Selenium has the built-in ability to handle various types of keyboard and mouse events. In this post, we’ll teach you about the Selenium Actions class which enables user interaction with web applications.
To perform mouse operations on a website, first of all, we’ve to launch the browser and then navigate to the URL of the site. The entire control of the browser as well as the application is in the reference variable of type WebDriver.
The WebDriver reference variable can identify any web element that is present on the page. But it doesn’t have the ability to handle the following mouse events.
– Mouse actions on some elements.
– Drag and drop.
– Right-click, and so on.
Hence, you should clearly know that the WebDriver reference variable alone can’t work. So, to perform the mouse actions, we need to make use of the Selenium Actions class.
Selenium Actions Class – Handle Keyboard and Mouse Events
Here is the list of topics that we are going to take up in this post.
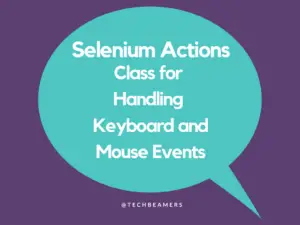
If you are planning for a Selenium interview, then you must read the below post.
First, we’ll initialize the WebDriver instance followed by creating the action builder object. Please check out the below code.
WebDriver driver = new FirefoxDriver(); WebElement ele = driver.findElement(By.xpath(…………)); Actions ref = new Actions (driver); ref.movetoElement(ele); ref.click().build().perform();
In the above code, we are executing more than one action. So we need to use the <build()> method to compile all these into a single step.
Selenium Actions Class Methods
Following is the list of the most commonly used keyboard and mouse events provided by the Selenium Actions class.
Method | Description |
---|---|
<clickAndHold()> | Clicks at the present mouse location (without releasing). |
<contextClick()> | Performs click-and-hold at the source location, shifts by a given offset, and then frees the mouse. Parameters: source- element to grab. xOffset – to shift horizontally.yOffset – to shift vertically. |
<doubleClick()> | It performs a double-click at the existing mouse location. |
<dragAndDrop(source, target)> | Makes a context click at the existing mouse location. |
<dragAndDropBy(source, x-offset, y-offset)> | Performs click-and-hold at the source location, shifts by a given offset, and then frees the mouse. Parameters: source- element to grab. xOffset – to shift horizontally.yOffset – to shift vertically. |
<keyDown(modifier_key)> | Invokes a modifier key press. Does not free the modifier key – subsequent actions may assume it as pressed. Parameters: modifier_key – any of the modifier keys (Keys.ALT, Keys.SHIFT, or Keys.CONTROL ) |
<keyUp(modifier _key)> | Frees up a mouse press. Parameters:modifier_key – any of the modifier keys (Keys.ALT, Keys.SHIFT, or Keys.CONTROL ) |
<moveByOffset(x-offset, y-offset)> | Shifts the mouse from its current position (or 0,0) by the given offset. Parameters: x-offset – Sets the horizontal offset. A (-ve) value means shifting the mouse to the left.y-offset – Sets the vertical offset. A (-ve) value means shifting the mouse to the up. |
<moveToElement(toElement)> | It shifts the mouse to the center of the element. Parameters:<toElement> – target element. |
<release()> | Frees up the depressed left mouse button at the existing mouse location. |
<sendKeys(onElement, charsequence)> | Transmits a series of keystrokes onto the element. Parameters: onElement – an element that will receive the keystrokes, usually a text field.charsequence – any string value representing the sequence of keystrokes to be sent. |
Handle MouseOver Action
Let’s take an example of mouseover action using the Selenium Actions class. In this example, we’ll showcase the automation of the mouseover actions on the following website.
- www.myntra.com
Note: If you wish to use a different site for Selenium testing, then read the below blog post.
Must Read: Demo Websites for Selenium Testing
Our test script will execute the below steps.
- Open the browser.
- Navigate to the given URL (www.myntra.com).
- Mouse Hover on the Men’s tab is present in the top navigational bar.
- Select the option from the Accessories tab.
- Move to the first item displayed on the web page and add it to the cart.
- Lastly, remove the same item from the cart.
- Close the browser.
Selenium Actions Class Example-1
package com.techbeamers.testing; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.interactions.Actions; public class MouseHoverExample { public static void main(String[] args) throws Exception { // Initialize WebDriver WebDriver driver = new FirefoxDriver(); // Wait For Page To Load driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Go to URL driver.get("http://www.myntra.com/"); // Maximize Window driver.manage().window().maximize(); // Mouse Over On " Men link " Actions act = new Actions(driver); By testlink = By.linkText("Men"); WebElement test = driver.findElement(testlink); act.moveToElement(test).build().perform(); // Click on " bags & backpacks " link driver.findElement(By.linkText("Bags & Backpacks")).click(); // Click on the categories - Bag-packs driver.findElement(By.xpath("//*[text()='Categories']//following::li[1]/label")).click(); // Mouse Hover on the 1st bag Actions sel = new Actions(driver); sel.moveToElement(driver.findElement(By.xpath("//ul[@class='results small']/li[1]"))).build().perform(); // Click on the "Add to Bag" icon of the 1st bag driver.findElement(By.xpath("//ul[@class='results small']/li[1]/div[1]//div")).click(); // Hover over the shopping bag icon present on the top navigation bar Actions mov = new Actions(driver); mov.moveToElement(driver.findElement(By.xpath("//a[contains(@class, 'cart')]//div"))).click().build().perform(); // Click on the remove icon driver.findElement(By.xpath("(//span[@data-hint='REMOVE FROM BAG'])[1]")).click(); // Closing current driver window driver.close(); } }
Execute a Sequence of Actions
Suppose we want to execute a sequence of actions on the same element. We can achieve this by using the <build()> method. Let’s demonstrate this with an example.
Please check out the use case and the steps covered in the sample code.
- Open the browser.
- Navigate to the given URL (www.facebook.com).
- Fetch the reference for Web Element Email (textbox).
- Enter the text “hello” into the textbox after converting it into Capital letters.
- Highlight the text in the textbox by clicking on it.
- Lastly, right-click to open the context menu.
- Close the browser.
Our Selenium Actions class sample will run the above steps and call them using the <build()> method.
The sample code for the given scenario is as follows.
Selenium Actions Class Example-2
package com.techbeamers.testing; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.interactions.Action; import org.openqa.selenium.interactions.Actions; public class MultipleActionsExample { public static void main(String[] args) throws Exception { // Initialize WebDriver WebDriver driver = new FirefoxDriver(); // Wait For Page To Load driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Go to URL driver.get("http://www.facebook.com/"); // Maximize Window driver.manage().window().maximize(); // get the reference for Web Element Email WebElement userName = driver.findElement(By.id("email")); // Handling Multiple Actions Actions act = new Actions(driver); Action seriesOfActions = act.moveToElement(userName).click().keyDown(userName, Keys.SHIFT) .sendKeys(userName, "hello").keyUp(userName, Keys.SHIFT).doubleClick(userName).contextClick().build(); seriesOfActions.perform(); // Closing current driver window driver.close(); } }
Final Word – Selenium Actions Class
We most of the time try to pick a topic that can help in our work. Also, we intend to teach you skills that matter the most in your job profile. That’s why we came up with this blog post on using the Selenium Actions class.
It would be great to hear from you if this post helped you in learning Selenium. So do let us know about your experience.
Also, you can submit a request for any topic of your choice. We’ll include it in our plan and add it to the priority list.
If you liked the post, then please don’t miss out on sharing it with friends and on social media.
Keep Learning,
TechBeamers