This tutorial explains how to work with the date and time values using Python datetime. This module provides classes for representing and manipulating dates and times.
Please note that this module and its functions could come in quite handy for a variety of applications such as:
-Display dates and times on web pages and perform date- and time-related calculations.
-Analyze and visualize data that contains dates and times.
-Automate tasks that require working with dates and times.
-Calculate financial metrics that depend on dates and times.
How to Use Date and Time in Python
Greetings, hope you had a great day. Today, we plan to educate you on how to find the date, day, time, etc. using a module known as the datetime
in Python.
You should be comfortable with modules in Python before studying the concept of date and time objects in Python. This tutorial uses syntax for Python3 which can be modified for use with Python2.
Pre-requisites
You need to have some level of experience using the modules in Python. They provide readymade functions that you can directly call from any program.
Introduction to Datetime Module
Although we can use the time module to find out the current date and time, it lacks features such as the ability to create Date objects for a particular manipulation.
To remedy this, Python has a built-in datetime module. Hence, to do any object manipulation regarding date and time, we need to import datetime
module.
The datetime
module is used to modify date and time objects in various ways. It contains five classes to manipulate date and time. They are shown in the below table:
Python Datetime Classes | Short Description |
---|---|
date | This class deals with date objects. |
time | It works with time objects. |
datetime | It deals with date and time object combinations. |
timedelta | This class provides functionality related to intervals of time. Used in calculations of past and future date and time objects. |
info | It deals with time zone info of local time. |
We are going to deal with these date and time classes in the example section.
How to Use Datetime Module in Python Programs
To create and modify new date and time objects, we need to import the module. We load them by using the following statement.
import datetime
Or if you want only one of the modules-
from datetime import date from datetime import time from datetime import datetime
We could create new objects that have different date and time values stored for manipulation using the Python datetime module.
The following syntax would do the needful. It is just the pseudo-code.
import datetime datetime.datetime(year_number, month_number, date_number, hours_number, minutes_number, seconds_number)
To format the dates into human-readable strings, we use the function strftime()
that is available in the datetime
module.
The datetime module has another function timedelta()
. It allows calculations on the date and time objects and is useful for doing time operations.
The syntax used in invoking timedelta()
is as follows:
from datetime import timedelta timedelta(days, hours, minutes, seconds, year,...)
Python Datetime Examples
You can copy/paste the below code either in a Python interpreter or in an online Python compiler and run it.
To display today’s date we use the following code:
import datetime print (datetime.date.today())
Or
from datetime import date print (date.today())
To view the individual date components, we need:
Python datetime provides the date class which keeps track of all date and time components. The below code illustrates how can we access them.
from datetime import date print (date.today().day) print (date.today().month) print (date.today().year) #If you prefer to write in one sentence, write it as print (date.today().day,date.today().month,date.today().year)
Since the above code is long, we can rewrite it as:
from datetime import date now = date.today() print (now.day) print (now.month) print (now.year) #You can also display the previous 3 sentences in one sentence print (now.day, now.month, now.year)
This code is cleaner and compact. Try to write the code in the shortest way possible without affecting readability.
The below picture shows the output of the code if you run it from the Python command line interface:
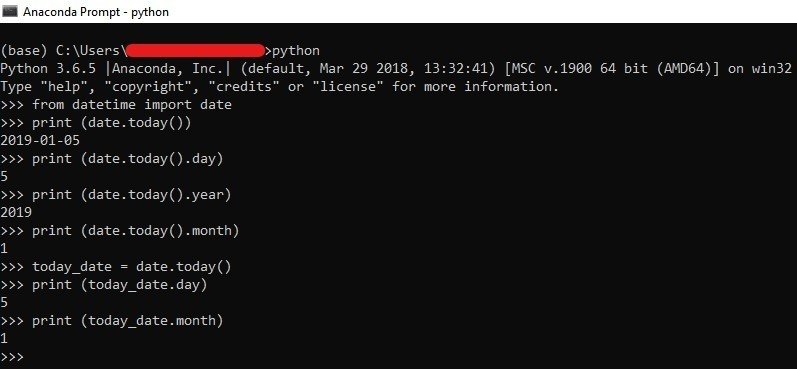
Find out the weekday of the current date:
You could also use the date class from Python datetime to find out the weekday of the current date:
from datetime import date now = date.today() print (f"The week of the day is {now.weekday()}")
The following is the output of the above code:
The week of the day is 4.
To format the date and time into human-readable strings, we use strftime()
in the datetime
module.
The following example clarifies how to use this function and prints the date/time in a styled manner:
import datetime from datetime import datetime as tm Today = tm.now() print (Today.strftime("%a, %B, %d, %y"))
When you run it, you get the following output. Basically, all the date components print separately.
Fri, October, 13, 23
It is important to understand the format codes used in the above code.
Format Code | Short Description |
---|---|
%a | refers to the day |
%B | refers to the month |
%d | refers to the date |
%y | refers to the year |
The above codes are the most common format types to use in Python datetime programs.
However, if you want to print the local date and time using the function strftime()
like the way given in the following example.
import datetime from datetime import datetime as tm Today = tm.now() print ("Today’s date is " ,Today.strftime("%c"))
To display only the local date and local time separately, instead of%c
use %x
.
The following is a sample Python Program using multiple date-time modules.
from datetime import date,time, datetime, timedelta # Time_gap is the timedelta object where # we can do different calculations on it. Time_gap = timedelta(hours = 23, minutes = 34) print ("Future time is" , str(datetime.now() + Time_gap))
The output is as follows.
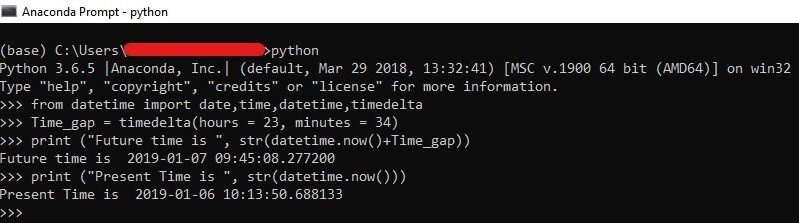
Conclusion – Usages of Datetime Module
Python Datatime It is a powerful feature that allows us to manipulate the system’s date and time without risking any system changes. We can define a new date object and modify its representation.
Arithmetic calculations can be done on the date and time objects for several purposes such as finding the future date, and the present year, formatting of date value into a string, creating a time management solution, etc.