AngularJS scope is one of the core concepts of the Angular framework. And, it plays a vital role in defining the workflows of an AngularJS application.
In this post, we’ve tried to decipher this concept by elaborating its definition, usage, and examples. After reading, you’ll find it easy to use in your projects.
If you like to quickly brush up on Angular concepts, then do go through the below post once.
Must Read: 100+ AngularJS Interview Questions
AngularJS Scope
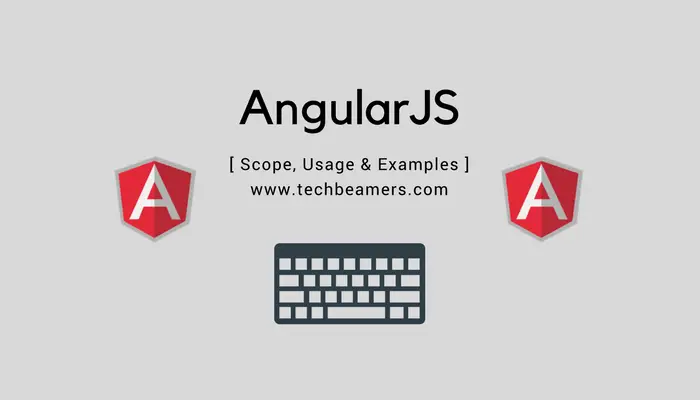
The Scope is a special javascript object, that plays the role of joining the Controller with the View. It contains the Model data. A Controller can access this Model data using <$scope> object. It transfers the data from the Controller to the View and vice-versa. In other words, the Controller initializes the data for the View by making changes to the <$scope>.
How to Use the AngularJS Scope?
Let’s see the code of a Controller that is using a Scope object.
Sample Code.
<div ng-app="myApp" ng-controller="sampleCtrl"> <h1>{{Subject}}</h1> </div> <script> var app = angular.module('myApp', []); app.controller('sampleCtrl', function($scope) { $scope.subject = "Maths"; $scope.marks = " 75%"; }); </script>
Following are the important concepts that we should understand from the above code sample.
- AngularJS passes <$scope> as the first argument to the Controller while defining its constructor.
- <$scope.subject> and <$scope.marks> are the Models to be used in the HTML page.
- We have set values for these Models, which are reflected in the application module. <sampleCtrl> is the Controller for this application.
- The View (HTML) gets access to the properties that we attach to the <$scope> object of the Controller. If we make any modifications in the View, this, in turn, changes the Controller. It brings a change in the values of the properties or methods belonging to <$scope> object.
- In the view, we do not use the prefix <$scope>. Instead, we refer to a property by its name as {{name}}.
- We can also define functions in <$scope>.
Understanding the Scope.
If we assume that an AngularJS application is made up of
- View, which is its HTML code.
- Model, which is the data available for the current view and,
- Controller, which is the JavaScript function that prepares, modifies, deletes, and controls the data.
- Then, we can say that Scope is part of the Model.
To summarize, a Scope is a JavaScript object containing properties and methods, which are available to both the View and the Controller.
If we make any change in the View, the Model and the Controller get updated automatically.
Sample Code.
<div ng-app="myApp" ng-controller="sampleCtrl"> <input ng-model="name"> <h1>My name is {{name}}</h1> </div> <script> var app = angular.module('myApp', []); app.controller('sampleCtrl', function($scope) { $scope.name = "Ram Agarwal"; }); </script>
Know Your Scope.
In the above example, we have defined only one Scope, so determining the Scope is easy. However, HTML DOM of large applications may contain sections, that can access only specific Scope objects.
However, at any time of the code development process, we should know about the Scope object, that we are handling.
Let’s see the following example having two Scopes, Controllers, and roots.
Sample Code.
app.controller('WelcomeCtrl', function($scope) { $scope.message = 'Welcome Viewers !!'; }); app.controller('ErrorCtrl', function($scope) { $scope.message = 'Oops, there is some error, please Retry.'; }); <div ng-controller="WelcomeCtrl"> <input ng-model="message"> <span>{{message}}</span> </div> <div ng-controller="ErrorCtrl"> <input ng-model="message"> <span>{{message}}</span> </div>
Output.
Welcome Viewers !!
Oops, there is some error, please Retry.
Even though both inputs bind to message property, they are completely independent. The reason being their association with different Scopes. Till now we have been talking about Scope, but are we aware of its creation?
Scope Creation: <$rootScope>
When we start an application, AngularJS creates the initial Scope and denotes it as <$rootScope>. All the other <$scope> objects are child objects. The properties and methods attached to <$rootScope> become available to all the Controllers. The following example demonstrates the use of <$rootScope> and <$scope> objects.
Sample Code.
<!DOCTYPE html> <html> <head> <title>AngualrJS Controller</title> <script src="~/Scripts/angular.js"></script> </head> <body ng-app="myApp"> <div ng-controller="parentCtrl"> Controller Name: {{controllerName}} <br /> Message: {{message}} <br /> <div style="margin:10px 0 10px 20px;" ng-controller="childCtrl"> Controller Name: {{controllerName}} <br /> Message: {{message}} <br /> </div> </div> <div ng-controller="siblingCtrl"> Controller Name: {{controllerName}} <br /> Message: {{message}} <br /> </div> <script> var ngApp = angular.module('myApp', []); ngApp.controller('parentCtrl', function ($scope, $rootScope) { $scope.controllerName = "parentCtrl"; $rootScope.message = "Welcome Viewers !!"; }); ngApp.controller('childCtrl', function ($scope) { $scope.controllerName = "childCtrl"; }); ngApp.controller('siblingCtrl', function ($scope) { }); </script> </body> </html>
Output.
Following is the Output for the above code snippet.
Controller Name: parentCtrl Message: Welcome Viewers !! Controller Name: childCtrl Message: Welcome Viewers !! Controller Name: Message: Welcome Viewers !!
As per the above example, each Controller has a separate scope created for it. Properties that get added in <$rootScope> are available to all the Controllers.
Scope Inheritance.
The Scope is bound to a Controller. If we define nested Controllers, then the child Controller will inherit the scope of its parent. Let’s take a sample code.
Sample Code.
<script> var mainApp = angular.module("mainApp", []); mainApp.controller("subjectCtrl", function($scope) { $scope.message = "In subject controller"; $scope.type = "Subject"; }); mainApp.controller("englishCtrl", function($scope) { $scope.message = "In English Controller"; }); </script>
The following are the important points to be considered in the above code.
- We have set the values of Models in <subjectCtrl>.
- We have overridden the message property in the child Controller, <englishCtrl>. When we use the <message> property in <englishCtrl>, it prints the overridden message.
Here is the complete code for the code sample discussed above.
Sample Code.
<html> <head> <title>Angular JS Forms</title> </head> <body> <h2>AngularJS Sample Application</h2> <div ng-app = "mainApp" ng-controller = "subjectCtrl"> <p>{{message}} <br/> {{type}} </p> <div ng-controller = "englishCtrl"> <p>{{message}} <br/> {{type}} </p> </div> <div ng-controller = "mathsCtrl"> <p>{{message}} <br/> {{type}} </p> </div> </div> <script src = "https://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"> </script> <script> var mainApp = angular.module("mainApp", []); mainApp.controller("subjectCtrl", function($scope) { $scope.message = "In Subject Controller"; $scope.type = "Subject"; }); mainApp.controller("englishCtrl", function($scope) { $scope.message = "In English Controller"; }); mainApp.controller("mathsCtrl", function($scope) { $scope.message = "In Maths Controller"; $scope.type = "Maths"; }); </script> </body> </html>
Output.
Name it as <textAngularJS.htm>. Open it in a web browser. The following output gets printed.
AngularJS Sample Application In Subject Controller Subject In English Controller Subject In Maths Controller Maths
If you liked this post on “AngularJS Scope“, then don’t mind sharing it further. Also, follow us on our social media accounts to receive more free learning material.