and Smart.
MongoDB database is quite flexible in how it deals with the data. Check out the following table for more details.
Document Checks | MongoDB is great for flexibility. However, it is not easy to keep the data in order. Since it doesn’t force a strict structure (schema-less), ensuring data integrity becomes challenging. That’s where tools like JSON Schema step in—they help ensure your data stays organized and consistent. |
Optimistic Locking | To keep data safe from conflicting changes, use optimistic locking in MongoDB. This means storing a version number with each piece of data. Before making updates, check this version number. If it matches, go ahead with the changes. If not, someone else made changes, and you need to handle that to maintain data integrity. |
Smart Application Logic | When it comes to keeping data consistent, it’s mostly the job of your app. Make sure your application is designed to handle any possible issues that might pop up, especially when there aren’t built-in guarantees for smooth transactions. |
Simple Data Insertion in MongoDB
Let’s look at a MongoDB query to add an order along with its items:
db.orders.insertOne({ customer_id: 123, order_date: new Date(), items: db.cart_items.find({ customer_id: 123, is_purchased: false }).toArray() });
This query highlights how MongoDB is adaptable in managing data, giving you the freedom to structure and add documents on the go.
Making the Right Choice
Remember: MySQL is great for detailed searches and joins. MongoDB excels when you need lots of data fast, like sorting through a big storeroom. Pick the one that fits your needs best!
MySQL | Go for MySQL if you need strong and steady data control with well-defined rules. |
MongoDB | Choose MongoDB if you want flexibility, and your app can manage consistency through its logic. Just be aware of the trade-offs. |
Ensuring Data Availability: MySQL vs. MongoDB
Let’s see how MySQL and MongoDB ensure your data is safe and always accessible:
MySQL
Regular Backups | Think of it like saving your computer data. MySQL lets you create regular backups to act as a safety net. If the server crashes, you can quickly restore your data and keep things running. |
Master-Slave Replication | Imagine two servers – one holds the main data (master), and the other keeps copying it (slave). If the main server has an issue, the backup server steps in, reducing downtime and data loss. |
MongoDB
Replica Sets | Similar to MySQL, MongoDB uses replica sets. One node stores the main data, and others keep copying it. If the main node has an issue, a backup node takes charge, ensuring you can still access your data. |
Automatic Failover | MongoDB’s replica sets include automatic failover. If the main node fails, a backup takes charge automatically, minimizing downtime and keeping your data accessible. |
Sharding (for Large Datasets) | For huge datasets, MongoDB allows sharding, spreading data across multiple servers. It boosts performance but needs careful planning. A well-thought-out strategy ensures data remains available, even if one server has a problem. |
Choosing the Right Fit
Your choice depends on what you need:
Strong Data Consistency | If you need strict consistency and minimal delay between writing and reading data across all servers, MySQL’s master-slave replication is a solid choice. |
High Availability and Scalability | For data available all the time and handling massive datasets, MongoDB’s replica sets and sharding are excellent. Keep in mind there might be a tiny delay in data consistency on backup servers. |
Summary
We hope you would have found the MongoDB database vs MySQL comparison useful. Think about what your application needs – whether it’s consistent data, tolerance for downtime, or the ability to handle lots of data – to make the right choice for your situation.
Lastly, we seek your support, so share this post on your Facebook / Twitter accounts.
Happy learning!