This tutorial compares Page Object Model Vs Properties File approach. Page object model aka POM is a frequently used design pattern for automating the UI flows of a Web application. While using the properties file in Selenium Webdriver is a general concept. It stores large and complex locators by giving them user-friendly names. The only similarity between the duo is that both of them provide a way to form an object repository in Selenium Webdriver.
Page object model encapsulates the locators into classes and exposes methods to manage them. Whereas the properties file in Selenium Webdriver requires a custom Property class to provide a method to read locators. You can also achieve it by writing a Static method to access properties files and get locators. In this blog post, we’ll broadly discuss the differences between the Page Object Model and Properties File.
For readers who wish to learn Selenium from scratch, check out our step-by-step Selenium Webdriver Tutorial.
How to Choose Between Page Object Model & Properties File
Page Object Model Vs Properties File
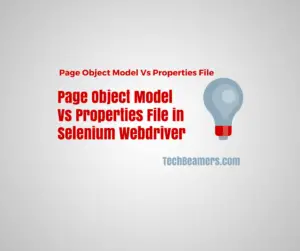
There is no better way of learning than by using practical examples. Let’s begin with the properties file first so that you can easily understand the difference later. You’ll get to learn almost every little detail about the POM and Properties File.
Properties File Approach
There are many ways to structure and reference the Object Repositories (OR) using the properties file in Selenium Webdriver. We’ve also written a dedicated post on implementing property files, refer to it for more details.
Singular Properties File
In this method, you’ve to perform the following steps.
- Store all the object locators in a single <UIMap.properties> file in the form of key-value pairs.
- Create a <Properties.java> class that loads the locators at runtime.
Example
txtEmailId = //input[@id="email"] btnRegister = //button[@class="register"] ...
Pros
- Saves you from using long XPath and ID locators.
- It’s easy to update the locator values.
Cons
- The time to search a locator would increase in proportion to the size of the OR.
- With time the size of the singular OR is going to grow. Managing a large repo would lead to performance issues.
Locator Dependent Properties File.
Classify the properties files based on the locator types in the following style.
- Id.properties.
- ClassName.properties.
- XPath.properties and so on.
Examples
txtEmailId = //input[@id="email"] btnRegister = //button[@class="register"] ...
lnkAccept=btn.green lnkDeny=btn.red lnkWait=btn.orange ...
btnDynamicRow=//table[@id="DynamicData"]/tbody/tr/td[3] ...
Pros
- It’s easy to code when you want to –
- Handle different types of locators based on their file names.
- See how much each of the types is contributing to the size of the object repo.
Cons
- Increases maintenance cost.
- Lead to confusion when a single page consists of locators with different types.
Meta Interfaces to Store Locators
Use the following steps to apply this method.
- Provide an interface for holding <By> locators.
- Implement the interface by creating a Java class.
- Create a class for every page of your web application.
Example
public class RegisterPage implements IRegisterPage { ... } public interface IRegisterPage { By idEmail = By.id("email") By idRegister = By.classname("btnRegister") ... }
Pros
- Since it’ll create context-based objects, so it would be easy to use them instead of directly dealing with the methods.
- It solves most of the problems specified above.
Cons
- Since it requires additional interfaces for each page class, so there could be a noticeable increase in the project footprint.
Page Object Model Approach
The page Object model works best when used in the right situation. It follows the lazy-initialization design. So the Webdriver doesn’t load the elements until they come in the picture. This model is a by-product of the Factory Design Pattern. It recommends creating separate Factory Objects to initialize the other objects. This method leaves behind all others in terms of both maintenance and performance.
Let’s see a quick example of using the page object model.
public class RegisterPage { @FindBy(id = "register") private WebElement registerButton; public RegisterPage(WebDriver driver) { PageFactory.initElements(driver, this); } public void clickRegisterButton() { registerButton.click(); } } public class PageTest { @Test public void firstTest() { RegisterPage page = new RegisterPage(WebDriver driver); page.clickRegisterButton(); } }
Pros and Cons of POM
There are many benefits of the Page Object Model, some of them are as follows.
Pros
- It enforces the creation of classes that are simple and follow user-friendly naming conventions.
- You can rename the methods to make them relevant to the situation.
- Since all the objects of a page stay in one class, so it’s easy to form a context between the page and locators.
- The code is more maintainable and easy to debug.
Cons
- Since the code in POM rests behind an abstraction layer, the testers may find it a bit difficult to grasp at the onset.
- This model leads to loose coupling as it creates additional objects. And it could cause a slight drop in the performance at runtime.
Summary – Page Object Model Vs Properties File
However, the Page object model is the best & the easiest solution to implement. But it’s up to you and the circumstances which may make you choose a different approach.
That’s where the above post can guide you in making the decision to go either with the Page Object Model or the Properties File in Selenium Webdriver.
Finally, if you are happy with this post, please share it on social media. Also, care to like us on our social media accounts for access to our free resources.
Best,
TechBeamers